


The Maze of Java Inheritance: Navigating Superclasses, Subclasses, and Polymorphism
Mar 16, 2024 pm 12:52 PMIn Java inheritance, the super class (parent class) is a general class that defines the behavior and properties of the object, while the subclass (derived class) inherits from the super class and extends its functionality . Subclasses can use non-private members of the superclass and can override superclass methods.
Polymorphism:
Polymorphism allows the behavior of an object to vary depending on its actual type. In Java, a subclass object can be assigned to a superclass object and when a superclass method is called, the actual method executed depends on the actual type of the object.
Advantages of polymorphism:
- Code reuse: Super classes can define common behaviors and multiple subclasses can be reused.
- Extensibility: It is easy to add new subclasses without modifying the superclass.
- Loose coupling: Client code only needs to interact with the superclass without knowing the actual type of the object.
The challenge of polymorphism:
- Type erasure: After compilation, the subclass type information will disappear, resulting in the inability to distinguish different subclasses at runtime.
- Method overwriting: Subclasses can override superclass methods. If you are not careful, you may destroy the behavior of the parent class.
- Security Violation: If the actual type of the subclass object is different from the type assigned to the superclass object, security issues may result.
Best Practices:
- Clearly define the inheritance relationship: Clearly specify which superclass the subclass inherits from.
- Override methods with caution: Carefully consider the consequences of overriding superclass methods.
- Use abstract classes and interfaces: Abstract classes and interfaces can provide more flexible and safer inheritance.
- Perform unit testing: Ensure polymorphic behavior works properly in all situations.
Common misunderstandings:
- Polymorphism is not inheritance: Polymorphism is based on inheritance, but not inheritance itself.
- Subclasses are always better than superclasses: Subclasses are not necessarily a better choice than superclasses.
- Polymorphism can solve all problems: Polymorphism is a powerful tool, but it cannot solve all software design problems.
Example:
Consider the following example:
class Shape { protected String name; public void draw() { System.out.println("Drawing a shape"); } } class Rectangle extends Shape { public void draw() { super.draw(); System.out.println("Drawing a rectangle"); } } class Circle extends Shape { public void draw() { super.draw(); System.out.println("Drawing a circle"); } } public class Main { public static void main(String[] args) { Shape s1 = new Rectangle(); Shape s2 = new Circle(); s1.draw(); // Prints "Drawing a rectangle" s2.draw(); // Prints "Drawing a circle" } }
In this example, Shape is the superclass that defines common behavior and properties. Rectangle and Circle are subclasses inherited from Shape that extend the behavior of Shape. The main method creates two Shape objects, one assigned to the Rectangle and the other to the Circle. When the draw() method is called, the actual method executed depends on the actual type of the object, demonstrating polymorphism.
The above is the detailed content of The Maze of Java Inheritance: Navigating Superclasses, Subclasses, and Polymorphism. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
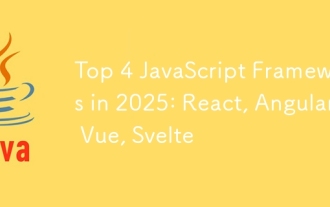
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
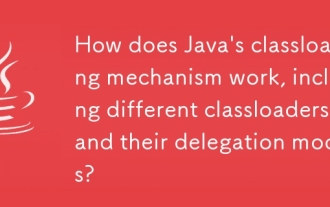
How does Java's classloading mechanism work, including different classloaders and their delegation models?
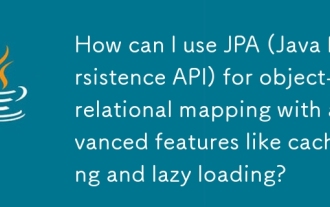
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
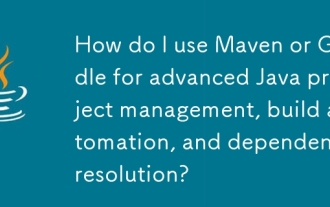
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
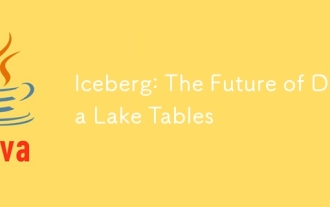
Iceberg: The Future of Data Lake Tables
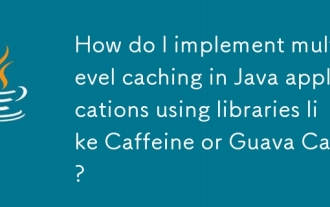
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
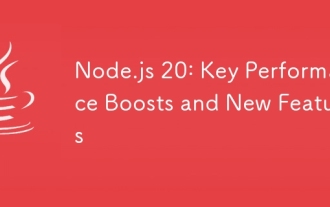
Node.js 20: Key Performance Boosts and New Features
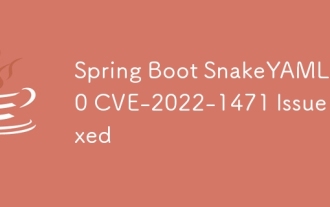
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
