How to read the first few records in a database using PHP?
How to read the first few records in the database using PHP?
When developing web applications, we often need to read data from the database and display it to the user. Sometimes, we only need to display the first few records in the database, not the entire content. This article will teach you how to use PHP to read the first few records in the database and provide specific code examples.
First, assume you have connected to the database and selected the table you want to operate on. The following is a simple database connection example:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
Next, we will show how to use PHP to read the first few records in the database. We will use the MySQL database as an example, assuming we want to read the first 3 records in a table named users
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
In the above code, we first build a SQL query statement, use LIMIT 3
to limit the number of query results to the first 3 records. We then execute the query and iterate through the result set, printing the ID and name of each record.
Please make sure to change the database connection information, table name and field name according to your actual situation, and modify LIMIT
in the query statement to limit the number of records. You can also add additional conditions to filter the data you want to query as needed.
In short, with the above example, you should be able to easily read the first few records in the database using PHP. Hope this article can be helpful to you!
The above is the detailed content of How to read the first few records in a database using PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




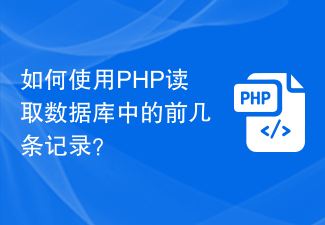
How to read the first few records in a database using PHP? When developing web applications, we often need to read data from the database and display it to the user. Sometimes, we only need to display the first few records in the database, not the entire content. This article will teach you how to use PHP to read the first few records in the database and provide specific code examples. First, assume that you have connected to the database and selected the table you want to operate on. The following is a simple database connection example:
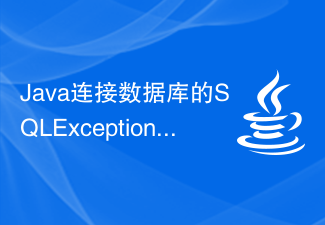
In Java programs, connecting to the database is a very common operation. Although ready-made class libraries and tools can be used to connect to the database, various abnormal situations may still occur during program development, among which SQLException is one of them. SQLException is an exception class provided by Java. It describes errors that occur when accessing the database, such as query statement errors, table non-existence, connection disconnection, etc. For Java programmers, especially those using JDBC (Java Data

Go language connects to the database by importing the database driver, establishing a database connection, executing SQL statements, using prepared statements and transaction processing. Detailed introduction: 1. Import the database driver and use the github.com/go-sql-driver/mysql package to connect to the MySQL database; 2. Establish a database connection and provide the database connection information, including the database address, user name, password, etc. Establish a database connection and so on through the sql.Open function.
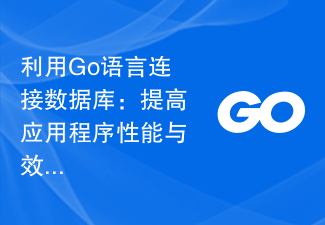
Using Go language to connect to the database: Improving application performance and efficiency As applications develop and the number of users increases, data storage and processing become more and more important. In order to improve the performance and efficiency of applications, properly connecting and operating the database is a crucial part. As a fast, reliable, and highly concurrency development language, Go language has the potential to provide efficient performance when processing databases. This article will introduce how to use Go language to connect to the database and provide some code examples. Install database driver using Go language
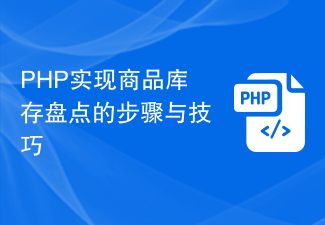
Steps and techniques for implementing product inventory in PHP In the e-commerce industry, product inventory management is a very important task. Timely and accurate inventory counting can avoid sales delays, customer complaints and other problems caused by inventory errors. This article will introduce the steps and techniques on how to use PHP to implement product inventory counting, and provide code examples. Step 1: Create a database First, we need to create a database to store product information. Create a database named "inventory" and then create a database named "prod
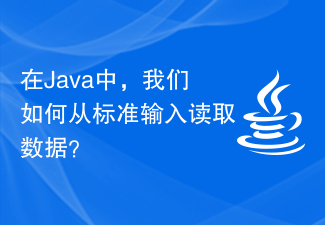
Standard input (stdin) can be represented by System.in in Java. System.in is an instance of InputStream class. This means that all its methods work on bytes, not strings. To read any data from keyboard we can use Reader class or Scanner class. Example 1importjava.io.*;publicclassReadDataFromInput{ publicstaticvoidmain(String[]args){ &nbs
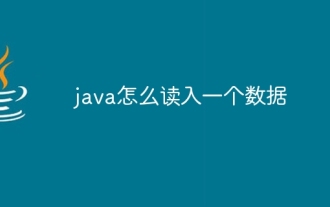
In Java, the way data is read depends on the data source and format. Common methods include: - **Reading data from the console:** Use the Scanner class to read data entered by the user. - **Reading data from files:** Use the BufferedReader and FileReader classes to read text files. For binary files, you can use the Files and Paths classes (Java 8 and above). - **Read data from the database: **Use JDBC (Java Database Connectivity) to connect to the relational database and execute queries. - **Read data from other sources:
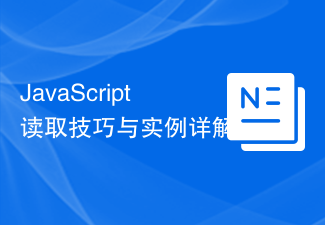
JavaScript is a programming language widely used in web development. It has many powerful functions and flexibility, allowing developers to implement various interactive effects and dynamic functions. In the daily development process, it is often necessary to read data from the page, operate elements or perform other operations. This article will introduce some reading techniques in JavaScript in detail and give detailed example codes. 1. Obtain elements by id. In JavaScript, you can obtain the characteristics of the page through the id attribute of the element.
