


In-depth understanding of the application of keywords in Golang programming
In Golang programming, keywords are identifiers with special meanings that play an important role in the program. Proficiency in the use of keywords can help programmers better write efficient and reliable code. This article will delve into several commonly used keywords in Golang programming and illustrate them with specific code examples.
1. var
var
is a keyword used to declare a variable and optionally specify the variable type. In Golang, the declaration of variables must start with the keyword var
.
package main import "fmt" func main() { var a int // Declare a variable a of type int a = 10 //Assign a value to variable a fmt.Println(a) // Output the value of variable a }
2. const
const
is the keyword used to declare constants. Once declared, its value cannot be modified. Constants are usually used to define fixed values in programs.
package main import "fmt" func main() { const pi = 3.14159 //Define a constant for pi fmt.Println(pi) // Output the value of pi }
3. func
func
is the keyword to define a function. It is used to declare a function and define its function. In Golang, all program logic must be placed in functions.
package main import "fmt" func greet(name string) { fmt.Println("Hello, " name "!") } func main() { greet("Alice") // Call the greet function and pass in the parameters }
4. package
package
is the keyword used to define a package. In Golang, all code must be placed in a package. Every Go file must have a package declaration to indicate which package the file belongs to.
package main import "fmt" func main() { fmt.Println("Hello, Golang!") }
5. import
import
is the keyword used to import external packages. Modular programming in Golang is very important. Through import
Functionality provided by other packages can be introduced.
package main import "fmt" func main() { fmt.Println("Hello, Golang!") }
The above are some examples of commonly used keywords in Golang programming. Mastering the usage of these keywords can help us better understand and write Golang programs. Of course, there are many other keywords that are used in actual programming. I hope that the introduction in this article can enhance the understanding and application of Golang keywords.
The above is the detailed content of In-depth understanding of the application of keywords in Golang programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
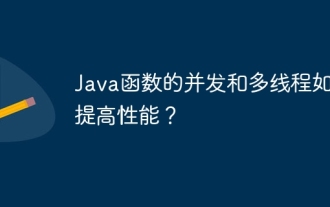
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
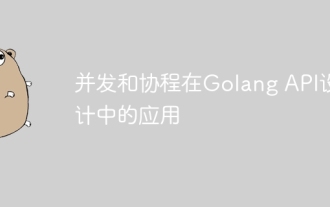
Concurrency and coroutines are used in GoAPI design for: High-performance processing: Processing multiple requests simultaneously to improve performance. Asynchronous processing: Use coroutines to process tasks (such as sending emails) asynchronously, releasing the main thread. Stream processing: Use coroutines to efficiently process data streams (such as database reads).

Lambda expression is an anonymous function without a name, and its syntax is: (parameter_list)->expression. They feature anonymity, diversity, currying, and closure. In practical applications, Lambda expressions can be used to define functions concisely, such as the summation function sum_lambda=lambdax,y:x+y, and apply the map() function to the list to perform the summation operation.
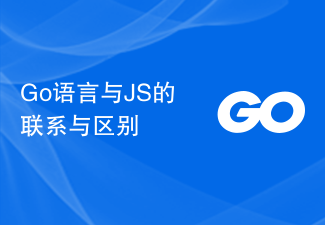
The connection and difference between Go language and JS Go language (also known as Golang) and JavaScript (JS) are currently popular programming languages. They are related in some aspects and have obvious differences in other aspects. This article will explore the connections and differences between the Go language and JavaScript, and provide specific code examples to help readers better understand these two programming languages. Connection: Both Go language and JavaScript are cross-platform and can run on different operating systems.
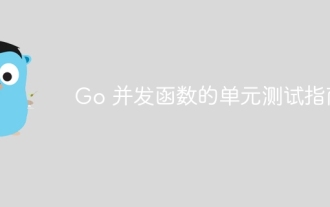
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
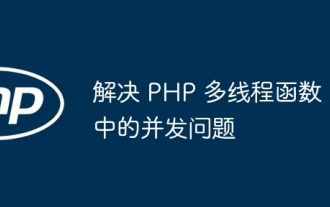
Concurrency issues in PHP multi-threaded functions can be solved by using synchronization tools (such as mutex locks) to manage multi-threaded access to shared resources. Use functions that support mutual exclusion options to ensure that the function is not called again while another thread is executing. Wrap non-reentrant functions in synchronized blocks to protect function calls.

The Java concurrency library provides a variety of tools, including: Thread pool: used to manage threads and improve efficiency. Lock: used to synchronize access to shared resources. Barrier: Used to wait for all threads to reach a specified point. Atomic operations: indivisible units, ensuring thread safety. Concurrent queue: A thread-safe queue that allows multiple threads to operate simultaneously.
