


Practical tutorial: Detailed explanation of shopping cart function with PHP and MySQL
Practical tutorial: Detailed explanation of the shopping cart function with PHP and MySQL
The shopping cart function is one of the common functions in website development. Through the shopping cart, users can easily Add the items you want to buy to the shopping cart, then proceed to checkout and payment. In this article, we will detail how to implement a simple shopping cart function using PHP and MySQL and provide specific code examples.
- Create database and data table
First you need to create a data table in the MySQL database to store product information. The following is an example of a simple data table structure:
CREATE TABLE products ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100) NOT NULL, price DECIMAL(10, 2) NOT NULL, image VARCHAR(100) NOT NULL );
- Web interface design
Next, we need to design a simple web interface to display product information and shopping cart functions. The following is an example of a basic HTML interface:
<!DOCTYPE html> <html> <head> <title>Shopping Cart</title> </head> <body> <h1 id="Product-list">Product list</h1> <ul> <li>Item 1 - 100 yuan <button onclick="addToCart(1)">Add to cart</button></li> <li>Item 2 - 200 yuan <button onclick="addToCart(2)">Add to cart</button></li> <li>Item 3 - 300 yuan <button onclick="addToCart(3)">Add to cart</button></li> </ul> <h1 id="Shopping-Cart">Shopping Cart</h1> <ul id="cart"></ul> <script> function addToCart(productId) { //Add items to shopping cart } </script> </body> </html>
- Write PHP code to implement the shopping cart function
In the PHP code, we need to add and remove items from the shopping cart Products and functions such as calculating the total amount of the shopping cart. Here is a simple PHP code example:
<?php session_start(); //Add items to shopping cart if(isset($_POST['productId'])){ $_SESSION['cart'][] = $_POST['productId']; } //Remove items from shopping cart if(isset($_POST['removeIndex'])){ unset($_SESSION['cart'][$_POST['removeIndex']]); } // Calculate the total amount of the shopping cart $total = 0; if(isset($_SESSION['cart'])){ foreach($_SESSION['cart'] as $productId){ $product = getProductById($productId); $total = $product['price']; } } // Get product information from the database based on the product ID function getProductById($productId){ // Connect to the database $conn = mysqli_connect("localhost", "root", "", "my_database"); // Query product information $result = mysqli_query($conn, "SELECT * FROM products WHERE id=$productId"); $product = mysqli_fetch_assoc($result); //Close database connection mysqli_close($conn); return $product; } ?>
- Update the web interface to display the shopping cart information
Finally, we need to update the web interface to display the product information and total amount that the user has selected in the shopping cart section . Here is an example of the updated HTML interface:
<h1 id="Shopping-Cart">Shopping Cart</h1> <ul id="cart"> <?php if(isset($_SESSION['cart'])){ foreach($_SESSION['cart'] as $key => $productId){ $product = getProductById($productId); echo "<li>{$product['name']} - {$product['price']} yuan<button onclick='removeFromCart($key)'>remove</button></ li>"; } } ?> </ul> <p>Total amount: <?php echo $total; ?>yuan</p>
Through the above steps, we successfully implemented the tutorial of using PHP and MySQL to implement the shopping cart function. Users can browse products on the web page and add them to the shopping cart, and then view the product information and total amount in the shopping cart. In actual projects, the shopping cart function can be expanded and optimized according to needs, such as adding user login and settlement functions. I hope this tutorial is helpful to you, and have a happy programming journey!
The above is the detailed content of Practical tutorial: Detailed explanation of shopping cart function with PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


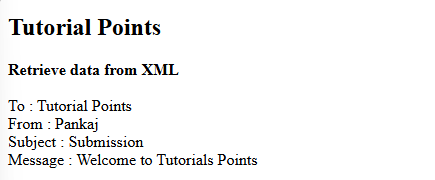
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
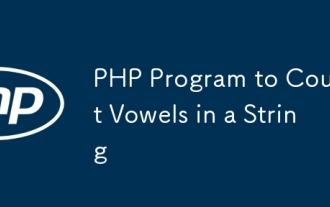
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
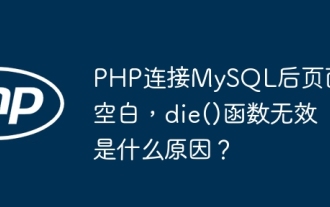
The page is blank after PHP connects to MySQL, and the reason why die() function fails. When learning the connection between PHP and MySQL database, you often encounter some confusing things...
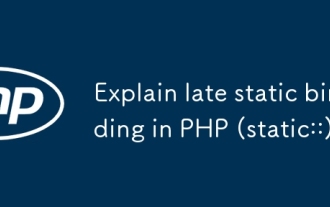
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
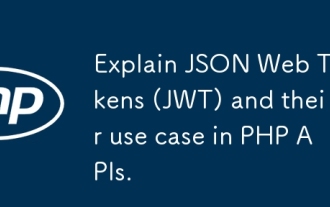
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
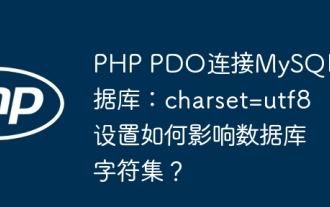
PHP...
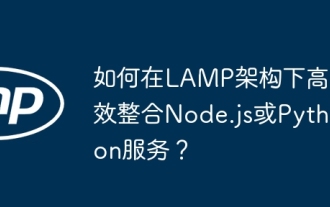
Many website developers face the problem of integrating Node.js or Python services under the LAMP architecture: the existing LAMP (Linux Apache MySQL PHP) architecture website needs...
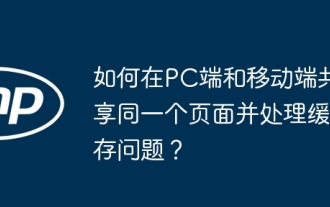
How to share the same page on the PC and mobile side and handle cache issues? In the nginx php mysql environment built using the Baota background, how to make the PC side and...
