


PHP Programming Guidelines: Valid validation strings are limited to numbers and letters
Programming discipline is crucial to ensure code quality and maintainability, especially when developing PHP applications. One of the common requirements is efficient validation of input strings to ensure that they contain only numeric and alphabetic characters. This article will introduce how to write code in PHP to achieve this requirement while following programming conventions.
Overview of Programming Standards
In PHP programming, following certain programming standards can make the code easier to read and maintain, while also helping to reduce errors and improve code performance. The following are some suggestions for programming standards:
- Use meaningful variable names and function names to improve code readability.
- Comment the code to explain the function and logic of the code so that others can understand it.
- Use consistent indentation and formatting styles to maintain code uniformity.
- Avoid using global variables and reduce the scope of variables as much as possible.
- Exception handling and error handling must be improved to ensure the robustness of the code.
- Effectively verify input to prevent injection attacks and malicious input.
Code example: Verify that the string contains only numbers and letters
The following is a sample code to verify that the input string contains only numbers and letters, and return the verification result .
<?php function validateString($input) { if (preg_match('/^[a-zA-Z0-9]+$/', $input)) { return true; } else { return false; } } $input1 = "Hello123"; $input2 = "123456"; $input3 = "Hello123!"; if (validateString($input1)) { echo "输入字符串 $input1 仅包含数字和字母 "; } else { echo "输入字符串 $input1 包含非法字符 "; } if (validateString($input2)) { echo "输入字符串 $input2 仅包含数字和字母 "; } else { echo "输入字符串 $input2 包含非法字符 "; } if (validateString($input3)) { echo "输入字符串 $input3 仅包含数字和字母 "; } else { echo "输入字符串 $input3 包含非法字符 "; } ?>
In the above code, we define a function validateString
, using the regular expression '/^[a-zA-Z0-9] $/'
To check if the input string contains only numeric and alphabetic characters. Then, we verify three different inputs and output the verification results.
Following the above programming conventions, we succinctly wrote an effective verification function and tested it. In this way, you can ensure that the input data is as expected and safe, while improving the readability and maintainability of the code.
In general, programming specifications play a vital role in PHP programming, and effectively validating that a string contains only numeric and alphabetic characters is one of the specific practices. I hope this article can inspire you and prompt you to write more standardized and secure PHP code.
The above is the detailed content of PHP Programming Guidelines: Valid validation strings are limited to numbers and letters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


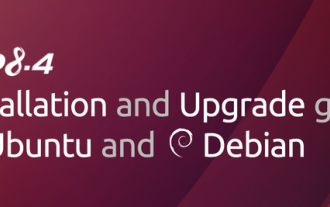
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
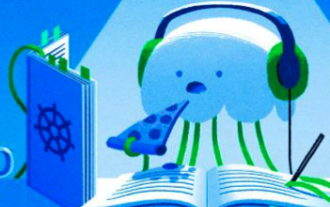
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
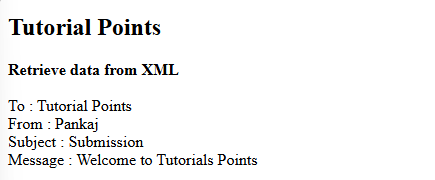
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
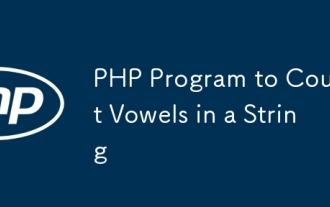
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
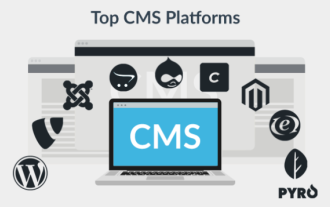
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
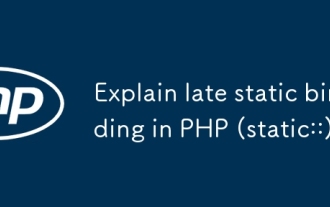
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
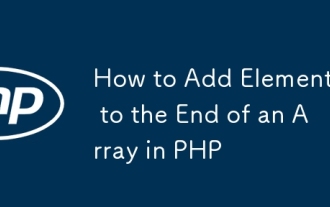
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
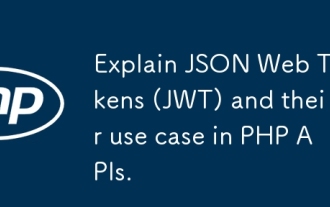
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
