PHP Tutorial: How to convert int type to string
PHP Tutorial: How to convert int type to string
In PHP, converting integer data to string is a common operation. This tutorial will introduce how to use PHP's built-in functions to convert the int type to a string, while providing specific code examples.
- Use forced type conversion:
In PHP, you can use forced type conversion to convert integer data into a string. This method is very simple. You only need to add (string) before the integer data to convert it into a string. The following is a simple sample code:
$intNum = 123; // 定义一个整型变量 $strNum = (string)$intNum; // 将整型数据转换为字符串 echo $strNum; // 输出转换后的字符串
In the above example, an integer variable $intNum is first defined, then cast is used to convert it to a string, and the result is assigned to $strNum . Finally, use the echo statement to output the converted string.
- Use PHP built-in function strval():
In addition to using forced type conversion, PHP also provides a built-in function strval() that can convert integer data to string. Using the strval() function is also very simple. You only need to pass integer data as a parameter to the function. The following is a sample code using the strval() function:
$intNum = 456; // 定义一个整型变量 $strNum = strval($intNum); // 使用strval()函数将整型数据转换为字符串 echo $strNum; // 输出转换后的字符串
In the above example, an integer variable $intNum is first defined, then the strval() function is called to convert it to a string, and the result is Assigned to $strNum. Finally, use the echo statement to output the converted string.
- Note:
When converting the int type to a string, you need to pay attention to some special cases, such as when the integer data is 0, convert it to a string will become an empty string later. Therefore, in actual development, corresponding processing needs to be made according to specific situations.
To sum up, this tutorial introduces how to use PHP's cast and strval() function to convert the int type to a string, and provides specific code examples. In daily development, choose the appropriate method for conversion according to needs to ensure the normal operation of the program.
The above is the detailed content of PHP Tutorial: How to convert int type to string. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


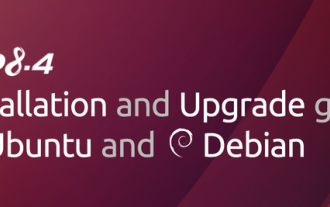
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
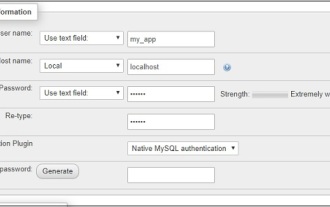
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
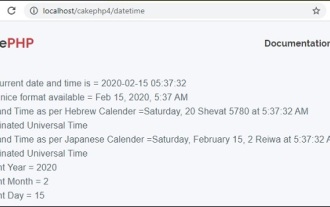
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
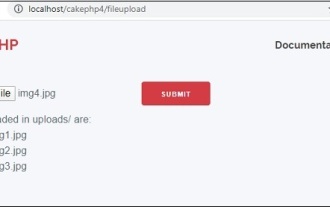
To work on file upload we are going to use the form helper. Here, is an example for file upload.
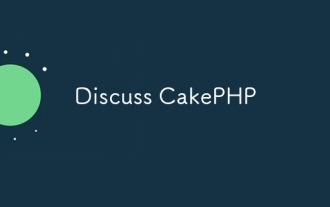
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
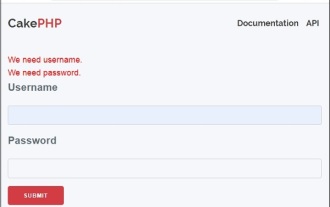
Validator can be created by adding the following two lines in the controller.
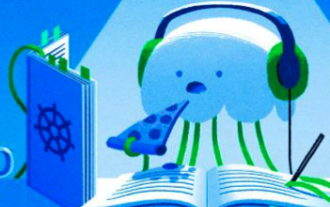
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
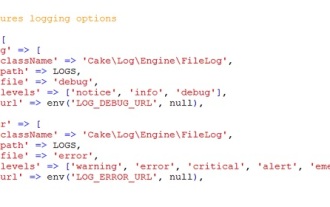
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
