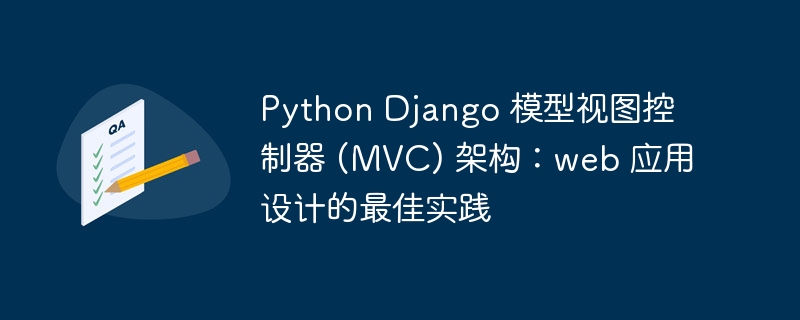
Model
-
Responsibilities: Represents the application's data, including database tables, forms, and model managers.
-
Best Practices:
- Use Django's model definition to create a database table.
- Use the ModelFORM class to handle form validation.
- Use the model manager to perform complex queries and operations.
view
-
Responsibilities: Handle user requests and return appropriate responses, i.e. templates or redirects.
-
Best Practices:
- Use Class-Based View to organize code and simplify reuse.
- Use the template system to generate html responses.
- Use redirection to change the state of the application.
Controller
-
Responsibilities: Connect models and views, handle user interactions and update models.
-
Best Practices:
- Use the URL Configurator (URLConfig) to map URL patterns to views.
- Use middleware to handle cross-application request operations.
- Use view functions or view classes to handle specific requests.
Other Best Practices
-
Using ORM: DjanGo provides a powerful object-relational mapper (ORM) that enables you to interact with the database in an object-oriented way .
-
Using Template Inheritance: Template inheritance allows you to create reusable template blocks, thus simplifying your code and reducing duplication.
-
Use cache: Cache can improve the performance of the application and reduce the number of database calls.
-
Perform Unit Testing: Unit Testing is critical to ensure the correctness and robustness of your application.
-
Follow coding standards: Use consistent coding style and naming conventions to improve code readability and maintainability.
Advantage
mvc Architecture provides many advantages, including:
-
Separation of concerns: MVC separates the business logic, presentation layer and control layer of the application, thereby improving maintainability.
-
Extensibility: As applications become more complex, the MVC architecture allows new functionality to be easily added or existing functionality to be extended.
-
Testability: MVC makes it easier to unit test individual components of an application to ensure its reliability.
-
Code Reuse: MVC promotes code reuse, saving development time and improving code quality.
in conclusion
By following the best practices of python Django MVC architecture, you can design WEB applications that are robust, maintainable, and scalable. By separating the model, view, and controller layers, using the appropriate tools and techniques, and following coding conventions, you'll create high-quality applications that satisfy your users and meet your business needs.
The above is the detailed content of Python Django Model View Controller (MVC) Architecture: Best Practices for Web Application Design. For more information, please follow other related articles on the PHP Chinese website!