In-depth analysis of the meaning of PHP interface design
Title: Exploring the importance and practical application of PHP interface design
With the continuous development of Web development technology, interface design has become more and more important in modern software development important. In PHP development, well-designed interfaces can help achieve modular, easy-to-maintain, and highly scalable code. This article will delve into what PHP interface design means and how to put it into practice using specific code examples.
What is interface design?
In object-oriented programming, an interface is an abstract concept that defines the behavior and protocols that a class or object should follow. An interface defines the methods that a class or object should provide, but it does not implement the specific logic of these methods. Instead, the class that implements the interface implements these methods. Through interfaces, loose coupling between objects can be achieved, improving code reusability and maintainability.
Definition and implementation of PHP interface
In PHP, the interface is defined using the keyword interface
. Let us illustrate the definition and implementation of the interface through an example:
<?php // 定义一个接口 interface Animal { public function sound(); } // 实现接口 class Cat implements Animal { public function sound() { echo "喵喵喵"; } } class Dog implements Animal { public function sound() { echo "汪汪汪"; } } // 使用接口 $cat = new Cat(); $cat->sound(); // 输出:喵喵喵 $dog = new Dog(); $dog->sound(); // 输出:汪汪汪 ?>
In the above example, we defined an Animal
interface and implemented two classes Cat
and Dog
to implement this interface. Through the interface, we stipulate that the Animal
interface must provide the sound()
method, and different concrete classes can implement this method according to their own logic.
Application scenarios of PHP interface
- Achieve code reuse: Through the interface, you can define methods that are jointly implemented by multiple classes, reducing repeated coding and improving code Reusability.
- Achieve polymorphism: Since PHP is a weakly typed language, polymorphism can be achieved between different objects through interfaces, improving the flexibility of the code.
- Implementing framework extension: In PHP framework development, interfaces are often used to define plug-in specifications and achieve flexible expansion of the framework.
- Integrating third-party services: When you need to integrate third-party services or APIs, you can define interfaces to standardize the communication between the application and the third-party service.
Best Practices in PHP Interface Design
- Single Responsibility Principle: An interface should only contain one abstract method, follow the single responsibility principle, and improve Code maintainability.
- Clear naming: The naming of the interface should have clear semantics and accurately describe the role of the interface.
- Avoid over-design: The interface design should meet actual needs, do not over-design, and avoid interface redundancy and cumbersomeness.
- Reasonable splitting of interfaces: Reasonably split the interface according to business logic to improve the scalability and flexibility of the interface.
Conclusion
In PHP development, reasonable interface design can improve the maintainability, scalability and flexibility of the code, and is one of the important means to achieve object-oriented programming. . Through the introduction of this article, I believe that readers will have a deeper understanding of the meaning of PHP interface design, and be able to flexibly apply the ideas of interface design in actual projects to write high-quality PHP code.
The above is the detailed content of In-depth analysis of the meaning of PHP interface design. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
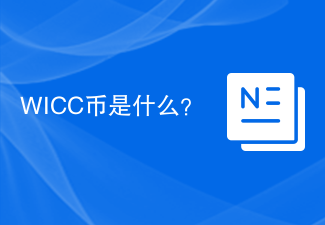
What is WICC Coin? WICC Coin is the abbreviation of WaykiChainCoin, which is a digital currency based on blockchain technology. As an efficient, scalable and secure public chain, WaykiChain is committed to providing enterprises and developers with complete blockchain infrastructure and innovative tools. As the core token of the WaykiChain ecosystem, WICC Coin plays an important role on the platform. Features of WICC currency 1. Safe and reliable: WaykiChain adopts the DPoS consensus algorithm and has a reliable distributed locking mechanism and consensus mechanism to ensure a high degree of network security. 2. Efficient and scalable: WaykiChain has millisecond-level transaction confirmation speeds, can handle thousands of transactions per second, and
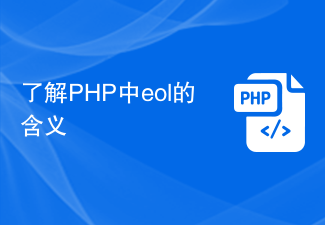
In-depth understanding of the meaning and code examples of eol in PHP In PHP programming, eol is a common term that represents "EndOfLine", which is the end of the line. In different operating systems, the end of a line may be expressed differently, which leads to the concept of eol. In Windows systems, the end of a line is composed of carriage return () and line feed (), that is, ""; while in Unix/Linux systems, the end of a line is only represented by line feed (), that is, "". Such differences may result in different operating systems
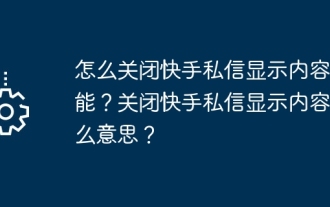
As the leading short video platform in China, Kuaishou has a large number of users, and the private messaging function is an important channel for interaction between users. However, some users may find the ability to display content in private messages bothering them and would like to be able to selectively turn this feature off. 1. How to turn off the content display function of Kuaishou private messages? 1. Open the Kuaishou app and log in to your personal account. 2. Enter the Kuaishou main interface and click the "My" button in the lower right corner to enter the personal center. 3. On the personal center page, click the avatar to enter personal settings. 4. On the personal settings page, find the "Privacy Settings" option and click to enter. 5. On the privacy settings page, find the "Display content in private messages" option and click to enter. 6. On the private message display content setting page, turn off the "private message display content" function

The meaning and specific usage of MySQL host name MySQL is a popular open source relational database management system that is widely used in various web applications. In MySQL, hostname is an important concept, which is used to specify the name of the host connected to the database server. In this article, we will explain in detail what the MySQL hostname means and how to use it in actual development. The meaning of MySQL host name: In MySQL, the host name is used to specify which connections are allowed to
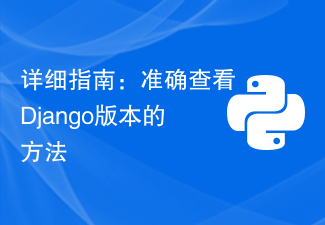
For an in-depth analysis of how to accurately view the Django version, specific code examples are required. Introduction: As a popular Python Web framework, Django often requires version management and upgrades. However, sometimes it may be difficult to check the Django version number in the project, especially when the project has entered the production environment, or uses a large number of custom extensions and partial modules. This article will introduce in detail how to accurately check the version of the Django framework, and provide some code examples to help developers better manage
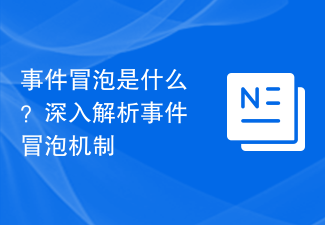
What is event bubbling? In-depth analysis of the event bubbling mechanism Event bubbling is an important concept in web development, which defines the way events are delivered on the page. When an event on an element is triggered, the event will be transmitted starting from the innermost element and passed outwards until it is passed to the outermost element. This delivery method is like bubbles bubbling in water, so it is called event bubbling. In this article, we will analyze the event bubbling mechanism in depth. The principle of event bubbling can be understood through a simple example. Suppose we have an H
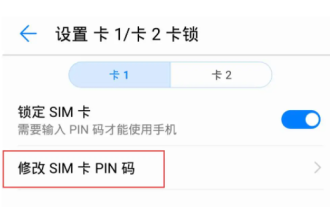
The pin code refers to the personal identification password of the mobile phone's SIM card. Parsing the 1pin code (pin1) is a telecommunications noun, and its full name is PersonalIdentificationNumber. It refers to the personal identification code of the mobile phone SIM card. The mobile phone PIN code is a security measure to protect the mobile phone SIM card to prevent others from using the mobile phone SIM card illegally. If the PIN code is enabled, a 4-8 digit pin code must be filled in every time the phone is turned on. When the pin code is invalid for more than three times, the card will be automatically locked for protection. To unlock, you need to use the PUK code to call the operator's customer service number. Supplement: How to unlock pin code 1. Open settings and click other settings on the settings page. 2Click Devices & Privacy on the Other Settings page. 3 under construction
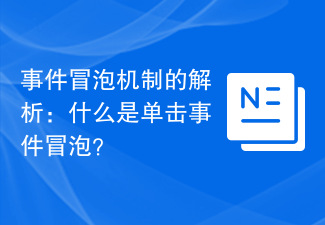
What is click event bubbling? In-depth analysis of the event bubbling mechanism requires specific code examples. Event bubbling (Event Bubbling) means that in the DOM tree structure, when an element triggers an event, the event will be passed along the DOM tree from the child elements to the root element. This process is like bubbles bubbling, so it is called event bubbling. Event bubbling is a mechanism of the DOM event model, included in documents such as HTML, XML, and SVG. This mechanism allows event handlers registered on the parent element to receive
