Detailed explanation of C#Task
Task is an object used to represent asynchronous operations in C#. It is located in the System.Threading.Tasks namespace. Task provides a high-level API for handling concurrent, asynchronous operations, making it easier to write asynchronous code in .NET applications.
Task is an object used to represent asynchronous operations in C#, and it is located in the System.Threading.Tasks namespace. Task provides a high-level API for handling concurrent, asynchronous operations, making it easier to write asynchronous code in .NET applications. The following is a detailed description of some of the main features and usage of Task.
1. Create a Task You can use the Task.Run method to perform an operation on a background thread and return a Task object representing the operation.
csharp
Task task = Task.Run(() => { // 这里是后台线程上执行的代码 Console.WriteLine("Running in a separate thread."); });
2. Wait for Task to complete
You can use the Task.Wait method to wait for one or more Task objects to complete.
csharp
task.Wait(); // 等待 task 完成
Alternatively, you can use the await keyword (in the async method) to asynchronously wait for the Task to complete without blocking the current thread.
csharp
await task; // 异步等待 task 完成
3. Task status
Task objects have several states, including Created (created), WaitingForActivation (waiting for activation), and Running (running) , RanToCompletion (run to completion), Canceled (cancelled) and Faulted (error has occurred). You can check the current status of a Task through the Task.Status property.
4. Exception handling
If an exception occurs in the Task and the exception is not caught, the status of the Task will become Faulted. You can access exception information through the Task.Exception property. When using await, the await expression will re-throw the exception and you need to handle it in a try-catch block.
csharp
try { await task; } catch (AggregateException ae) { // 处理异常 }
5. Task return value
If Task represents a calculation or operation, and you want to return the result, then you can use Task
csharp
Task<int> taskWithResult = Task.Run(() => { // 执行一些计算 return 42; }); int result = await taskWithResult; // 异步等待并获取结果
6. Cancellation of Task
You can use CancellationToken to cancel one or more Tasks.
csharp
CancellationTokenSource cts = new CancellationTokenSource(); Task cancellableTask = Task.Run(() => { for (int i = 0; i < 100; i++) { cts.Token.ThrowIfCancellationRequested(); // 执行一些操作 } }, cts.Token); // 在某个时刻取消任务 cts.Cancel();
7. Task combination
You can use Task.WhenAll or Task.WhenAny to wait for multiple Task objects to complete. Task.WhenAll waits for all tasks to complete, while Task.WhenAny waits for any one task to complete.
csharp
Task task1 = Task.Run(() => { /* ... */ }); Task task2 = Task.Run(() => { /* ... */ }); Task allTasks = Task.WhenAll(task1, task2); await allTasks; // 等待所有任务完成 csharp Task firstTaskToComplete = Task.WhenAny(task1, task2); await firstTaskToComplete; // 等待任何一个任务完成
8. Configure the execution context of the Task
By configuring the TaskScheduler, you can control which thread or thread pool the Task executes on. This is useful in scenarios where finer-grained control over thread usage is required.
Task is a powerful tool for handling asynchronous programming in .NET, allowing you to write more responsive and efficient code, especially when dealing with I/O-intensive or compute-intensive tasks. By understanding the basic concepts and usage of Tasks, you can better take advantage of the asynchronous programming model to improve the performance and scalability of your applications.
The above is the detailed content of Detailed explanation of C#Task. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
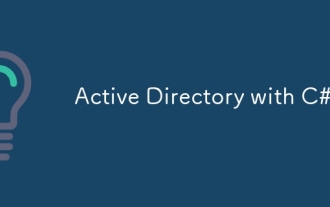
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
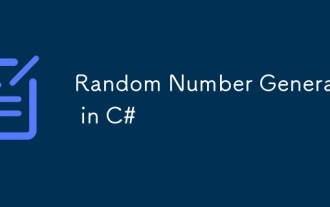
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
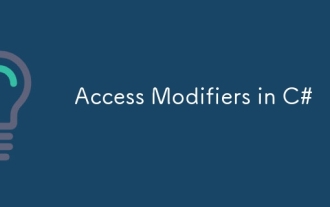
Guide to the Access Modifiers in C#. We have discussed the Introduction Types of Access Modifiers in C# along with examples and outputs.
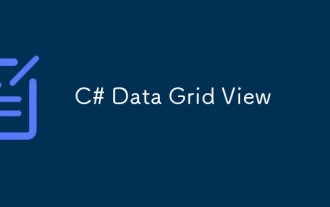
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
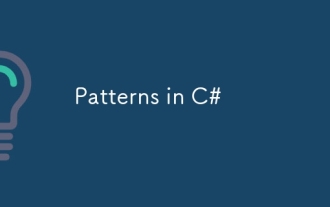
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
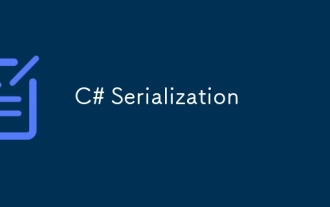
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
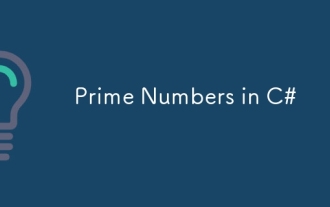
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
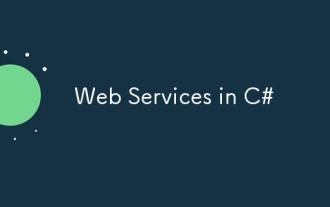
Guide to Web Services in C#. Here we discuss an introduction to Web Services in C# with technology use, limitation, and examples.
