


In-depth understanding of division operations in Go language: integer division and remainder
In-depth understanding of division operation in Go language: integer division and remainder
In Go language, division operation is an operator often used in our daily programming . Division operations mainly involve two concepts: integer division and remainder. In this article, we will delve into the division operation in Go language and provide specific code examples to help readers better understand these two concepts.
Integral division
Integral division refers to the situation where the quotient obtained after dividing two integers is also an integer. In the Go language, when using the /
operator to perform division operations, if both operands are of integer type, the result will also be of integer type. If either the divisor or the dividend is of type floating point, the result will also be of type floating point.
The following is a sample code that demonstrates the result in the case of integer division:
package main import "fmt" func main() { a := 10 b := 3 result := a / b fmt.Println(result) // 输出结果为3 }
In the above example, the variable a
is divided by the variable b
to get The result is 3. In fact, an integer division operation is performed here, and the result is directly taken as the integer part. It is important to note that the rounding rule for integer division results is to round to 0, that is, discard the decimal part instead of rounding.
Remainder
The remainder refers to the division remainder obtained after dividing two integers. In Go language, you can use the %
operator to get the remainder after dividing two integers.
The following is a sample code that demonstrates the calculation process of the remainder:
package main import "fmt" func main() { a := 10 b := 3 remainder := a % b fmt.Println(remainder) // 输出结果为1 }
In the above example, the variable a
is divided by the variable b
. The remainder is 1, that is, the remainder of dividing 10 by 3 is 1. The calculation process of the remainder is to divide the dividend by the divisor to get the quotient of the integer divisor, then multiply the quotient by the divisor and subtract the dividend to get the remainder.
Integers and remainders in division operations are important concepts we often use in programming, which can help us perform numerical calculations and logical judgments. Through the sample code provided in this article, I believe that readers have a deeper understanding of the division operation in the Go language and can apply it more flexibly to actual programming work.
The above is the detailed content of In-depth understanding of division operations in Go language: integer division and remainder. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


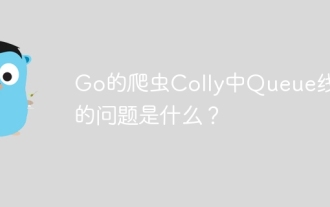
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
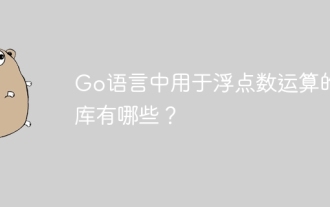
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
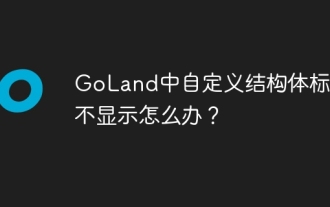
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
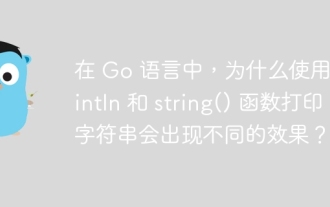
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
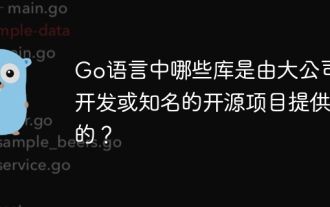
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
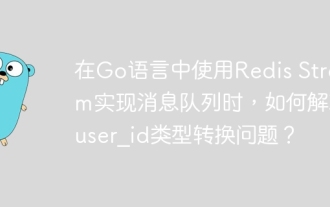
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
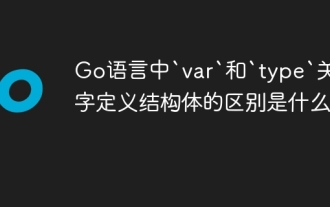
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
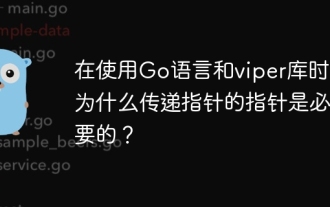
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
