


The road to back-end development in Go language: achieving efficient and stable services
Go language back-end development has attracted much attention in recent years. Its efficient, stable features and good concurrency support make it one of the preferred languages for many developers. In this article, we will delve into how to implement efficient and stable back-end services through specific code examples to help readers better understand and apply the Go language for back-end development.
1. Introduction to Go language
Go language is an open source programming language developed by Google. The first stable version was released in 2009. It combines the efficiency of static languages and the ease of use of dynamic languages. It has the characteristics of fast compilation and extremely low memory usage. It is suitable for building high-performance and high-concurrency back-end services.
2. Initialize a simple Go backend service
First, we need to initialize a simple Go backend service for subsequent addition of functions and optimization. The following is a simple HTTP server example:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In the above example, we created an HTTP server that listens on port 8080 and returns "Hello, World!" when the root path is accessed. This is a very basic Go backend service, and we will gradually improve it next.
3. Achieve efficient concurrent processing
Go language inherently supports efficient concurrent processing, and concurrent task processing can be easily realized through goroutine and channel. The following is an example of using goroutine to handle concurrent requests:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { go func() { // 模拟处理耗时任务 for i := 0; i < 5; i++ { fmt.Println("Processing task", i) } }() fmt.Fprintf(w, "Task started") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In the above example, we created a goroutine in the handler function to handle time-consuming tasks to avoid blocking the main thread. This helps improve the throughput and responsiveness of the service.
4. Optimize service stability
In order to ensure the stability of the service, we need to add functions such as error handling, logging, and graceful shutdown. Here is a sample code that implements these functions:
package main import ( "fmt" "log" "net/http" "os" "os/signal" "syscall" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { go func() { sig := make(chan os.Signal, 1) signal.Notify(sig, os.Interrupt, syscall.SIGTERM) <-sig log.Println("Shutting down server...") os.Exit(0) }() http.HandleFunc("/", handler) err := http.ListenAndServe(":8080", nil) if err != nil { log.Fatal("Server error:", err) } }
In the above example, we added signal listening to gracefully shut down the server when a termination signal is received. At the same time, by recording logs and handling errors, potential problems can be better discovered and solved to ensure the stability of the service.
5. Summary
Through the above examples, we have deeply discussed how to achieve efficient and stable back-end services through Go language. Through reasonable concurrency processing, optimization and error handling, the performance and stability of the service can be improved to meet the needs of users. I hope this article can help readers better apply Go language for back-end development and achieve excellent back-end services.
The above is the detailed content of The road to back-end development in Go language: achieving efficient and stable services. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
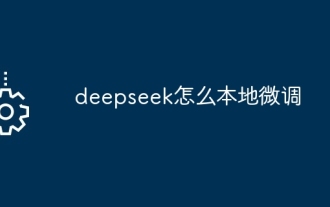
Local fine-tuning of DeepSeek class models faces the challenge of insufficient computing resources and expertise. To address these challenges, the following strategies can be adopted: Model quantization: convert model parameters into low-precision integers, reducing memory footprint. Use smaller models: Select a pretrained model with smaller parameters for easier local fine-tuning. Data selection and preprocessing: Select high-quality data and perform appropriate preprocessing to avoid poor data quality affecting model effectiveness. Batch training: For large data sets, load data in batches for training to avoid memory overflow. Acceleration with GPU: Use independent graphics cards to accelerate the training process and shorten the training time.
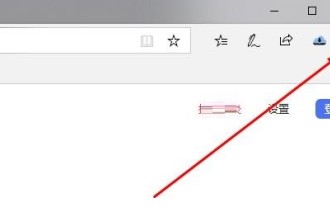
1. First, enter the Edge browser and click the three dots in the upper right corner. 2. Then, select [Extensions] in the taskbar. 3. Next, close or uninstall the plug-ins you do not need.
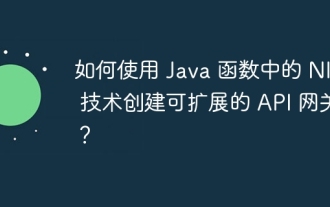
Answer: Using NIO technology you can create a scalable API gateway in Java functions to handle a large number of concurrent requests. Steps: Create NIOChannel, register event handler, accept connection, register data, read and write handler, process request, send response
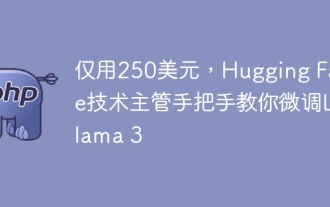
The familiar open source large language models such as Llama3 launched by Meta, Mistral and Mixtral models launched by MistralAI, and Jamba launched by AI21 Lab have become competitors of OpenAI. In most cases, users need to fine-tune these open source models based on their own data to fully unleash the model's potential. It is not difficult to fine-tune a large language model (such as Mistral) compared to a small one using Q-Learning on a single GPU, but efficient fine-tuning of a large model like Llama370b or Mixtral has remained a challenge until now. Therefore, Philipp Sch, technical director of HuggingFace
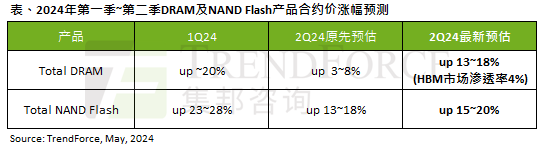
According to a TrendForce survey report, the AI wave has a significant impact on the DRAM memory and NAND flash memory markets. In this site’s news on May 7, TrendForce said in its latest research report today that the agency has increased the contract price increases for two types of storage products this quarter. Specifically, TrendForce originally estimated that the DRAM memory contract price in the second quarter of 2024 will increase by 3~8%, and now estimates it at 13~18%; in terms of NAND flash memory, the original estimate will increase by 13~18%, and the new estimate is 15%. ~20%, only eMMC/UFS has a lower increase of 10%. ▲Image source TrendForce TrendForce stated that the agency originally expected to continue to
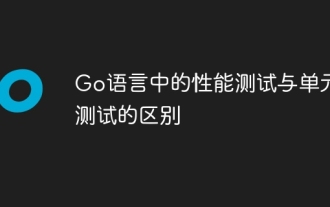
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
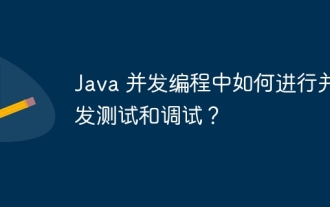
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
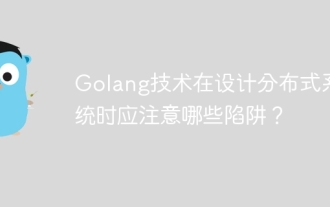
Pitfalls in Go Language When Designing Distributed Systems Go is a popular language used for developing distributed systems. However, there are some pitfalls to be aware of when using Go, which can undermine the robustness, performance, and correctness of your system. This article will explore some common pitfalls and provide practical examples on how to avoid them. 1. Overuse of concurrency Go is a concurrency language that encourages developers to use goroutines to increase parallelism. However, excessive use of concurrency can lead to system instability because too many goroutines compete for resources and cause context switching overhead. Practical case: Excessive use of concurrency leads to service response delays and resource competition, which manifests as high CPU utilization and high garbage collection overhead.
