


Practical applications and challenges of Go language in network programming
Go language, as a fast and efficient programming language, has received widespread attention in the field of network development due to its concurrency features and standard libraries suitable for network programming. This article will explore the practical applications and challenges of Go language in network programming, and illustrate it with specific code examples.
First of all, let us understand the main advantages of Go language in network programming. A major feature of the Go language is that it natively supports concurrent programming and has lightweight goroutines and channels, making it very simple and efficient to write concurrent network applications. In addition, the Go language standard library provides a wealth of network-related packages, such as net, http, etc., which can help developers quickly build various network applications.
Next, let us demonstrate the application of Go language in network programming through a simple example of TCP server and client. First is the code example of the TCP server:
package main import ( "fmt" "net" ) func main() { listener, err := net.Listen("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Error listening:", err.Error()) return } defer listener.Close() fmt.Println("Server started. Listening on 127.0.0.1:8080") for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting: ", err.Error()) return } go handleConnection(conn) } } func handleConnection(conn net.Conn) { defer conn.Close() buffer := make([]byte, 1024) for { _, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) return } fmt.Println("Received message:", string(buffer)) _, err = conn.Write([]byte("Message received")) if err != nil { fmt.Println("Error writing:", err.Error()) return } } }
The above code is a simple TCP server that listens to the local 8080 port, and after receiving the client connection, reads the message sent by the client and replies" Message received". Next is a code example of the TCP client:
package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Error connecting:", err.Error()) return } defer conn.Close() message := "Hello, server" _, err = conn.Write([]byte(message)) if err != nil { fmt.Println("Error writing:", err.Error()) return } buffer := make([]byte, 1024) _, err = conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) return } fmt.Println("Server reply:", string(buffer)) }
The above is a simple TCP client, which connects to the local TCP server on port 8080 and sends the "Hello, server" message, and then receives the server's reply and Output to the console. Through this simple example, we can see that writing network applications in Go language is very simple and intuitive.
However, despite the many advantages of the Go language in network programming, there are also some challenges. One of them is that concurrency needs to be handled with caution. Improper concurrency control may lead to problems such as race conditions and resource competition. In addition, the debugging and performance optimization of the Go language may be slightly insufficient compared to other languages, and more practice and experience are required to handle it proficiently.
In summary, this article explores the practical applications and challenges of Go language in network programming, and demonstrates its advantages and simplicity through specific code examples. However, issues such as concurrency and performance still need to be carefully handled when developing network applications to ensure the stability and efficiency of the application. I hope this article can provide some reference and help for developers in Go language network programming.
The above is the detailed content of Practical applications and challenges of Go language in network programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
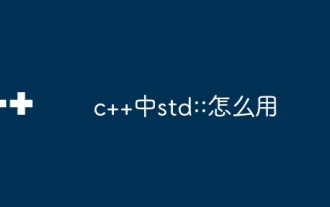
std is the namespace in C++ that contains components of the standard library. In order to use std, use the "using namespace std;" statement. Using symbols directly from the std namespace can simplify your code, but is recommended only when needed to avoid namespace pollution.
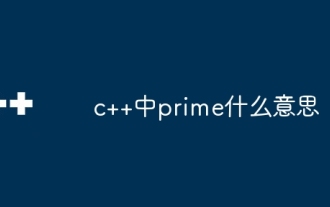
prime is a keyword in C++, indicating the prime number type, which can only be divided by 1 and itself. It is used as a Boolean type to indicate whether the given value is a prime number. If it is a prime number, it is true, otherwise it is false.

The fabs() function is a mathematical function in C++ that calculates the absolute value of a floating point number, removes the negative sign and returns a positive value. It accepts a floating point parameter and returns an absolute value of type double. For example, fabs(-5.5) returns 5.5. This function works with floating point numbers, whose accuracy is affected by the underlying hardware.
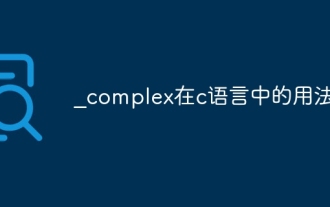
The complex type is used to represent complex numbers in C language, including real and imaginary parts. Its initialization form is complex_number = 3.14 + 2.71i, the real part can be accessed through creal(complex_number), and the imaginary part can be accessed through cimag(complex_number). This type supports common mathematical operations such as addition, subtraction, multiplication, division, and modulo. In addition, a set of functions for working with complex numbers is provided, such as cpow, csqrt, cexp, and csin.
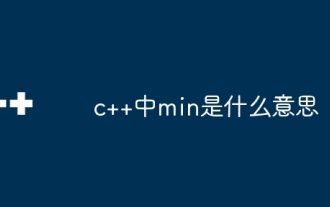
The min function in C++ returns the minimum of multiple values. The syntax is: min(a, b), where a and b are the values to be compared. You can also specify a comparison function to support types that do not support the < operator. C++20 introduced the std::clamp function, which handles the minimum of three or more values.
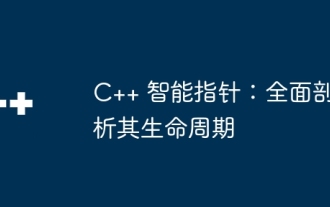
Life cycle of C++ smart pointers: Creation: Smart pointers are created when memory is allocated. Ownership transfer: Transfer ownership through a move operation. Release: Memory is released when a smart pointer goes out of scope or is explicitly released. Object destruction: When the pointed object is destroyed, the smart pointer becomes an invalid pointer.
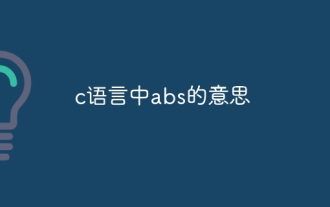
The abs() function in c language is used to calculate the absolute value of an integer or floating point number, i.e. its distance from zero, which is always a non-negative number. It takes a number argument and returns the absolute value of that number.
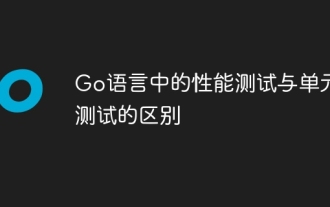
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
