Explore the advanced features of fn in Go language
Explore the advanced features of fn in Go language
As an efficient, fast and concise programming language, Go language is deeply loved by developers. In the Go language, functions are first-class citizens and have some advanced features. This article will explore them one by one.
- Anonymous Function
Go language supports anonymous functions, that is, without naming the function when declaring it, directly assign the function to a variable, or Use it directly where you need it. Anonymous functions are often used to implement closures and other scenarios. For example:
package main import "fmt" func main() { add := func(a, b int) int { return a + b } result := add(3, 5) fmt.Println(result) // 输出:8 }
- Multiple Return Values
The Go language allows functions to return multiple values, which is particularly useful when handling errors and exceptions. For example:
package main import "fmt" func divAndMod(a, b int) (int, int) { return a / b, a % b } func main() { div, mod := divAndMod(10, 3) fmt.Printf("10 ÷ 3 = %d, 10 %% 3 = %d ", div, mod) // 输出:10 ÷ 3 = 3, 10 % 3 = 1 }
- Functions as Parameters and Return Values
In the Go language, a function can be passed as a parameter to another function, It can also be used as the return value of another function. This feature makes functions more flexible. For example:
package main import "fmt" func applyFunc(a, b int, fn func(int, int) int) int { return fn(a, b) } func add(a, b int) int { return a + b } func main() { result := applyFunc(3, 5, add) fmt.Println(result) // 输出:8 }
- defer statement (Defer Statement)
The defer statement is used to delay the execution of functions, and is usually used in scenarios such as resource release and file closing. The defer statement will be executed after the function containing it has completed execution. For example:
package main import "fmt" func readFile() { file := openFile("example.txt") defer closeFile(file) // 读取文件内容 } func openFile(filename string) *File { // 打开文件 return file } func closeFile(file *File) { // 关闭文件 } func main() { readFile() }
- Function Closure
Closure means that a function can access variables in its outer scope, even if these variables are defined outside the function . Closures can implement object-like functions in Go language. For example:
package main import "fmt" func outerFunc() func() int { count := 0 return func() int { count++ return count } } func main() { counter := outerFunc() fmt.Println(counter()) // 输出:1 fmt.Println(counter()) // 输出:2 }
Summary
Through the above examples, we have explored some advanced features of functions in Go language, including anonymous functions, multiple return values, functions as parameters and return values, and defer statements and function closures. These features make Go language functions more flexible and powerful, able to meet various programming needs. I hope this article will help you gain a deeper understanding of the features of Go language functions.
The above is the detailed content of Explore the advanced features of fn in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


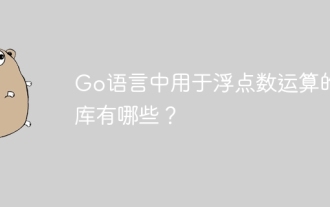
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
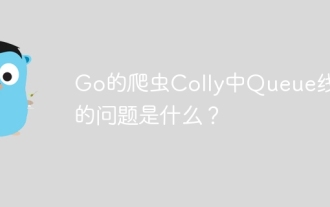
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
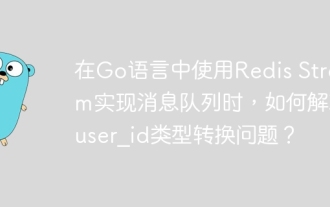
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
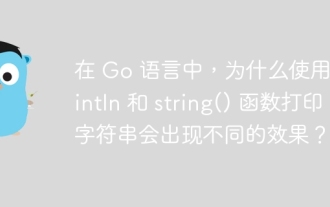
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
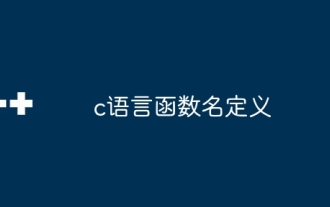
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
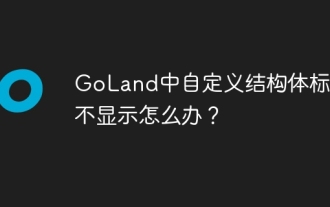
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
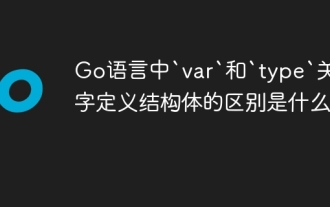
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
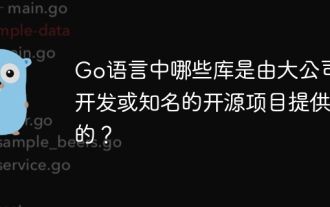
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
