


PHP programming tips: How to deal with the last semicolon situation
PHP Programming Tips: How to Handle the Last Semicolon
In PHP programming, we often encounter situations where we need to handle the last semicolon. Especially in loops and conditional statements, it is easy to cause program errors by writing one less or one more semicolon. In order to avoid this situation, we can adopt some programming techniques to handle the last semicolon situation.
The following are some common techniques and code examples for handling the last semicolon:
1. Use if statements to determine the last semicolon
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
In the above code example , we use an if statement after the loop ends to determine whether the last semicolon is redundant, and if so, remove it.
2. Use the implode function to connect array elements
1 2 3 4 5 6 7 |
|
In the above code example, we use the implode function to connect the array elements and add a comma and between each element spaces, so there is no need to deal with the last semicolon.
3. Use the rtrim function to remove the semicolon at the end of the string
1 2 3 4 5 6 7 8 9 10 11 12 |
|
In the above code example, we use the rtrim function to remove the extra semicolon at the end of the string before outputting.
Summary
In PHP programming, dealing with the last semicolon is a common problem. Program errors can be effectively avoided by using techniques such as using if statements to judge, using the implode function to connect array elements, or using the rtrim function to remove the semicolon at the end of a string. Choosing the right method can make our code more concise and readable, and avoid unnecessary errors. Hopefully the above tips and examples will be helpful to you when dealing with the last semicolon in PHP programming.
The above is the detailed content of PHP programming tips: How to deal with the last semicolon situation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


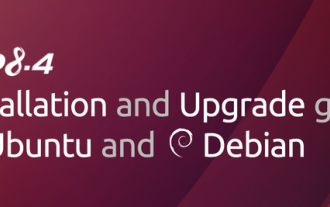
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
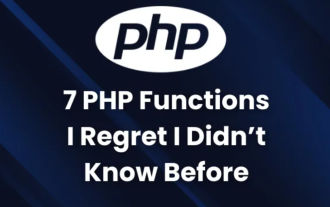
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
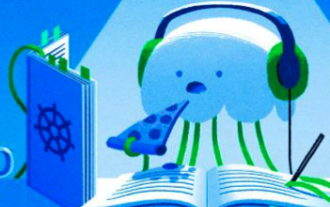
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
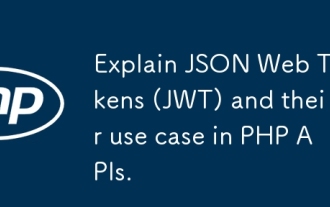
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
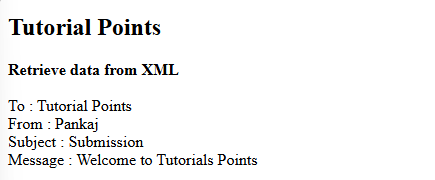
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
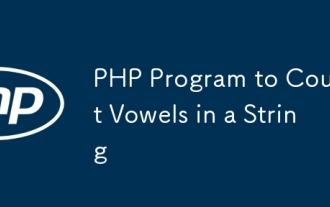
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
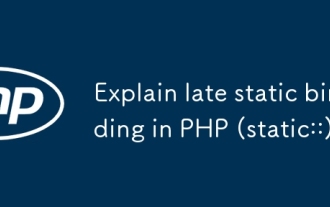
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
