


In-depth understanding of the underlying implementation principles of singly linked lists in Go language
Go language is a fast, efficient, and strongly typed programming language. Its elegant syntax and rich standard library make it widely used in the Internet field. In Go language, singly linked list is a common data structure that can be used to store and organize data. This article will delve into the underlying implementation principles of singly linked lists in the Go language and give specific code examples.
Basic concept of singly linked list
Singly linked list is a basic data structure of a linear list, consisting of a series of nodes. Each node contains two parts: data and a pointer to the next node. . The advantage of a singly linked list is that insertion and deletion operations are efficient, but search operations are relatively inefficient.
In the Go language, we can use structures to define nodes of a singly linked list:
type Node struct { data int next *Node }
The above code defines a node structure containing integer data and a pointer to the next node. body. Next, we will implement several basic operations of a singly linked list: creation, insertion, deletion and printing.
Create a singly linked list
func createLinkedList() *Node { head := &Node{} return head }
The above code defines a function that creates a singly linked list, which returns an empty head node. Next, we will implement the insertion operation into a singly linked list.
Insert node
func insertNode(head *Node, data int) { newNode := &Node{data, nil} if head.next == nil { head.next = newNode } else { temp := head for temp.next != nil { temp = temp.next } temp.next = newNode } }
The above code defines a function that inserts a node, which inserts the new node to the end of the singly linked list. Next, we will implement the deletion operation of a singly linked list.
Delete Node
func deleteNode(head *Node, data int) { temp := head for temp.next != nil { if temp.next.data == data { temp.next = temp.next.next break } temp = temp.next } }
The above code defines a function to delete nodes, which deletes the corresponding node in the singly linked list based on the specified data. Finally, we will implement the operation of printing a singly linked list.
Print singly linked list
func printLinkedList(head *Node) { temp := head.next for temp != nil { fmt.Print(temp.data, " ") temp = temp.next } fmt.Println() }
The above code defines a function to print a singly linked list, which traverses the singly linked list starting from the head node and outputs the data of each node. Now, we can use the above code to create, insert, delete and print singly linked list.
func main() { head := createLinkedList() insertNode(head, 1) insertNode(head, 2) insertNode(head, 3) printLinkedList(head) deleteNode(head, 2) printLinkedList(head) }
The above code example creates a singly linked list containing data 1, 2, and 3, then deletes the node with data 2, and prints the final singly linked list result. Through the above code examples, we have an in-depth understanding of the underlying implementation principles of singly linked lists in the Go language and mastered the basic operations of singly linked lists. Xiduo readers can flexibly use this classic data structure of singly linked lists in practical applications.
The above is the detailed content of In-depth understanding of the underlying implementation principles of singly linked lists in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
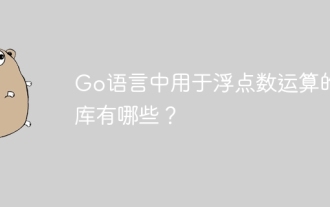
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
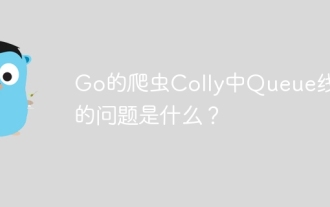
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
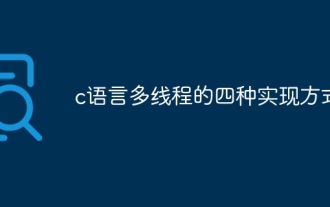
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
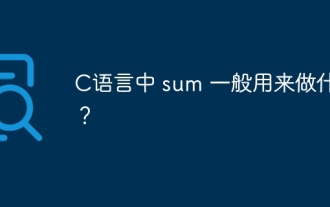
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
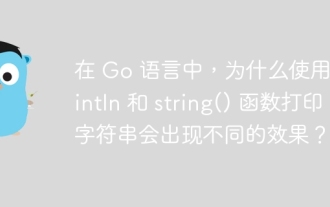
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
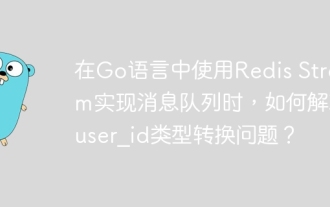
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
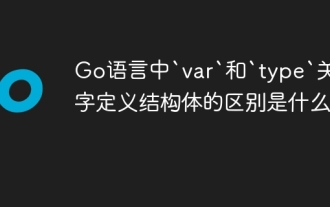
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
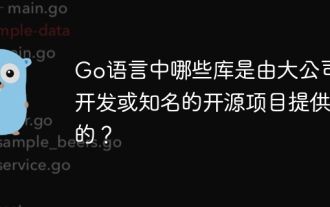
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
