How to get started with Go language development?
How to get started with Go language development?
Go language (also known as Golang) is an open source programming language developed by Google. It has the characteristics of fast compilation, static typing, concurrent programming, etc. It is suitable for developing high-performance back-end services, network applications, Cloud computing and other fields. For beginners who want to learn the Go language, it is crucial to master some basic concepts and grammatical rules. Next, we will introduce how to get started with Go language development through specific code examples.
- Install the Go language environment
First of all, before getting started with Go language development, we need to install the Go language environment. You can download the Go language installation package suitable for your operating system through the official website (https://golang.org/) and follow the prompts to complete the installation. After the installation is completed, you can enter the following command in the command line to check whether the installation is successful:
go version
If the Go language version number is displayed, the installation is successful.
- Writing the first Go program
Next, we will write a simple Go program to output "Hello, Go!".
// hello.go package main import "fmt" func main() { fmt.Println("Hello, Go!") }
You can use any text editor to create the above file named hello.go
, and then run the following command on the command line to compile and execute the program:
go run hello.go
If everything goes well, you will see the output as "Hello, Go!" on the command line.
- Variables and data types
The Go language is a statically typed language, so when using variables, their data types must be explicitly specified. The following are some common data types and variable declaration methods:
// 变量声明 var a int var b string // 变量赋值 a = 10 b = "Hello" // 初始化变量 var c int = 20 var d string = "World" // 简短声明 e := 30 f := "Go"
- Control flow
Go language supports common control flow statements, such as if-else
, for
, switch
, etc. The following is some sample code:
// if-else语句 if a > 0 { fmt.Println("a is positive") } else if a < 0 { fmt.Println("a is negative") } else { fmt.Println("a is zero") } // for循环 for i := 0; i < 5; i++ { fmt.Println(i) } // switch语句 switch b { case "Hello": fmt.Println("b is Hello") case "World": fmt.Println("b is World") default: fmt.Println("b is unknown") }
- Function
Function is the basic unit of Go language. The following is a simple function example:
func add(x, y int) int { return x + y } result := add(3, 5) fmt.Println(result) // 输出8
- Package
In the Go language, a group of related functions can be placed in a package to better organize the code. Here is a simple package example:
math.go
:
package math func Add(x, y int) int { return x + y } func Subtract(x, y int) int { return x - y }
main.go
:
package main import ( "fmt" "math" ) func main() { fmt.Println(math.Add(3, 5)) // 输出8 fmt.Println(math.Subtract(5, 2)) // 输出3 }
The above is A basic introductory guide, hoping to help beginners quickly get started with Go language development. Continue to learn more about the syntax and features of the Go language, and continuous practice and project implementation are the keys to improving your programming skills. I wish you smooth learning and happy programming!
The above is the detailed content of How to get started with Go language development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
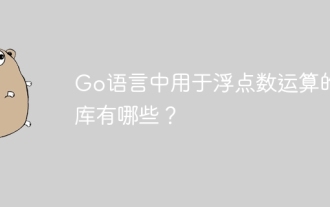
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
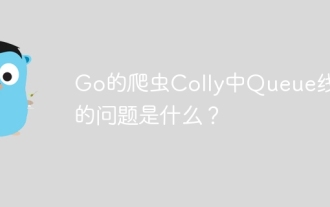
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
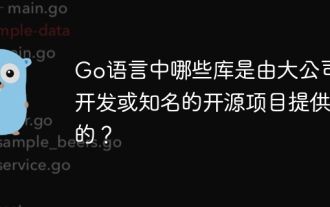
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
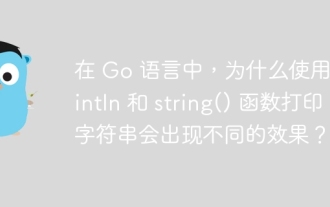
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
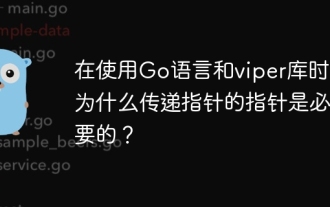
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
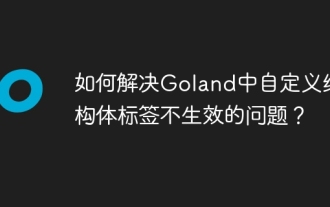
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
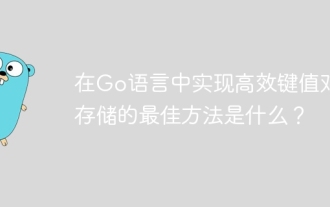
The correct way to implement efficient key-value pair storage in Go language How to achieve the best performance when developing key-value pair memory similar to Redis in Go language...
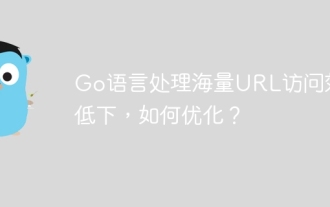
Performance optimization strategy for Go language massive URL access This article proposes a performance optimization solution for the problem of using Go language to process massive URL access. Existing programs from CSV...
