How to retain the number of decimal places in C++
In C, retaining several decimal places usually involves formatting the output. This can be achieved by using std::setprecision and std::fixed from the I/O streams library. You can use std::cout and I/O stream formatting, std::stringstream, std::round or std::floor/std::ceil for rounding, and use the C-style printf function.
In C, retaining a few decimal places usually involves formatting the output, which can be done by using std from the I/O stream library: :setprecision and std::fixed to achieve this. The following are some ways to preserve the decimal places:
1. Use std::cout and I/O stream formatting
You You can use std::cout with std::fixed and std::setprecision to set the output format.
cpp
##
#include <iostream> #include <iomanip> // 包含 setprecision 和 fixed int main() { double value = 3.141592653589793; std::cout << std::fixed << std::setprecision(2) << value << std::endl; // 输出: 3.14 return 0; }
In this example, std::fixed makes sure to use fixed Point notation, and std::setprecision(2) sets the number of digits after the decimal point to 2.
2, Use std::stringstream
If you need to store the formatted string in a variable instead of outputting it directly To the console you can use std::stringstream.
cpp
###include <sstream>
#include <iomanip>
#include <string>
int main() {
double value = 3.141592653589793;
std::stringstream ss;
ss << std::fixed << std::setprecision(2) << value;
std::string formatted_value = ss.str(); // formatted_value 现在包含 "3.14"
return 0;
}
If you want to round to a specified number of decimal places, you can use the std::round function. Please note that std::round accepts a floating point number multiplied by a power of 10 as an argument, so you need to calculate accordingly based on the number of decimal places required.
cpp
#include <cmath> // 包含 round 函数
#include <iostream>
int main() {
double value = 3.141592653589793;
double rounded_value = std::round(value * 100.0) / 100.0; // 四舍五入到小数点后两位
std::cout << rounded_value << std::endl; // 输出: 3.14
return 0;
}
4. Use C-style formatted output
Although C recommends using I/O streams for formatting, you can also use C style printf function.
cpp
##
#include <cstdio> int main() { double value = 3.141592653589793; printf("%.2f\n", value); // 输出: 3.14 return 0; }
These methods can be used to retain several decimal places in C. Which method you choose depends on your specific needs and which programming style you prefer.
The above is the detailed content of How to retain the number of decimal places in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


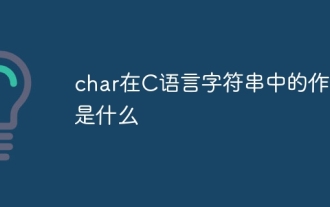
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
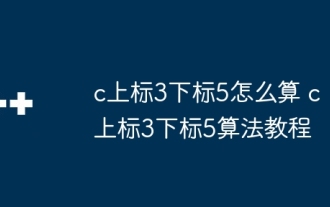
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
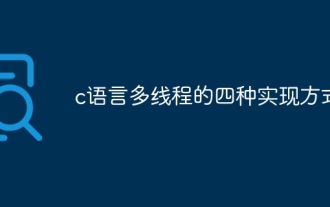
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
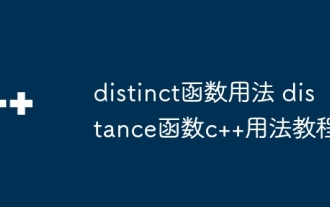
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
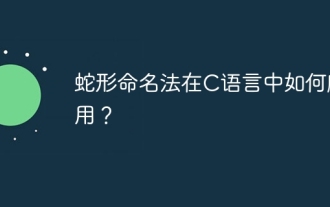
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
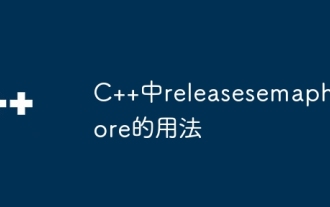
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
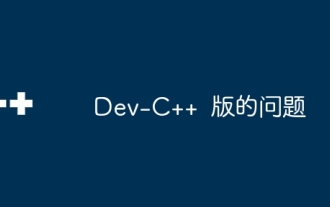
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
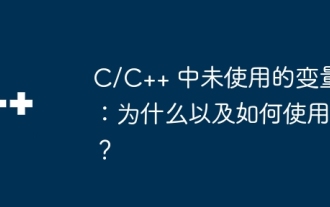
In C/C code review, there are often cases where variables are not used. This article will explore common reasons for unused variables and explain how to get the compiler to issue warnings and how to suppress specific warnings. Causes of unused variables There are many reasons for unused variables in the code: code flaws or errors: The most direct reason is that there are problems with the code itself, and the variables may not be needed at all, or they are needed but not used correctly. Code refactoring: During the software development process, the code will be continuously modified and refactored, and some once important variables may be left behind and unused. Reserved variables: Developers may predeclare some variables for future use, but they will not be used in the end. Conditional compilation: Some variables may only be under specific conditions (such as debug mode)
