Explore the disadvantages and solutions of Go language
Disadvantages of Go language and solutions
As a fast, reliable and efficient programming language, Go language has been popular in recent years. The Internet field has been widely used and recognized. However, just like any other programming language, Go has some disadvantages that may present some challenges for some developers. This article will explore the disadvantages of the Go language and propose ways to solve these disadvantages, along with some specific code examples to help readers better understand.
1. Lack of generic support
As a statically typed language, Go language has weak support for generics, which makes it a bit clumsy when dealing with some common data structures. Frequently perform type assertions or use interface{} to achieve generic effects.
Solution: Generic functions can be simulated through interfaces, reflection or code generation. Next, a simple example will be used to illustrate how to use the interface to implement a general slice filter function.
package main import ( "fmt" ) type FilterFunc func(int) bool func Filter(slice []int, filter FilterFunc) []int { var result []int for _, v := range slice { if filter(v) { result = append(result, v) } } return result } func main() { slice := []int{1, 2, 3, 4, 5, 6} evenFilter := func(num int) bool { return num%2 == 0 } evenNumbers := Filter(slice, evenFilter) fmt.Println(evenNumbers) // Output: [2 4 6] }
2. Exception handling is more cumbersome
The error handling mechanism in Go language adopts an explicit method and needs to transfer error information through return values, which makes writing a large amount of error handling The code seems redundant and cumbersome.
Solution: You can simplify the exception handling process by using keywords such as defer, panic, and recover. The following is a simple example to demonstrate how to use panic and recover to handle exceptions.
package main import ( "fmt" ) func Divide(a, b int) int { defer func() { if err := recover(); err != nil { fmt.Println("发生了除零错误:", err) } }() if b == 0 { panic("除数不能为零") } return a / b } func main() { result := Divide(6, 3) fmt.Println("结果:", result) result = Divide(8, 0) fmt.Println("结果:", result) }
3. Performance optimization needs to be handled with caution
Although the Go language is famous for its efficient concurrency model and garbage collector, in some specific scenarios, performance optimization still requires developers to Be careful as over-optimization may lead to reduced code readability and maintainability.
Solution: When optimizing performance, it is necessary to analyze and test according to specific scenarios to avoid premature optimization and excessive optimization. In the following example, we show how to use Go's pprof tool for performance analysis.
package main import ( "fmt" "log" "net/http" _ "net/http/pprof" ) func fib(n int) int { if n <= 1 { return n } return fib(n-1) + fib(n-2) } func main() { go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }() fmt.Println("服务器已启动,请访问http://localhost:6060/debug/pprof/进行性能分析") result := fib(30) fmt.Println("斐波那契数列第30项:", result) }
Through the above examples, we have demonstrated some disadvantages and solutions of the Go language, and provided specific code examples to help readers better understand. As with any programming language, mastering its strengths and weaknesses and finding appropriate solutions are essential skills to become a good developer. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of Explore the disadvantages and solutions of Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


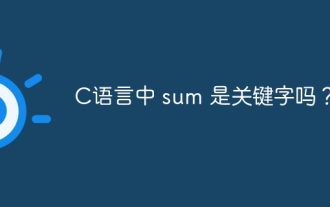
The sum keyword does not exist in C language, it is a normal identifier and can be used as a variable or function name. But to avoid misunderstandings, it is recommended to avoid using it for identifiers of mathematical-related codes. More descriptive names such as array_sum or calculate_sum can be used to improve code readability.
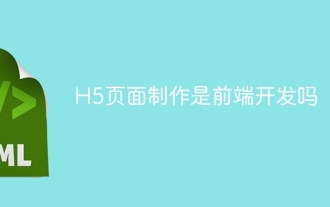
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
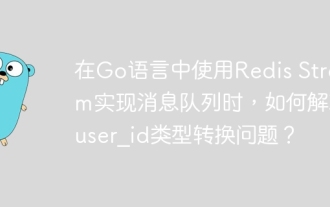
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
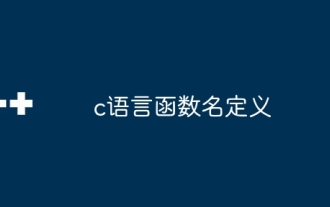
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
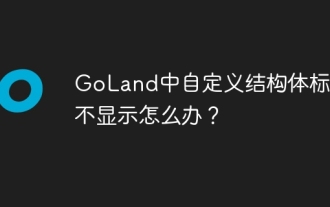
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
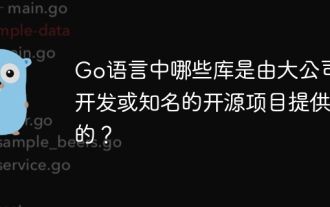
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
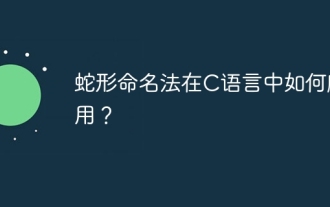
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
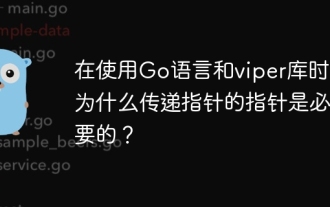
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
