Implement efficient data persistence using Python ORM
Object-relational mapping (ORM) is a technology that allows the use of object-orientedprogramming languagesand relational Establish a bridge between databases. Using python ORM can significantly simplify data persistence operations, thereby improving the development efficiency and maintainability of your application.
Advantage
Using Python ORM has the following advantages:
- Reduce boilerplate code: ORM automatically generates sql queries, thereby avoiding writing a lot of boilerplate code.
- Simplify database interaction: ORM provides a unified interface for interacting with database, simplifying data operations.
- Improve security: ORM uses parameterized queries to prevent security vulnerabilities such as SQL injection.
- Promote data consistency: ORM ensures synchronization between objects and databases and maintains data consistency.
Select ORM
There are many popular Python ORMs, including SQLAlchemy, Django ORM and peewee. When choosing the best ORM for your application, you should consider the following factors:
- Features: Different ORMs provide different features, such as object-relational mapping, relational loading, and query building.
- Performance: The performance of an ORM varies depending on the database type, query complexity, and the ORM itself.
- Community Support: ORMs with active communities usually provide better documentation and support.
Using Python ORM
The following are the general steps for using ORM in Python:
-
Establish a connection to the database: Use the ORM's
create_engine()
function to establish a connection to the database. - Define model classes: Create model classes to represent entities in database tables. Each model class corresponds to a database table.
-
Mapping model classes: Use ORM’s
Table()
function to map model classes to database tables. - Create session: Create a session object to manage database transactions.
- Perform operations: Use the session object to perform data operations such as query, insert, update, and delete.
-
Commit changes: Call the
commit()
method of the session object to persist changes to the database.
Optimize data persistence
Here are some tips for optimizing data persistence:
- Use batch operations: Combine multiple data operations into batches to reduce the number of database round-trips.
- Enable query caching: Using the query caching feature of the ORM, previously executed queries can be reused.
- Index database table: Create indexes on frequently queried columns to improve query performance.
- Use preloading: Perform preloading on associated objects to avoid multiple database queries.
Example
The following example demonstrates how to use SQLAlchemy ORM to persist Python objects to a postgresql database:
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.orm import sessionmaker from sqlalchemy.ext.declarative import declarative_base # Establish database connection engine = create_engine("postgresql://user:passWord@host:port/database") #Define model class Base = declarative_base() class User(Base): __tablename__ = "users" id = Column(Integer, primary_key=True) name = Column(String(50)) email = Column(String(100)) # Mapping model class Base.metadata.create_all(engine) # Create session Session = sessionmaker(bind=engine) session = Session() #Create user entity user = User(name="John Doe", email="john.doe@example.com") #Add entity to session session.add(user) # Submit changes session.commit() #Query user entity user = session.query(User).filter_by(name="John Doe").first() #Print user name print(user.name) # Close session session.close()
in conclusion
By using Python ORM, developers can effectively manage data persistence, thereby improving application development efficiency and maintainability. By choosing the right ORM and following optimization best practices, you can further improve the performance and reliability of your data persistence.
The above is the detailed content of Implement efficient data persistence using Python ORM. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


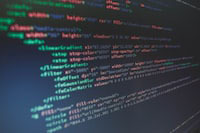
Object-relational mapping (ORM) frameworks play a vital role in python development, they simplify data access and management by building a bridge between object and relational databases. In order to evaluate the performance of different ORM frameworks, this article will benchmark against the following popular frameworks: sqlAlchemyPeeweeDjangoORMPonyORMTortoiseORM Test Method The benchmarking uses a SQLite database containing 1 million records. The test performed the following operations on the database: Insert: Insert 10,000 new records into the table Read: Read all records in the table Update: Update a single field for all records in the table Delete: Delete all records in the table Each operation
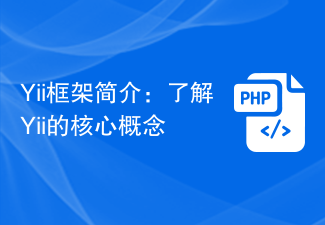
The Yii framework is a high-performance, highly scalable, and highly maintainable PHP development framework that is highly efficient and reliable when developing Web applications. The main advantage of the Yii framework is its unique features and development methods, while also integrating many practical tools and functions. The core concept of the Yii framework, the MVC pattern, Yii adopts the MVC (Model-View-Controller) pattern, which is a pattern that divides the application into three independent parts, namely the business logic processing model and the user interface presentation model.
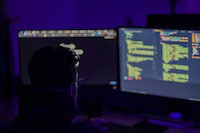
Object-relational mapping (ORM) is a programming technology that allows developers to use object programming languages to manipulate databases without writing SQL queries directly. ORM tools in python (such as SQLAlchemy, Peewee, and DjangoORM) simplify database interaction for big data projects. Advantages Code Simplicity: ORM eliminates the need to write lengthy SQL queries, which improves code simplicity and readability. Data abstraction: ORM provides an abstraction layer that isolates application code from database implementation details, improving flexibility. Performance optimization: ORMs often use caching and batch operations to optimize database queries, thereby improving performance. Portability: ORM allows developers to
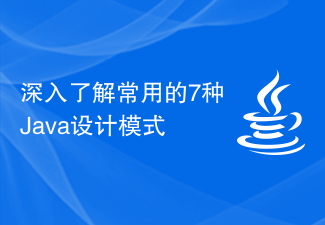
Understanding Java Design Patterns: An introduction to 7 commonly used design patterns, specific code examples are required. Java design patterns are a universal solution to software design problems. It provides a set of widely accepted design ideas and codes of conduct. Design patterns help us better organize and plan the code structure, making the code more maintainable, readable and scalable. In this article, we will introduce 7 commonly used design patterns in Java and provide corresponding code examples. Singleton Patte
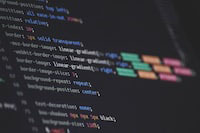
Object-relational mapping (ORM) is a technology that allows building a bridge between object-oriented programming languages and relational databases. Using pythonORM can significantly simplify data persistence operations, thereby improving application development efficiency and maintainability. Advantages Using PythonORM has the following advantages: Reduce boilerplate code: ORM automatically generates sql queries, thereby avoiding writing a lot of boilerplate code. Simplify database interaction: ORM provides a unified interface for interacting with the database, simplifying data operations. Improve security: ORM uses parameterized queries, which can prevent security vulnerabilities such as SQL injection. Promote data consistency: ORM ensures synchronization between objects and databases and maintains data consistency. Choose ORM to have
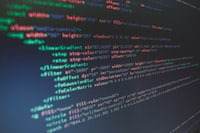
Tkinter is a powerful library for creating graphical user interfaces (GUIs) in python. It is known for its simplicity, cross-platform compatibility, and seamless integration with the Python ecosystem. By using Tkinter, you can add a user-friendly interface to your project, improving user experience and simplifying interaction with your application. Creating a Tkinter GUI application To create a GUI application using Tkinter, perform the following steps: Import the Tkinter library: importtkinterastk Create the Tkinter main window: root=tk.Tk() Configure the main window: Set the window title, size, position, etc. Add GUI elements: Using Tki
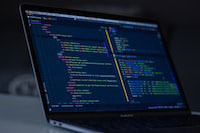
Git is a distributed version control system that helps teams collaborate on software development. For Java developers, understanding Git is crucial because it provides a platform for managing code changes, tracking code history, and collaborating with others. Install Git for newbies (understand the basics): Install Git software and set environment variables. Create repository: Use gitinit to create a local repository. Add files: Use gitadd to add files to the staging area. Commit changes: Use gitcommit to commit changes in the staging area to the local repository. Intermediate (collaboration and version control) cloning a repository: Use gitclone to clone a local copy from a remote repository. Branching and Merging: Use branches to create isolated copies of your code
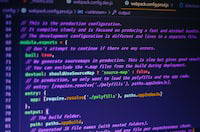
Both python and Jython are popular programming languages, but they are optimized for different use cases and have unique advantages and disadvantages when it comes to cross-platform development. Python Advantages: Extensive libraries and community support Easy to learn and use, suitable for beginners Highly portable, can run across multiple platforms Supports multiple programming paradigms, including object-oriented, functional and imperative programming Disadvantages: Lower performance, Not suitable for processing intensive computing tasks High memory consumption May require additional tools and configuration on some platforms Jython Advantages: Fully compatible with Python, can use all Python libraries and tools Run on the Java Virtual Machine (JVM), providing Seamless integration with the Java ecosystem performs better than Py
