Correct usage of POST request in PHP
The use of POST requests in PHP is a common operation in website development. Data can be sent to the server through POST requests, such as form data, user information, etc. Proper use of POST requests can ensure data security and accuracy. The following will introduce the correct usage of POST requests in PHP and provide specific code examples.
1. Basic principles of POST requests in PHP
In PHP, the data submitted through the POST method can be obtained by using the $_POST
global variable. The POST method sends form data to the server in POST mode, and the data is encapsulated in the message body of the HTTP request. On the server side, these data can be obtained through $_POST
, and then processed accordingly.
2. Correct usage of POST request in PHP
2.1 Form submission data
When using a form to submit data in the front-end page, you need to change the form's method# The ## attribute is set to
post to ensure that the data is sent to the server in POST mode. Receiving POST data in PHP can be obtained through the
$_POST global variable. The following is a simple example:
<form action="submit.php" method="post"> <input type="text" name="username"> <input type="password" name="password"> <button type="submit">提交</button> </form>
in submit.php
$_POSTGet the submitted data:
$username = $_POST['username']; $password = $_POST['password'];
mysqli_real_escape_string, or use prepared statements to perform database operations. The following is an example:
$username = mysqli_real_escape_string($conn, $_POST['username']);
filter_var to filter and verify data, such as verifying email format, verifying mobile phone number format, etc. The example is as follows:
$email = $_POST['email']; if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "邮箱格式不正确"; }
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = mysqli_real_escape_string($conn, $_POST['username']); $password = mysqli_real_escape_string($conn, $_POST['password']); // 数据验证 if (empty($username) || empty($password)) { echo "用户名和密码不能为空"; exit; } // 执行相应操作,例如数据库插入、用户认证等 echo "数据提交成功!"; } ?>
The above is the detailed content of Correct usage of POST request in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


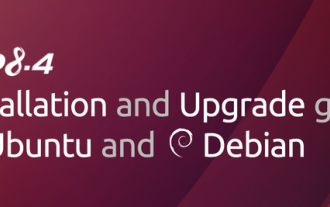
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
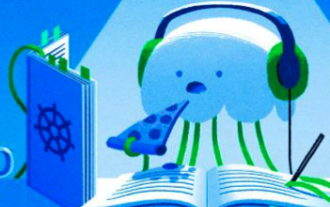
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
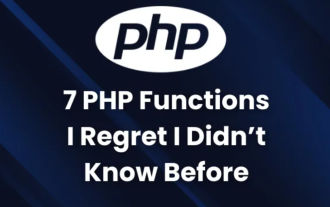
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
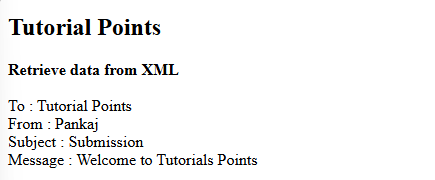
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
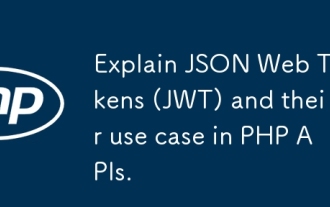
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
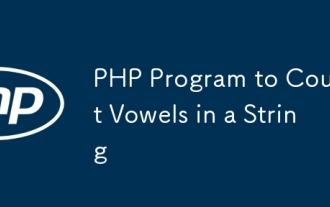
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
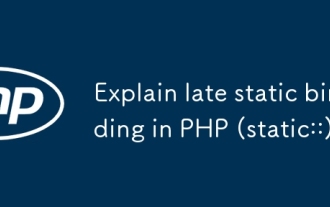
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
