Explore advanced usage of functions in Go language
Title: Exploring the advanced usage of functions in Go language
In Go language, function is a very important basic concept. It is one of the basic building blocks in the program. one. In addition to regular function definitions and calls, Go language functions also have many advanced uses, such as closures, anonymous functions, functions as parameters, functions as return values, etc. This article dives into the use of these advanced functions and provides specific code examples.
1. Closure
Closure refers to the situation where a function value can refer to variables outside its function body. Through closures, we can access variables in the outer function scope from within the function. The following is a simple closure example:
package main import "fmt" func main() { add := func(x, y int) int { return x + y } result := add(3, 5) fmt.Println(result) // 输出:8 }
2. Anonymous function
Anonymous function refers to a function that is defined only when used, usually used for simple logic processing. The following is an example of an anonymous function:
package main import "fmt" func main() { add := func(x, y int) int { return x + y } result := add(3, 5) fmt.Println(result) // 输出:8 }
3. Function as parameter
In the Go language, functions can be passed as parameters to other functions, which can achieve more advanced functions. The following is an example of a function as a parameter:
package main import "fmt" func calculate(x, y int, operation func(int, int) int) int { return operation(x, y) } func add(x, y int) int { return x + y } func subtract(x, y int) int { return x - y } func main() { result1 := calculate(3, 5, add) fmt.Println(result1) // 输出:8 result2 := calculate(10, 3, subtract) fmt.Println(result2) // 输出:7 }
4. Function as a return value
In addition to being passed as parameters, functions can also be returned as return values. This method is very useful in certain scenarios. The following is an example of a function as a return value:
package main import "fmt" func getCalculator(operation string) func(int, int) int { switch operation { case "add": return func(x, y int) int { return x + y } case "subtract": return func(x, y int) int { return x - y } default: return nil } } func main() { calculator := getCalculator("add") result := calculator(3, 5) fmt.Println(result) // 输出:8 }
Through the above example, we can see the advanced usage of Go language functions, including closures, anonymous functions, functions as parameters and functions as return values. These features can help us design and implement program logic more flexibly. Hope this article is helpful to you.
The above is the detailed content of Explore advanced usage of functions in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
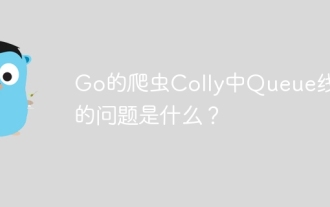
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
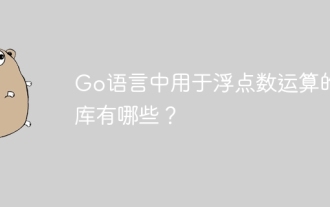
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
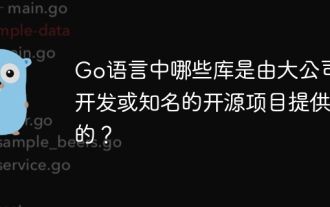
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
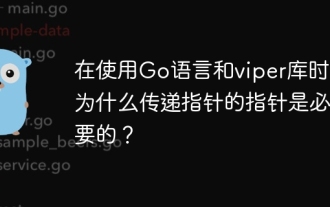
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
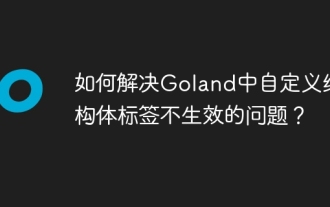
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
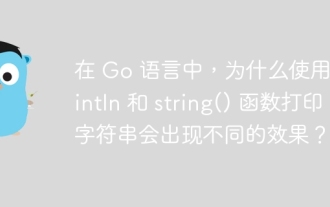
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
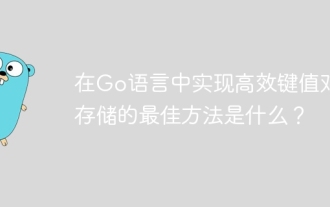
The correct way to implement efficient key-value pair storage in Go language How to achieve the best performance when developing key-value pair memory similar to Redis in Go language...
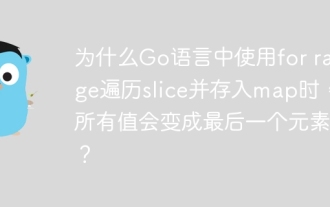
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
