


In-depth discussion of the application scenarios of Go language functions
Go language is a modern, concise and efficient programming language developed by Google and widely used in the open source community. In the Go language, a function is a basic code block that is independent and can be called repeatedly. It plays an important role in achieving modularization and structuring of the code. This article will deeply explore the application scenarios of Go language functions and illustrate them with specific code examples.
1. Basic concepts and syntax of functions
In Go language, the basic format of functions is as follows:
func 函数名(参数列表) 返回值列表 { // 函数体 return 返回值 }
Among them, func
is a function Keyword, followed by the function name and parameter list, enclosed in parentheses. The parameter list can contain zero or more parameters, each consisting of a parameter name and a parameter type. The return value list can also contain zero or more return values, separated by commas. The function body is enclosed in curly brackets and contains the specific implementation logic of the function. Finally, use the return
keyword to return the return value of the function.
2. Application scenarios of functions
1. Encapsulating repeated logic
Function can encapsulate repeated code logic to improve code reusability and maintainability. For example, the following example demonstrates a function calculateSum
, which is used to calculate the sum of two integers:
func calculateSum(a, b int) int { return a + b }
By defining this function, calculateSum# can be called multiple times in the program ##To calculate the sum of two integers, avoid writing the same calculation logic repeatedly.
goroutine, and functions play an important role in concurrent programming. You can encapsulate a time-consuming operation into a function, and then start a goroutine to execute the function through the
go keyword. The following is an example:
func doSomething() { // 耗时操作 } func main() { go doSomething() // 其他逻辑 }
doSomething function contains a time-consuming operation that can be executed in the background through goroutine, while the main program can continue to execute other logic.
func process(callback func(int)) { // 处理逻辑 result := 100 callback(result) } func main() { process(func(result int) { fmt.Println("处理结果:", result) }) }
process function accepts a parameter of function type
callback, and returns through the callback function after processing the logic process result.
The above is the detailed content of In-depth discussion of the application scenarios of Go language functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
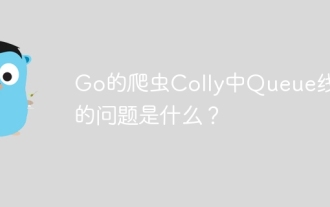
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
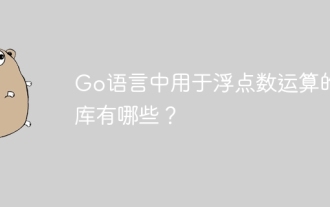
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
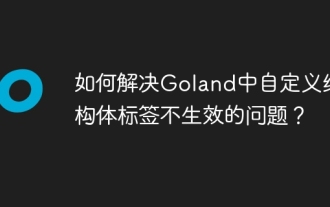
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
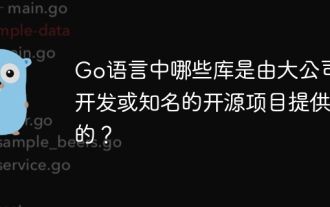
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
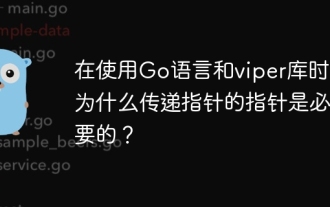
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
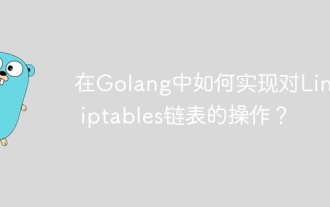
Using Golang to implement Linux...
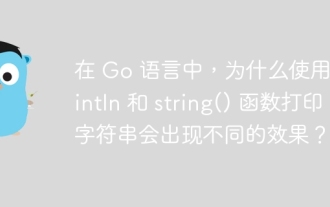
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
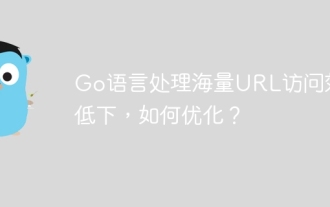
Performance optimization strategy for Go language massive URL access This article proposes a performance optimization solution for the problem of using Go language to process massive URL access. Existing programs from CSV...
