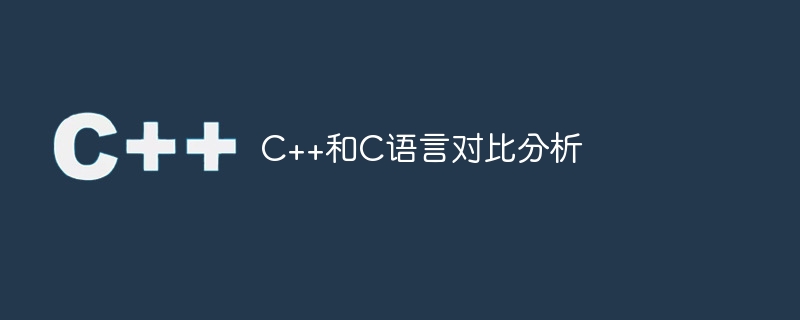
Comparative analysis of C and C language
C and C language are both widely used programming languages. They have many similarities, and there are also some significant differences. difference. This article will conduct a comparative analysis of these two languages, discuss them from the aspects of syntax features, object-oriented programming, pointer use, standard libraries, etc., and provide specific code examples for explanation.
1. Syntax Features
- C Language:
C language is a structured programming language, with functions as the main organizational unit, and has a concise grammatical structure and relatively simple structure. Low abstraction ability. The syntax of C language is relatively simple, mainly including basic data types, operators, flow control statements, etc.
- C Language:
C is an object-oriented programming language developed on the basis of C language. In addition to inheriting the grammatical features of C language, it also adds classes, objects, inheritance, polymorphism, etc. Object-oriented features. The syntax of C language is more complex, with higher abstraction capabilities and flexibility.
Specific code examples:
//C语言示例
#include <stdio.h>
int main() {
int a = 5;
printf("Hello World: %d
", a);
return 0;
}
Copy after login
//C++语言示例
#include <iostream>
using namespace std;
int main() {
int a = 5;
cout << "Hello World: " << a << endl;
return 0;
}
Copy after login
2. Object-oriented programming
- C language:
C language is a procedural programming language. It does not support the concept of object-oriented programming and does not have object-oriented features such as classes, objects, and inheritance. In C language, data encapsulation can be achieved through structures. - C language:
C is a language that fully supports object-oriented programming. It has features such as classes, objects, inheritance, and polymorphism, which can better realize the encapsulation and reuse of data and functions.
Specific code example:
//C++面向对象示例
#include <iostream>
using namespace std;
class Shape {
public:
virtual void display() {
cout << "This is a shape." << endl;
}
};
class Circle : public Shape {
public:
void display() {
cout << "This is a circle." << endl;
}
};
int main() {
Shape *s = new Circle();
s->display();
return 0;
}
Copy after login
3. Pointer use
- C language:
Pointer is a very important data in C language Type, the data in the memory can be directly accessed through pointers to realize the operation and transfer of data. In C language, memory allocation and release need to be managed manually. - C language:
C inherits the pointer characteristics of the C language, but introduces the concept of reference, which can simplify the operation of pointers and improve the readability of the code. In addition, C also provides tools such as smart pointers to facilitate memory management.
Specific code examples:
//指针使用示例
#include <iostream>
using namespace std;
int main() {
int *ptr = new int(10);
cout << "Value: " << *ptr << endl;
delete ptr;
return 0;
}
Copy after login
4. Standard library
- C language:
The standard library of C language includes stdio.h, stdlib Header files such as .h and string.h provide a rich set of basic functions and data types, but do not support object-oriented encapsulation and template programming. - C language:
C's standard library is richer and more powerful than C language, including header files such as iostream, string, vector, etc., providing a rich container class and algorithm library, and supporting template programming and object-oriented characteristics.
Specific code examples:
//标准库示例
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
for(int num : nums) {
cout << num << " ";
}
return 0;
}
Copy after login
To sum up, there are some obvious differences between C and C languages in terms of syntax features, object-oriented programming, use of pointers, and standard libraries. Which language to choose should be determined based on specific application scenarios and needs. I hope that the comparative analysis in this article can help readers better understand and use these two programming languages.
The above is the detailed content of Comparative analysis of C++ and C language. For more information, please follow other related articles on the PHP Chinese website!