


Achieve more efficient Go language programming through function transformation
Achieve more efficient Go language programming through function transformation
As a fast, concise and efficient programming language, Go language is widely used in development in various fields middle. In Go language programming, functions are a very important component and are highly flexible and functional. More efficient programming can be achieved through the transformation of functions. This article will introduce how to achieve more efficient Go language programming through function transformation and provide specific code examples.
1. Optimization of functions
- Reduce function parameters
When writing a function, try to reduce the number of parameters of the function as much as possible, which can effectively simplify the function Call procedures to improve code readability and maintainability. If a function has too many parameters, you can consider encapsulating the parameters into a structure or using function closures to reduce the complexity of parameter passing.
Sample code:
// 参数过多的函数 func processData(a int, b string, c float64, d bool) { // 执行逻辑 } // 优化后的函数 type Data struct { A int B string C float64 D bool } func processData(data Data) { // 执行逻辑 }
- Using function closure
Function closure is a very powerful feature in the Go language, which can be used inside a function Define a function and have access to the variables of the external function. More flexible and efficient programming can be achieved through function closures, which is especially suitable for applications in scenarios where function logic needs to be dynamically generated.
Sample code:
func add(x int) func(int) int { return func(y int) int { return x + y } } func main() { addFunc := add(10) result := addFunc(5) // 结果为15 }
2. Concurrent processing of functions
In the Go language, concurrent programming can be easily achieved using goroutine and channel. Through concurrent processing of functions, the performance of multi-core CPUs can be fully utilized and the running efficiency of the program can be improved.
- Using goroutine
Goroutine is a lightweight thread in the Go language that can execute functions or methods concurrently to achieve concurrent processing. Time-consuming operations can be performed through goroutine to avoid blocking the execution flow of the main program.
Sample code:
func doTask() { // 执行耗时操作 } func main() { go doTask() // 使用goroutine并发执行任务 // 主程序的其他逻辑 }
- Using channel
Channel is an important mechanism for communication and data transfer between goroutines. Through channels, data can be safely shared between different goroutines to avoid race conditions and data competition issues.
Sample code:
func sendData(ch chan string) { ch <- "data" } func main() { ch := make(chan string) go sendData(ch) data := <-ch // 从channel接收数据 }
3. Function error handling
In the Go language, the robustness and reliability of the code can be improved through function error handling. Using the error handling mechanism in the standard library can well handle errors that may occur during function execution and avoid program crashes or exceptions.
- Use error type
Return an error type value in the function to indicate possible error conditions during function execution. Errors need to be determined when calling functions and handled appropriately.
Sample code:
func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", result) } }
- Use the defer statement
The defer statement can ensure that the operation will be performed during the execution of the function, usually used for release Resource or processing error. Using defer statements in functions can better manage resources and avoid code redundancy.
Sample code:
func readData() error { file, err := os.Open("data.txt") if err != nil { return err } defer file.Close() // 确保文件关闭 // 读取文件操作 return nil }
4. Function tuning
In Go language programming, the performance and efficiency of the program can be improved by tuning functions. You can use performance analysis tools to evaluate the performance of functions and perform corresponding optimization.
- Using performance analysis
The Go language provides a series of performance analysis tools, such as pprof and trace, which can help developers analyze bottlenecks and performance issues in the program. . Use performance analysis tools to find functions that affect program performance and perform targeted optimizations.
Sample code:
import _ "net/http/pprof" func main() { // 启动性能分析服务 go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }() }
- Use performance optimization techniques
When optimizing functions, you can use some common performance optimization techniques, such as reducing Memory allocation, avoiding unnecessary type conversions, caching results, etc. These techniques can effectively improve the efficiency and performance of the program.
Sample code:
func sum(nums []int) int { var total int for _, num := range nums { total += num } return total }
Summary:
Through the transformation of functions, more efficient Go language programming can be achieved and the readability, robustness and performance of the code can be improved. Developers should focus on function design and optimization when writing Go language programs, and make full use of function features and concurrency features to achieve a more elegant and efficient way of writing code. I hope the introduction and examples in this article will be helpful to you, allowing you to go further on the road of Go language programming and write better programs!
The above is the detailed content of Achieve more efficient Go language programming through function transformation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
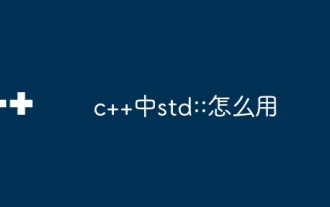
std is the namespace in C++ that contains components of the standard library. In order to use std, use the "using namespace std;" statement. Using symbols directly from the std namespace can simplify your code, but is recommended only when needed to avoid namespace pollution.
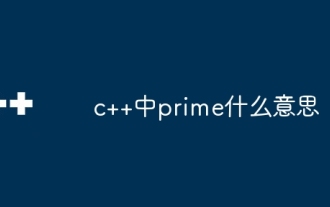
prime is a keyword in C++, indicating the prime number type, which can only be divided by 1 and itself. It is used as a Boolean type to indicate whether the given value is a prime number. If it is a prime number, it is true, otherwise it is false.
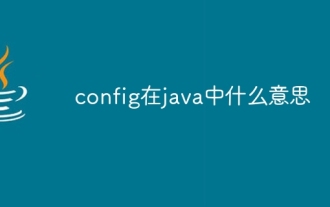
Config represents configuration information in Java and is used to adjust application behavior. It is usually stored in external files or databases and can be managed through Java Properties, PropertyResourceBundle, Java Configuration Framework or third-party libraries. Its benefits include decoupling and flexibility. , environmental awareness, manageability, scalability.

The fabs() function is a mathematical function in C++ that calculates the absolute value of a floating point number, removes the negative sign and returns a positive value. It accepts a floating point parameter and returns an absolute value of type double. For example, fabs(-5.5) returns 5.5. This function works with floating point numbers, whose accuracy is affected by the underlying hardware.
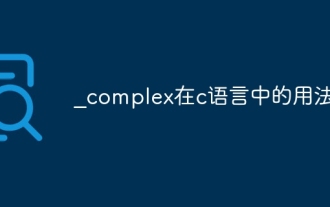
The complex type is used to represent complex numbers in C language, including real and imaginary parts. Its initialization form is complex_number = 3.14 + 2.71i, the real part can be accessed through creal(complex_number), and the imaginary part can be accessed through cimag(complex_number). This type supports common mathematical operations such as addition, subtraction, multiplication, division, and modulo. In addition, a set of functions for working with complex numbers is provided, such as cpow, csqrt, cexp, and csin.
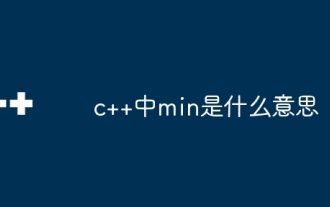
The min function in C++ returns the minimum of multiple values. The syntax is: min(a, b), where a and b are the values to be compared. You can also specify a comparison function to support types that do not support the < operator. C++20 introduced the std::clamp function, which handles the minimum of three or more values.
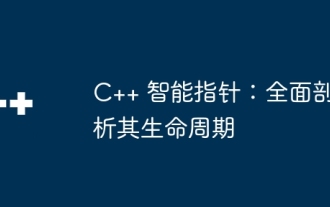
Life cycle of C++ smart pointers: Creation: Smart pointers are created when memory is allocated. Ownership transfer: Transfer ownership through a move operation. Release: Memory is released when a smart pointer goes out of scope or is explicitly released. Object destruction: When the pointed object is destroyed, the smart pointer becomes an invalid pointer.
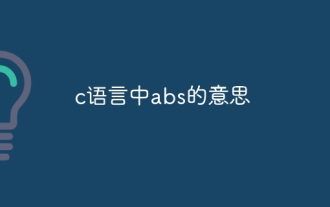
The abs() function in c language is used to calculate the absolute value of an integer or floating point number, i.e. its distance from zero, which is always a non-negative number. It takes a number argument and returns the absolute value of that number.
