


Become a master of Java exception handling: Take control of errors in your code
Becoming a master of Java exception handling is a must for every Java programmer. In the process of writing code, it is inevitable to encounter various errors and exceptions. How to handle these exceptions efficiently can not only improve the quality of the code, but also enhance the robustness and stability of the program. This article will delve into the relevant knowledge of Java exception handling, help readers master the skills and methods of exception handling, and become a master of Java exception handling.
2. Master the exception propagation mechanism
As an exception propagates through the program, it moves up the call stack. If the exception is not handled in the code, it will be propagated to the method that called it, and so on. Understanding exception propagation mechanisms is critical to ensuring exceptions are handled appropriately.
3. Use try-catch-finally block
try-catch-finally
Blocks are the preferred mechanism for handling exceptions in Java. The try
block contains the code that needs to be executed, while the catch
block handles specific types of exceptions. finally
The block is always executed regardless of whether an exception occurs, and is usually used to release resources or perform cleanup operations.
4. Use throw statements
When a method cannot handle an exception from itself, it can be thrown using the throw
statement. This will cause the exception to propagate up the call stack until a suitable method is found to handle it.
5. Custom exception
Custom exceptions allow you to create specific exception classes for specific error conditions. Custom exceptions should inherit from the Exception
or Error
class and provide additional information about the error.
6. Handling common exceptions
It is important to be familiar with common exception types in Java, such as NullPointerException
, IndexOutOfBoundsException
, and IllegalArgumentException
. Understanding the root cause and handling of these exceptions will improve the robustness of your code.
7. Log recording exception
Logging exceptions to a log file is a valuable tool for identifying, investigating, and resolving errors. Capturing exception details using a logging library such as Log4j or SLF4J can help diagnose problems.
8. Use assertions
Assertions are Boolean expressions used in code to check expected conditions. If the assertion fails, an AssertionError
exception is thrown. Assertions can help detect errors in advance and prevent your program from running in unexpected states.
9. Unit Test
Writing UnitsTestsYou can verify the behavior of your code under various inputs and conditions, including exceptions. Unit tests help ensure exceptions are handled correctly and prevent errors from spreading.
10. Continuous learning
Exception handling is an evolving field. As Java versions update and new technologies emerge, it's important to stay up to date on best practices. Keep your knowledge current by reading documentation, attending conferences, and interacting with other developers.
The above is the detailed content of Become a master of Java exception handling: Take control of errors in your code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

In the Java development process, exception handling has always been a very important topic. When an exception occurs in the code, the program often needs to catch and handle the exception through exception handling to ensure the stability and security of the program. One of the common exception types is the AssertionError exception. This article will introduce the meaning and usage of AssertionError exception to help readers better understand and apply Java exception handling. 1. The meaning of AssertionError exception Asserti

ClassNotFoundException exception in Java is one of the common problems in development. In Java development, it is a very common practice to obtain an instance of a class through the class name, but if the class to be loaded is not found, a ClassNotFoundException exception will be thrown. So, what are the common causes of ClassNotFoundException exceptions? The class path is incorrect. In Java, when a class needs to be loaded, the JV
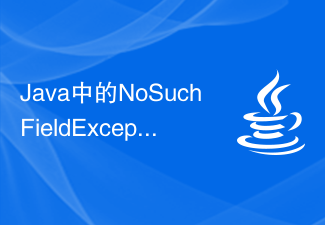
Java is one of the most widely used programming languages in the world, and exception handling is a very important part of the Java programming process. This article will introduce the NoSuchFieldException exception in Java, how it is generated and how to deal with it. 1. Definition of NoSuchFieldException NoSuchFieldException is a Checked exception in Java, which means it is thrown when the specified field is not found.
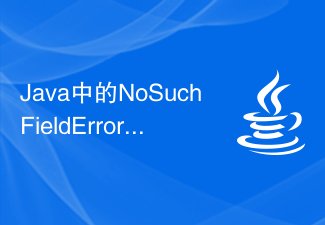
Java is a popular high-level programming language that enables developers to easily create a variety of applications. However, just like any other programming language, some errors and exceptions may occur during coding in Java. One of the common exceptions is NoSuchFieldError. This article explains the causes of this anomaly, how to avoid it, and how to deal with it. What is the NoSuchFieldError exception? Let’s first understand the NoSuchFieldError exception. Simple
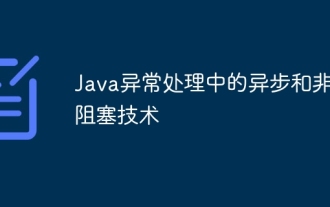
Asynchronous and non-blocking techniques can be used to complement traditional exception handling, allowing the creation of more responsive and efficient Java applications: Asynchronous exception handling: Handling exceptions in another thread or process, allowing the main thread to continue executing, avoiding blocking. Non-blocking exception handling: involves event-driven exception handling when an I/O operation goes wrong, avoiding blocking threads and allowing the event loop to handle exceptions.
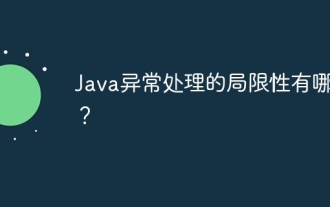
Limitations of Java exception handling include the inability to catch virtual machine and operating system exceptions. Exception handling can mask deeper problems. Nested exceptions are difficult to debug. Exception handling code reduces readability. Runtime checked exceptions have a performance overhead.
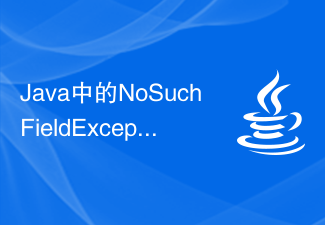
The NoSuchFieldException exception in Java refers to the exception thrown when trying to access a non-existent field (Field) during reflection. In Java, reflection allows us to manipulate classes, methods, variables, etc. in the program through code, making the program more flexible and scalable. However, when using reflection, if the accessed field does not exist, a NoSuchFieldException will be thrown. NoSuchFieldException
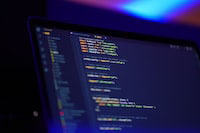
Types of exceptions There are two main types of exceptions in Java: CheckedExceptions: Exceptions that are forced to be handled by the compiler, usually indicating serious errors, such as file non-existence or database connection failure. UncheckedExceptions: Exceptions that the compiler is not forced to handle, usually indicating programming errors, such as array index out of bounds or null pointer reference. Exception handling mechanismException handling uses the following keywords: try-catch-finally block: used to surround code that may throw an exception. try block: Contains code that may throw exceptions. Catch block: used to catch specific types of exceptions and contains code to handle exceptions. finally block: start
