


Why is it difficult to implement collection-like functions in Go language?
It is difficult to implement collection-like functions in the Go language, which is a problem that troubles many developers. Compared with other programming languages such as Python or Java, the Go language does not have built-in collection types, such as set, map, etc., which brings some challenges to developers when implementing collection functions.
First of all, let us take a look at why it is difficult to directly implement collection-like functions in the Go language. In the Go language, the most commonly used data structures are slice and map. They can perform collection-like functions, but they are not collection types in the traditional sense. For example, slice is an array that can grow dynamically, and map is a data structure of key-value pairs. Although they can be used to simulate the functions of sets, they do not have some features of set types, such as mathematical operations on sets (union , intersection, difference set, etc.).
In actual development, if we want to implement collection-like functions, we may need to define our own structure to encapsulate slice or map, and write some methods to implement collection operations. The following is a simple code example that shows how to use structures and methods to implement a simple set data structure:
package main import "fmt" type Set struct { data map[string]struct{} } func NewSet() *Set { return &Set{data: make(map[string]struct{})} } func (s *Set) Add(item string) { s.data[item] = struct{}{} } func (s *Set) Remove(item string) { delete(s.data, item) } func (s *Set) Contains(item string) bool { _, exists := s.data[item] return exists } func (s *Set) Size() int { return len(s.data) } func (s *Set) Intersection(other *Set) *Set { intersectionSet := NewSet() for key := range s.data { if other.Contains(key) { intersectionSet.Add(key) } } return intersectionSet } func main() { set1 := NewSet() set1.Add("apple") set1.Add("banana") set2 := NewSet() set2.Add("banana") set2.Add("cherry") // 求交集 intersectionSet := set1.Intersection(set2) fmt.Println("Intersection:", intersectionSet.data) }
In the above code, we define a Set structure, which contains some basic Collection operation methods such as Add, Remove, Contains, Size, and Intersection. Through these methods, we can implement basic operations on sets and easily perform operations such as intersection.
Although it is relatively complicated to implement collection-like functions in the Go language, we can still implement collection-like data structures and operations by defining custom structures and methods. Although this approach requires more code and work, it also provides us with greater flexibility and control. I hope that this example can help readers better understand the methods and challenges of implementing collection-like functions in the Go language.
The above is the detailed content of Why is it difficult to implement collection-like functions in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
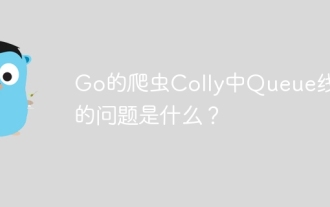
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
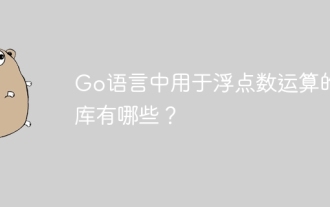
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
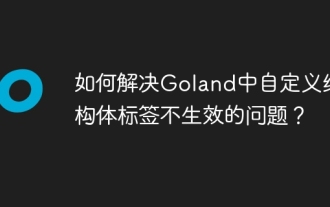
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
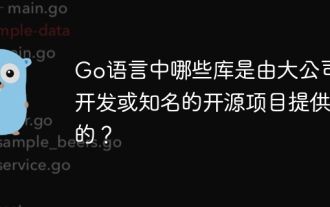
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
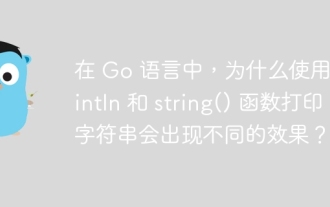
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
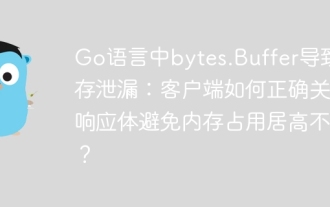
Analysis of memory leaks caused by bytes.makeSlice in Go language In Go language development, if the bytes.Buffer is used to splice strings, if the processing is not done properly...
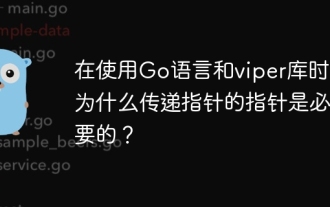
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
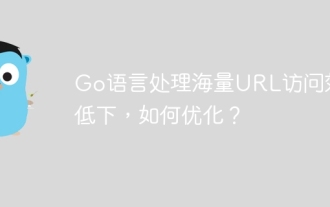
Performance optimization strategy for Go language massive URL access This article proposes a performance optimization solution for the problem of using Go language to process massive URL access. Existing programs from CSV...
