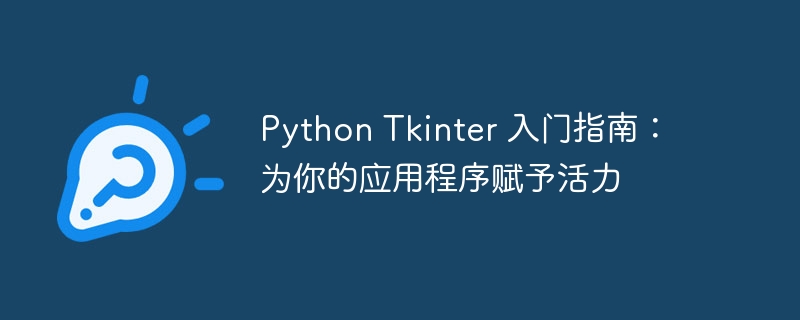
Step One: Tkinter Basics
-
Import Tkinter: Import Tk from the tkinter module, which is the main class of Tkinter.
-
Create the main window: Use Tk() to create the main window of the Tkinter application.
-
Add components:Use various component widgets (such as buttons, labels, text boxes) to build your GUI.
Step Two: Event Processing
-
Bind events: Bind event handler functions to component widgets in response to user interaction.
-
Event object: Each event has an event object containing event information.
-
Handling events: Handle user input and update the GUI in event handler functions.
Step Three: Layout Management
-
Layout manager: Use the layout manager to manage the layout of component widgets in the window.
-
Pack: Pack the component widget into its container, filling the available space.
-
Grid: Arrange component widgets into a grid, with clear rows and columns.
Step 4: Interactive interface
-
Get input: Use the get() method to get user input from the component widget.
-
Set value: Use the set() method to set the value of the component widget.
-
Validate input: Use validatecommand to validate user input and provide feedback.
Step 5: Advanced Concepts
-
Custom widgets: Create your own widgets to extend the functionality of Tkinter.
-
Images and Canvas: Use the canvas widget to draw images and graphics.
-
Menus and Toolbars: Add menus and Toolsbars to provide user functionality.
Sample Application
The following is a simple Tkinter application example:
import tkinter as tk
root = tk.Tk()
root.title("python Tkinter 入门")
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
button = tk.Button(root, text="点击我")
button.pack()
root.mainloop()
Copy after login
Best Practices
- Keep the code simple and maintainable.
- Use layout managers to create clean GUIs.
- Use event handling to respond to user input.
- Validate user input.
- Leverage the power of Tkinter to create interactive applications.
By following this guide, you can master the basics of Tkinter and start creating your own interactive desktop applications.
The above is the detailed content of Getting Started with Python Tkinter: Bringing Your Applications to Life. For more information, please follow other related articles on the PHP Chinese website!