Recommended selected excellent Go language projects
As a programming language, Go language has been widely used in various projects. It is loved by many developers for its efficiency, speed and simplicity. This article will recommend some excellent Go language projects to everyone and provide specific code examples.
Gorilla Mux
[Gorilla Mux](https://github.com/gorilla/mux) is a powerful Go language HTTP router. It supports regular expression-based URL matching, as well as flexible routing matching rules. The following is a simple example that demonstrates how to use Gorilla Mux to create a simple HTTP server and define several routes:
package main import ( "net/http" "github.com/gorilla/mux" ) func main() { r := mux.NewRouter() r.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Welcome to our website!")) }) r.HandleFunc("/products/{category}/{id:[0-9]+}", func(w http.ResponseWriter, r *http.Request) { vars := mux.Vars(r) w.Write([]byte("Category: " + vars["category"] + ", ID: " + vars["id"])) }) http.Handle("/", r) http.ListenAndServe(":8000", nil) }
Gorm
[Gorm](https://gorm.io /) is an excellent Go language ORM library used to simplify interaction with relational databases. It supports a variety of databases, including MySQL, PostgreSQL, SQLite, etc. The following is a simple example showing how to use Gorm to connect to a MySQL database and perform simple add, delete, modify and query operations:
package main import ( "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" ) type Product struct { ID uint Name string Price float64 } func main() { dsn := "username:password@tcp(localhost:3306)/dbname?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { fmt.Println("Failed to connect to database") return } db.AutoMigrate(&Product{}) // Create db.Create(&Product{Name: "Apple", Price: 1.5}) // Read var product Product db.First(&product, 1) // find product with id 1 fmt.Println(product) // Update db.Model(&product).Update("Price", 2.0) // Delete db.Delete(&product) }
Gin
[Gin](https://gin-gonic.com /) is a lightweight HTTP web framework with excellent performance and ease of use. The following is a simple example showing how to use Gin to create a simple HTTP server and define several routes:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.String(200, "Welcome to our website!") }) r.GET("/hello/:name", func(c *gin.Context) { name := c.Param("name") c.String(200, "Hello "+name) }) r.POST("/login", func(c *gin.Context) { username := c.PostForm("username") password := c.PostForm("password") c.JSON(200, gin.H{"username": username, "password": password}) }) r.Run(":8000") }
The above recommended projects are all very practical tools in the Go language development process. I hope they can Provide help to developers who are learning or using Go language. If interested, you can delve into more features and usage of these items.
The above is the detailed content of Recommended selected excellent Go language projects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


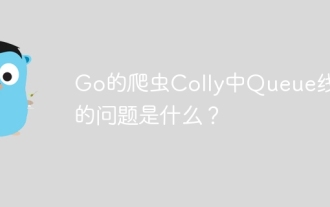
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
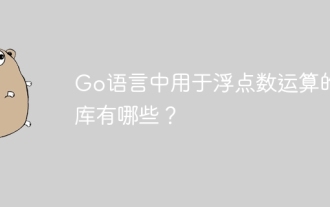
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
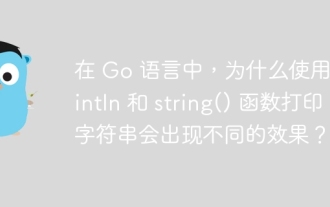
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
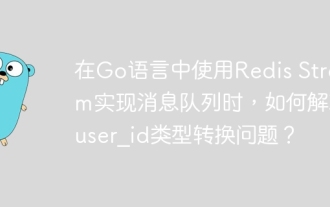
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
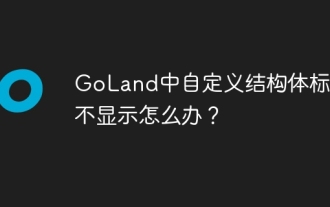
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
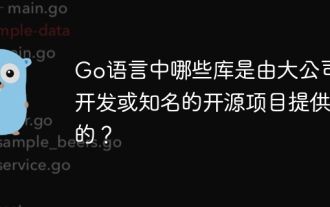
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
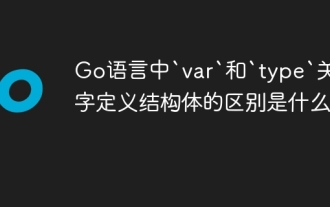
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
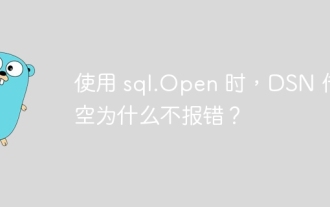
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
