Deep understanding of polymorphism features in PHP
Polymorphism is one of the important concepts in object-oriented programming, which allows different objects to use the same method name to perform different operations. In PHP, polymorphism is achieved through inheritance and implementing interfaces. This article will delve into the features of polymorphism in PHP and deepen your understanding through specific code examples.
What is polymorphism?
Polymorphism means that different objects have different reactions to the same message. In object-oriented programming, polymorphism allows us to define a general interface without having to consider specific implementation details. This improves the scalability and maintainability of your code.
How polymorphism is implemented in PHP
In PHP, polymorphism is usually implemented through inheritance and interfaces. Through inheritance, subclasses can override the methods of the parent class and implement different behaviors according to specific needs. Through interfaces, a class can define a set of methods, and the class that implements this interface needs to implement these methods to achieve polymorphism.
Sample code
Let us use a simple example to illustrate how polymorphism is implemented in PHP:
// 定义一个接口Shape interface Shape { public function calculateArea(); } // 定义一个矩形类Rectangle实现Shape接口 class Rectangle implements Shape { private $width; private $height; public function __construct($width, $height) { $this->width = $width; $this->height = $height; } public function calculateArea() { return $this->width * $this->height; } } // 定义一个圆形类Circle实现Shape接口 class Circle implements Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function calculateArea() { return 3.14 * pow($this->radius, 2); } } // 使用多态性调用不同的calculateArea方法 function printArea(Shape $shape) { echo "The area is: " . $shape->calculateArea() . " "; } // 创建矩形对象并打印面积 $rectangle = new Rectangle(5, 10); printArea($rectangle); // 创建圆形对象并打印面积 $circle = new Circle(5); printArea($circle);
In the above code, we define an interface Shape, and implements two different shape classes Rectangle and Circle, both of which implement the calculateArea()
method of the Shape interface. Through the printArea()
function, we can call the calculateArea()
method of different shape objects to calculate the area, and there is no need to care about the specific type of shape class.
Summary
Through the above examples, we can see how to achieve polymorphism through interfaces and inheritance in PHP, thereby achieving code flexibility and scalability. Polymorphism is a very important concept in object-oriented programming, which can help us better organize and manage code, and improve code reusability and maintainability. I hope this article can help readers gain a deeper understanding of the features of polymorphism in PHP.
The above is the detailed content of Deep understanding of polymorphism features in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


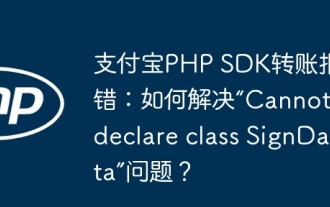
Alipay PHP...
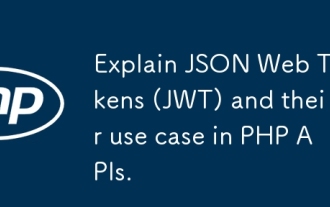
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
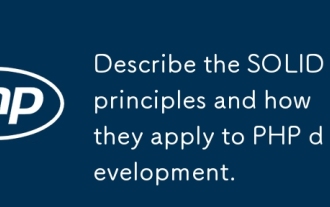
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
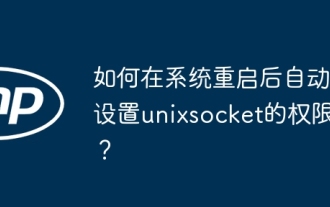
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
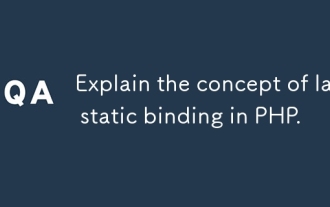
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
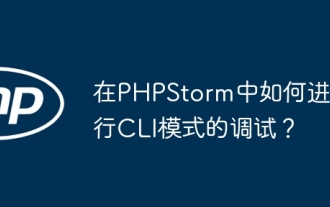
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
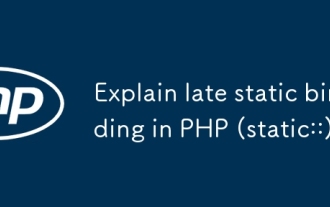
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
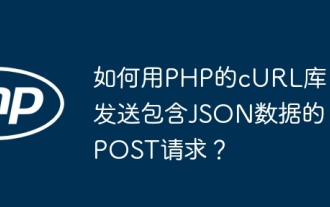
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
