


In-depth understanding of function refactoring techniques in Go language
In the development of Go language programs, function reconstruction skills are a very important part. By optimizing and refactoring functions, you can not only improve code quality and maintainability, but also improve program performance and readability. This article will delve into the function reconstruction techniques in the Go language, combined with specific code examples, to help readers better understand and apply these techniques.
1. Code example 1: Extract duplicate code fragments
In actual development, we often encounter reused code fragments. At this time, we can consider extracting the duplicate code as an independent code fragment. functions to improve code reusability and maintainability. For example, here is a simple example:
func add(x, y int) int { return x + y } func subtract(x, y int) int { return x - y } func multiply(x, y int) int { return x * y } func divide(x, y int) int { return x / y }
In the above example, add
, subtract
, multiply
, and divide
The function has a large number of repeated code fragments, and the calculation logic can be extracted as a general function:
func calculate(x, y int, operation func(int, int) int) int { return operation(x, y) } func add(x, y int) int { return calculate(x, y, func(a, b int) int { return a + b }) } func subtract(x, y int) int { return calculate(x, y, func(a, b int) int { return a - b }) } func multiply(x, y int) int { return calculate(x, y, func(a, b int) int { return a * b }) } func divide(x, y int) int { return calculate(x, y, func(a, b int) int { return a / b }) }
By extracting the repeated calculation logic into the general calculate
function, It can avoid code duplication and improve the maintainability of the code.
2. Code example 2: Optimizing function parameters
In the Go language, function parameters should usually be as concise and clear as possible to avoid excessive parameter passing and nesting. When a function has too many parameters or is too complex, you can consider optimizing the parameter design of the function through structures or function option parameters. The following is an example:
type User struct { ID int Name string } func getUserInfo(userID int, userName string) { // do something } func getUser(user User) { // do something } func main() { // 传递单个用户ID和用户名作为参数 getUserInfo(1, "Alice") // 传递User结构体作为参数 user := User{ID: 1, Name: "Alice"} getUser(user) }
In the example, the getUserInfo
function needs to pass two parameters, the user ID and the user name respectively, while the getUser
function passes a parameter containing User information structure to simplify parameter passing. By using structures as parameters, not only can function parameters be simplified, but the code can also be made clearer and easier to understand.
3. Code example 3: Using function closure
Function closure is a powerful function reconstruction technique, which is often used in Go language to implement delayed execution and state retention of functions. and other functions. The following is an example of using function closures:
func counter() func() int { num := 0 return func() int { num++ return num } } func main() { count := counter() fmt.Println(count()) // 输出: 1 fmt.Println(count()) // 输出: 2 fmt.Println(count()) // 输出: 3 }
In the example, the counter
function returns an internal anonymous function that can be accessed and modified through closuresnum
Variable. Each time the count
function is called, num
will increment and return the incremented value, realizing a simple and effective counting function.
Through the above examples, readers can better understand the function reconstruction techniques in Go language, including extracting duplicate code, optimizing function parameters, and using function closures. In actual development, reasonable application of these techniques can improve code quality and maintainability, and bring better development experience and effects. I hope this article is helpful to readers, and you are welcome to continue to learn more advanced features and techniques of the Go language.
The above is the detailed content of In-depth understanding of function refactoring techniques in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
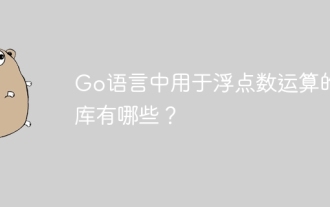
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
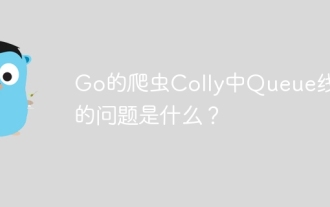
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
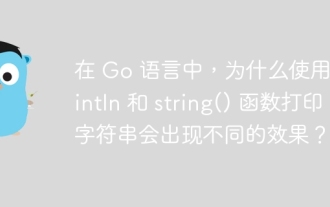
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
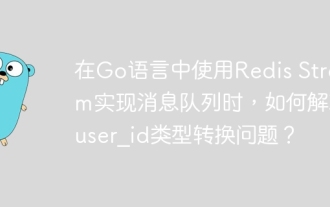
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
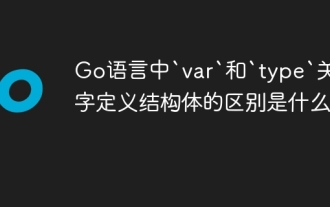
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
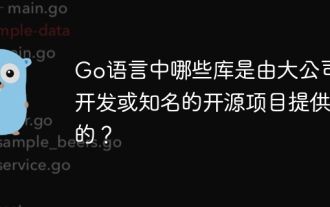
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
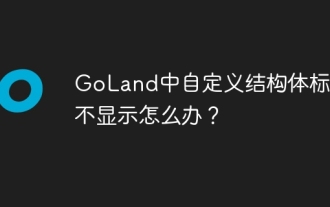
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
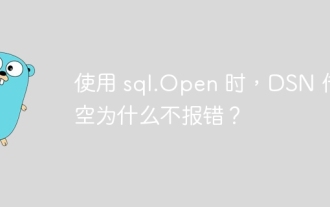
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
