Tips for converting strings to floating point numbers in PHP
PHP is a widely used server-side scripting language for creating dynamic websites and interactive web applications. In PHP, converting a string to a floating point number is a common operation. This article will introduce some techniques for converting strings to floating point numbers in PHP and provide specific code examples.
1. Use (float) forced type conversion
In PHP, you can use forced type conversion to convert a string into a floating point number. The specific code example is as follows:
$str = "3.14"; $floatNum = (float)$str; echo $floatNum; // 输出 3.14
In this example, we force the string "3.14" to a floating point number and then assign it to the variable $floatNum. Finally, the value of $floatNum is output through the echo statement.
2. Use (double) forced type conversion
In addition to (float), PHP also provides (double) for converting strings to floating point numbers. The example is as follows:
$str = "5.67"; $floatNum = (double)$str; echo $floatNum; // 输出 5.67
In this example, we also force the string "5.67" to a floating point number, assign it to the variable $floatNum, and finally output the value of $floatNum.
3. Use the (floatval) function
In addition to forced type conversion, PHP also provides a function floatval() for converting strings to floating point numbers. The specific example is as follows:
$str = "9.99"; $floatNum = floatval($str); echo $floatNum; // 输出 9.99
In this example, we use the floatval() function to convert the string "9.99" into a floating point number, assign it to the variable $floatNum, and finally output the value of $floatNum.
4. Use the (doubleval) function
Similarly, PHP also provides a function doubleval() for converting strings to floating point numbers. The example is as follows:
$str = "2.34"; $floatNum = doubleval($str); echo $floatNum; // 输出 2.34
In this example, we use the doubleval() function to convert the string "2.34" into a floating point number, assign it to the variable $floatNum, and finally output the value of $floatNum.
Summary:
This article introduces several common methods to convert strings to floating point numbers in PHP, including using forced type conversion, floatval() function and doubleval() function. These methods are all efficient at converting strings to floating point numbers and are very simple and easy to use. In actual development, you can choose the appropriate method to convert strings to floating point numbers according to specific needs.
The above is the detailed content of Tips for converting strings to floating point numbers in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
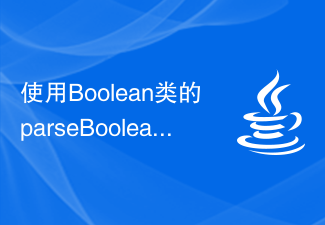
How to convert a string into a Boolean value using the parseBoolean() method of the Boolean class. In Java programming, you often encounter situations where you need to convert a string into a Boolean value. The Boolean class in Java provides a very convenient method - parseBoolean(), which can convert strings into corresponding Boolean values. This article will introduce the use of this method in detail and provide corresponding code examples. First, we need to understand the parseBoolean() method
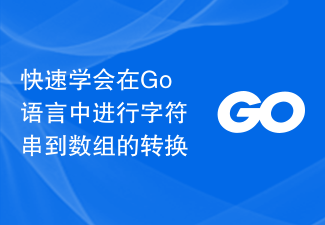
Quickly learn to convert strings to arrays in Go language. Conversion between strings and arrays is a common operation in Go language. Especially when processing data, you often encounter the need to convert strings into arrays. Condition. This article will introduce how to quickly learn to convert strings to arrays in Go language, so that you can easily deal with similar problems. In Go language, we can use the Split function provided by the strings package to split a string into an array according to the specified delimiter. The following is a
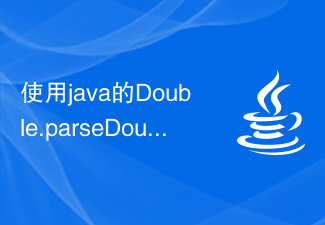
Convert a string to a double-precision floating point number using Java's Double.parseDouble() function In Java programming, we often need to convert a string to a numeric type. For double-precision floating-point numbers, Java provides a very convenient method, the Double.parseDouble() function. This article will introduce the usage of this function and attach some sample code to help readers better understand and use this function. Double.parseDouble() function is
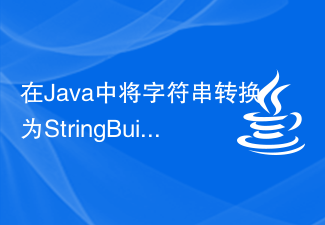
The append() method of StringBuilder class accepts a String value and adds it to the current object. Convert string value to StringBuilder object - Get string value. Append using the append() method to get the string into the StringBuilder. Example In the following Java program, we are converting an array of strings into a single StringBuilder object. Real-time demonstration publicclassStringToStringBuilder{ publicstaticvoidmain(Stringargs[]){&a
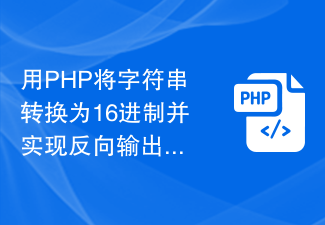
Title: Use PHP to convert strings to hexadecimal and achieve reverse output. In daily development, we sometimes need to convert strings to hexadecimal representation for data transmission or encryption. This article will introduce how to use PHP to convert a string into hexadecimal and realize the reverse output function. First, we need to write a PHP function to convert a string to hexadecimal. The following is an example code: functionstringToHex($string)
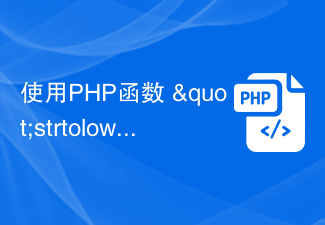
Convert a string to lowercase using PHP function "strtolower" In PHP, there are many functions that can be used to convert the case of a string. One of the very commonly used functions is strtolower(). This function converts all characters in a string to lowercase. Here is a simple example code showing how to use the strtolower() function to convert a string to lowercase: <?php//original string $string="
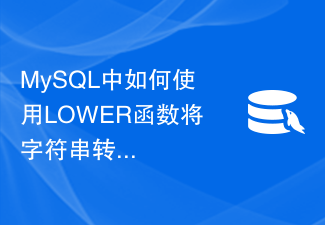
How to use the LOWER function in MySQL to convert a string to lowercase. In the MySQL database, we often encounter situations where we need to convert a string to lowercase, such as converting the user name entered by the user to lowercase for verification, or performing verification on a certain column. Case-insensitive search. At this time, you can use the MySQL built-in function LOWER to complete this task. The LOWER function is a string function that converts uppercase letters in a string to lowercase. Use the LOWER function to easily convert words
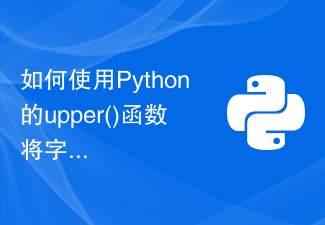
How to convert a string to uppercase using Python's upper() function, specific code example required Python is a simple and easy-to-learn programming language that provides many built-in functions to handle strings. One of the commonly used functions is the upper() function, which converts all letters in a string to uppercase. This article will introduce in detail how to use Python's upper() function and provide corresponding code examples. First, let us understand the usage of upper() function. up
