How to determine whether a date is the previous day in Go language?
Title: How to determine whether a date is the previous day in Go language?
In daily development, we often encounter situations where we need to determine whether the date is the previous day. In the Go language, we can implement this function through time calculation. The following will be combined with specific code examples to demonstrate how to determine whether the date is the previous day in Go language.
First, we need to import the time package in the Go language. The code is as follows:
import ( "time" )
Next, we define a function IsYesterday
, which accepts a time object as a parameter , used to determine whether the time is yesterday. The specific code is as follows:
func IsYesterday(t time.Time) bool { yesterday := time.Now().AddDate(0, 0, -1) return t.Year() == yesterday.Year() && t.Month() == yesterday.Month() && t.Day() == yesterday.Day() }
In the above code, we first obtain the time object of the day before the current time yesterday
, and then determine the incoming time object t
Is it the same as the previous day? If the year, month, and day are the same, true
is returned, otherwise false
is returned.
Next, we can write a simple test function to verify whether the above code is correct. The specific code is as follows:
func main() { today := time.Now() yesterday := time.Now().AddDate(0, 0, -1) fmt.Println("Today:", today) fmt.Println("Is yesterday?:", IsYesterday(today)) // 输出 false fmt.Println("Yesterday:", yesterday) fmt.Println("Is yesterday?:", IsYesterday(yesterday)) // 输出 true }
In the above test function, we printed the current time and the previous time respectively. The time of day, and the IsYesterday
function is called to determine whether the two times are the previous day. Finally, the corresponding output results will be obtained, which verifies the correctness of the date judgment code we wrote.
In summary, through the above code examples, we have learned how to determine whether a date is the previous day in Go language. This method can easily help us handle date-related logic and improve the readability and flexibility of the code. I hope readers can master this technique and use it flexibly in actual project development.
The above is the detailed content of How to determine whether a date is the previous day in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
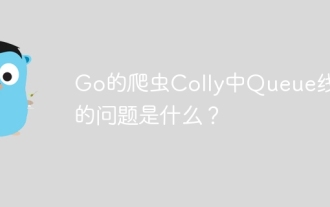
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
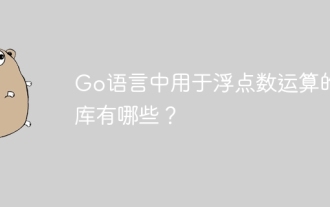
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
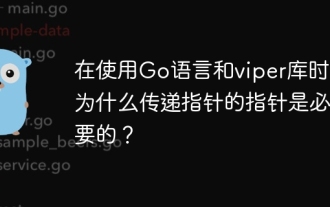
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
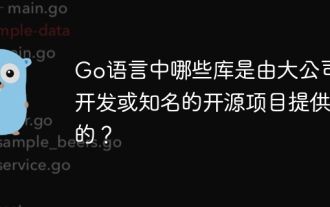
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
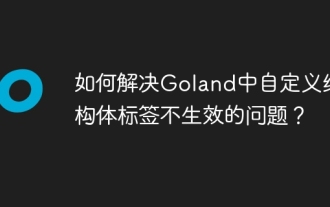
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
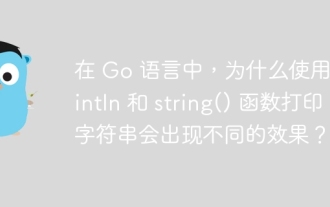
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
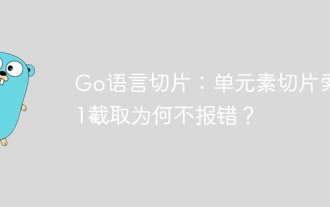
Go language slice index: Why does a single-element slice intercept from index 1 without an error? In Go language, slices are a flexible data structure that can refer to the bottom...
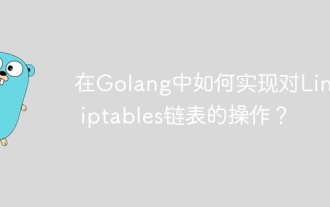
Using Golang to implement Linux...
