


Introduction to common network protocols and functions in the Linux protocol stack
As an open source operating system, Linux’s network protocol stack plays a vital role, responsible for processing network data transmission, routing, connection management and other functions. This article will introduce some common network protocols and their functions in the Linux protocol stack, and attach specific code examples.
- TCP Protocol
TCP (Transmission Control Protocol) is a connection-oriented, reliable, byte stream-based transmission protocol. It provides data integrity verification, flow control, congestion control and other functions to ensure reliable transmission of data.
The following is a simple example of using the TCP protocol for client-server communication:
// TCP client #include <sys/socket.h> #include <netinet/in.h> #include <stdio.h> #include <string.h> int main() { int sockfd; struct sockaddr_in server_addr; char buffer[1024]; sockfd = socket(AF_INET, SOCK_STREAM, 0); server_addr.sin_family = AF_INET; server_addr.sin_port = htons(8080); server_addr.sin_addr.s_addr = inet_addr("127.0.0.1"); connect(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr)); send(sockfd, "Hello, server!", strlen("Hello, server!"), 0); recv(sockfd, buffer, sizeof(buffer), 0); printf("%s ", buffer); close(sockfd); return 0; }
// TCP server #include <sys/socket.h> #include <netinet/in.h> #include <stdio.h> #include <string.h> int main() { int sockfd, client_sock; struct sockaddr_in server_addr, client_addr; char buffer[1024]; sockfd = socket(AF_INET, SOCK_STREAM, 0); server_addr.sin_family = AF_INET; server_addr.sin_port = htons(8080); server_addr.sin_addr.s_addr = htonl(INADDR_ANY); bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr)); listen(sockfd, 5); int addr_len = sizeof(client_addr); client_sock = accept(sockfd, (struct sockaddr*)&client_addr, &addr_len); recv(client_sock, buffer, sizeof(buffer), 0); printf("%s ", buffer); send(client_sock, "Hello, client!", strlen("Hello, client!"), 0); close(client_sock); close(sockfd); return 0; }
- UDP Protocol
UDP (User Datagram Protocol) is a connectionless, unreliable transmission protocol that does not guarantee data The reliability and sequence are suitable for some scenarios with high real-time requirements.
The following is a simple example of using UDP protocol for client-server communication:
// UDP client #include <sys/socket.h> #include <netinet/in.h> #include <stdio.h> #include <string.h> int main() { int sockfd; struct sockaddr_in server_addr; char buffer[1024]; sockfd = socket(AF_INET, SOCK_DGRAM, 0); server_addr.sin_family = AF_INET; server_addr.sin_port = htons(8080); server_addr.sin_addr.s_addr = inet_addr("127.0.0.1"); sendto(sockfd, "Hello, server!", strlen("Hello, server!"), 0, (struct sockaddr*)&server_addr, sizeof(server_addr)); recv(sockfd, buffer, sizeof(buffer), 0); printf("%s ", buffer); close(sockfd); return 0; }
// UDP server #include <sys/socket.h> #include <netinet/in.h> #include <stdio.h> #include <string.h> int main() { int sockfd; struct sockaddr_in server_addr, client_addr; char buffer[1024]; sockfd = socket(AF_INET, SOCK_DGRAM, 0); server_addr.sin_family = AF_INET; server_addr.sin_port = htons(8080); server_addr.sin_addr.s_addr = htonl(INADDR_ANY); bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr)); int addr_len = sizeof(client_addr); recvfrom(sockfd, buffer, sizeof(buffer), 0, (struct sockaddr*)&client_addr, &addr_len); printf("%s ", buffer); sendto(sockfd, "Hello, client!", strlen("Hello, client!"), 0, (struct sockaddr*)&client_addr, addr_len); close(sockfd); return 0; }
除了TCP和UDP协议外,Linux协议栈还支持诸如IP协议、ICMP协议、ARP协议等网络协议,它们共同构成了一个完整的网络通信体系,为应用程序提供了丰富的网络通信功能。通过学习和理解这些网络协议,我们可以更好地利用Linux操作系统进行网络编程,构建高效可靠的网络应用。
The above is the detailed content of Introduction to common network protocols and functions in the Linux protocol stack. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


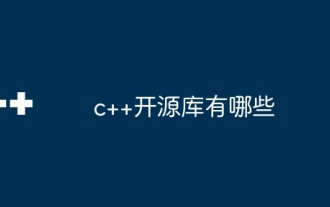
C++ provides a rich set of open source libraries covering the following functions: data structures and algorithms (Standard Template Library) multi-threading, regular expressions (Boost) linear algebra (Eigen) graphical user interface (Qt) computer vision (OpenCV) machine learning (TensorFlow) Encryption (OpenSSL) Data compression (zlib) Network programming (libcurl) Database management (sqlite3)

The C++ standard library provides functions to handle DNS queries in network programming: gethostbyname(): Find host information based on the host name. gethostbyaddr(): Find host information based on IP address. dns_lookup(): Asynchronously resolves DNS.

Commonly used protocols in Java network programming include: TCP/IP: used for reliable data transmission and connection management. HTTP: used for web data transmission. HTTPS: A secure version of HTTP that uses encryption to transmit data. UDP: For fast but unstable data transfer. JDBC: used to interact with relational databases.
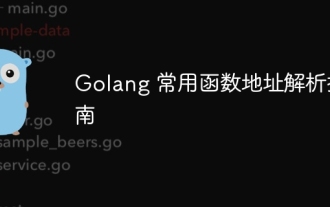
The key functions for parsing addresses in the Go language include: net.ParseIP(): Parse IPv4 or IPv6 addresses. net.ParseCIDR(): Parse CIDR tags. net.ResolveIPAddr(): Resolve hostname or IP address into IP address. net.ResolveTCPAddr(): Resolve host names and ports into TCP addresses. net.ResolveUDPAddr(): Resolve host name and port into UDP address.
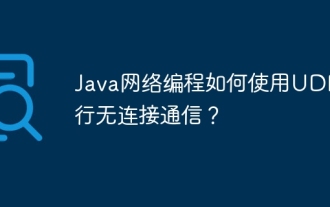
UDP (User Datagram Protocol) is a lightweight connectionless network protocol commonly used in time-sensitive applications. It allows applications to send and receive data without establishing a TCP connection. Sample Java code can be used to create a UDP server and client, with the server listening for incoming datagrams and responding, and the client sending messages and receiving responses. This code can be used to build real-world use cases such as chat applications or data collection systems.
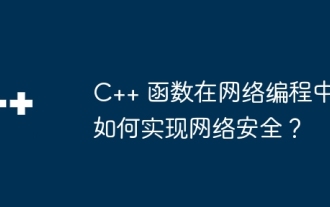
C++ functions can achieve network security in network programming. Methods include: 1. Using encryption algorithms (openssl) to encrypt communication; 2. Using digital signatures (cryptopp) to verify data integrity and sender identity; 3. Defending against cross-site scripting attacks ( htmlcxx) to filter and sanitize user input.

Java entry-to-practice guide: including introduction to basic syntax (variables, operators, control flow, objects, classes, methods, inheritance, polymorphism, encapsulation), core Java class libraries (exception handling, collections, generics, input/output streams , network programming, date and time API), practical cases (calculator application, including code examples).
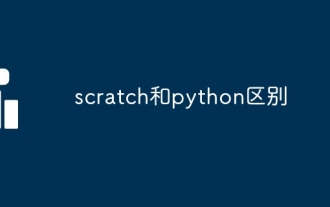
The differences between Scratch and Python are: Target Audience: Scratch is aimed at beginners and educational settings, while Python is aimed at intermediate to advanced programmers. Syntax: Scratch uses a drag-and-drop building block interface, while Python uses a text syntax. Features: Scratch focuses on ease of use and visual programming, while Python offers more advanced features and extensibility.
