PHP transaction error troubleshooting and solutions
PHP transaction error troubleshooting and solutions
When developing a website or application, database operations are an essential part. In order to ensure data integrity and consistency, we often use transactions to handle database operations. Transactions can ensure that a set of operations either all succeed or all fail, thereby avoiding abnormal data states. However, sometimes when using PHP for database operations, you may encounter transaction-related errors. This article will explore some common PHP transaction errors and give corresponding solutions, along with specific code examples.
- Error 1: The transaction was not committed or rolled back correctly
This is one of the most common errors. When using transactions, be sure to ensure that the transaction is either committed or rolled back after all operations are completed, otherwise data exceptions will occur.
The following is a sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
In the above code, when the operation in the try block is executed successfully, $conn->commit() is called to commit the transaction; if an exception occurs, execute The code in the catch block rolls back the transaction. Be sure to commit or rollback the transaction after the operation is completed to ensure data consistency.
- Error 2: Transaction nesting is incorrect
Sometimes we will nest one transaction within another transaction. In this case Be sure to handle commits and rollbacks of nested transactions correctly.
The following is a sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
|
In the above code, we demonstrate the situation where an inner transaction is nested in an outer transaction. Be sure to handle commit and rollback of internal and external transactions correctly to ensure data integrity.
- Error 3: Transaction timeout or lockup
Under concurrent operations, if the transaction holds the lock, but not within a reasonable time Releasing the lock within the transaction may result in transaction timeout or lockout.
The following is a sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
In the above code, we set the value of innodb_lock_wait_timeout to avoid transaction timeout or lockout problems. Be sure to handle the release of transaction locks carefully in a concurrent environment.
Summary:
This article introduces some common scenarios of transaction errors in PHP, and gives corresponding solutions and code examples. In database operations, it is very important to ensure the correct commit and rollback of transactions to ensure data integrity and consistency. I hope readers can gain a deeper understanding of PHP transaction errors through this article, thereby improving the efficiency and stability of database operations.
The above is the detailed content of PHP transaction error troubleshooting and solutions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




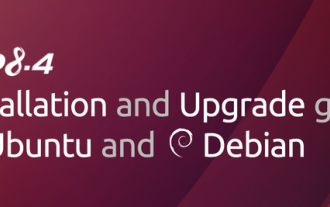
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
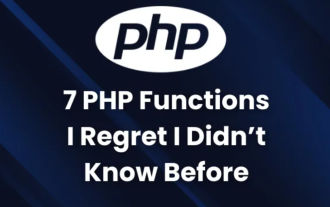
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
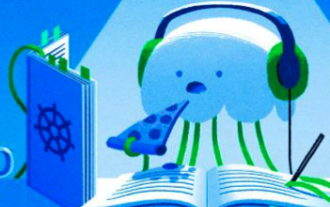
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
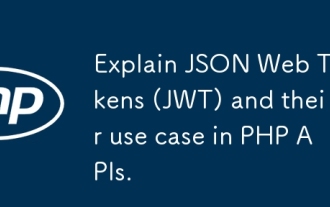
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
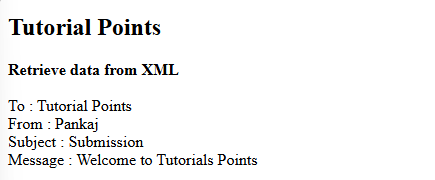
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
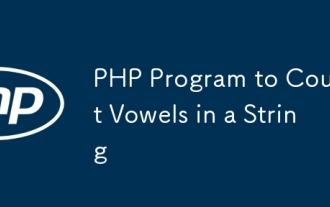
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
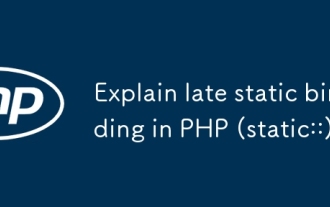
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
