How to split string into smaller chunks in PHP
php editor Xinyi introduces you how to use PHP to split a string into smaller chunks. During development, sometimes you need to split long strings into smaller pieces for processing or display. PHP provides a variety of methods to achieve this purpose, such as using the substr function, str_split function or regular expressions. This article will explain in detail the usage and applicable scenarios of these methods to help you easily implement the string splitting function.
PHP String Splitting
Split string
php provides multiple methods to split a string into smaller chunks:
1. explode() function
explode()
The function takes a string and a delimiter as input and returns an array containing the string split chunks.
$string = "John Doe,123 Main Street,New York"; $parts = explode(",", $string); // $parts will contain ["John Doe", "123 Main Street", "New York"]
2. preg_split() function
preg_split()
The function uses regular expressions to split strings. It provides more flexible control than the explode()
function.
$string = "John Doe;123 Main Street;New York"; $parts = preg_split("/;/", $string); // $parts will contain ["John Doe", "123 Main Street", "New York"]
3. str_split() function
str_split()
The function splits a string into an array of substrings of a specified length.
$string = "ABCDEFGHIJ"; $parts = str_split($string, 3); // $parts will contain ["ABC", "DEF", "GHI", "J"]
Operator
It is also possible to use the operators strtok()
and preg_match()
to split strings, but they are relatively uncommon choices.
Merge string blocks
After splitting a string, you can merge the blocks back into a single string using:
1. implode() function
implode()
Function combines the elements in an array into a single string, using the specified delimiter.
$parts = ["John Doe", "123 Main Street", "New York"]; $string = implode(",", $parts); // $string will equal "John Doe,123 Main Street,New York"
2. .= operator
The.=
operator appends a string to an existing string.
$string = ""; foreach ($parts as $part) { $string .= $part . ","; } // $string will equal "John Doe,123 Main Street,New York,"
Example usage
Find and replace text
$string = "The quick brown fox jumps over the lazy dog."; $parts = explode(" ", $string); $parts[3] = "fast"; $newString = implode(" ", $parts); // $newString will equal "The quick brown fast fox jumps over the lazy dog."
Extract query parameters from URL
$url = "https://example.com/index.php?name=John&age=30"; parse_str(parse_url($url, PHP_URL_QUERY), $params); // $params will contain ["name" => "John", "age" => "30"]
Split CSV file
$file = fopen("data.csv", "r"); while (($line = fgetcsv($file)) !== false) { // $line will contain each line in the file as an array }
The above is the detailed content of How to split string into smaller chunks in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
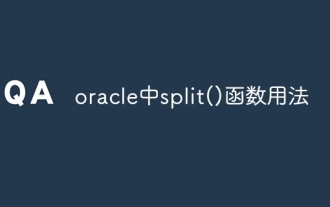
The SPLIT() function splits a string into an array by a specified delimiter, returning a string array where each element is a delimiter-separated portion of the original string. Usage includes: splitting a comma-separated list of values into an array, extracting filenames from paths, and splitting email addresses into usernames and domains.
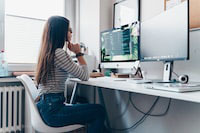
This article will explain in detail how PHP formats rows into CSV and writes file pointers. I think it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Format rows to CSV and write to file pointer Step 1: Open file pointer $file=fopen("path/to/file.csv","w"); Step 2: Convert rows to CSV string using fputcsv( ) function converts rows to CSV strings. The function accepts the following parameters: $file: file pointer $fields: CSV fields as an array $delimiter: field delimiter (optional) $enclosure: field quotes (
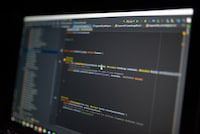
This article will explain in detail about changing the current umask in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Overview of PHP changing current umask umask is a php function used to set the default file permissions for newly created files and directories. It accepts one argument, which is an octal number representing the permission to block. For example, to prevent write permission on newly created files, you would use 002. Methods of changing umask There are two ways to change the current umask in PHP: Using the umask() function: The umask() function directly changes the current umask. Its syntax is: intumas
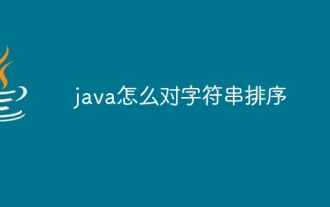
Ways to sort strings in Java: Use the Arrays.sort() method to sort an array of strings in ascending order. Use the Collections.sort() method to sort a list of strings in ascending order. Use the Comparator interface for custom sorting of strings.
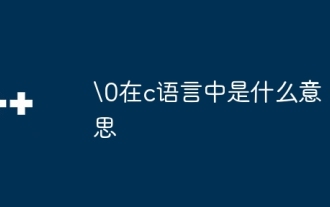
In C language, \0 is the end mark of a string, called the null character or terminator. Since strings are stored in memory as byte arrays, the compiler recognizes the end of the string via \0, ensuring that strings are handled correctly. \0 How it works: The compiler stops reading characters when it encounters \0, and subsequent characters are ignored. \0 itself does not occupy storage space. Benefits include reliable string handling, improved efficiency (no need to scan the entire array to find the end), and ease of comparison and manipulation.
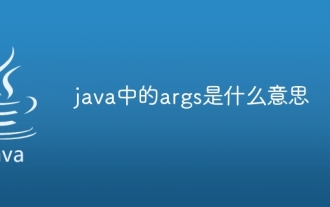
args is a special parameter array of the main method in Java, used to obtain a string array of command line parameters or external input. By accessing the args array, the program can read these arguments and process them as needed.
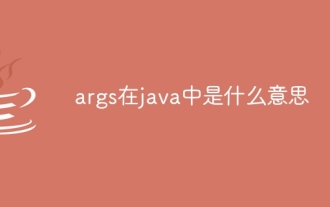
args stands for command line arguments in Java and is an array of strings containing the list of arguments passed to the program when it is started. It is only available in the main method, and its default value is an empty array, with each parameter accessible by index. args is used to receive and process command line arguments to configure or provide input data when a program starts.

This article will explain in detail the numerical encoding of the error message returned by PHP in the previous Mysql operation. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. . Using PHP to return MySQL error information Numeric Encoding Introduction When processing mysql queries, you may encounter errors. In order to handle these errors effectively, it is crucial to understand the numerical encoding of error messages. This article will guide you to use php to obtain the numerical encoding of Mysql error messages. Method of obtaining the numerical encoding of error information 1. mysqli_errno() The mysqli_errno() function returns the most recent error number of the current MySQL connection. The syntax is as follows: $erro
