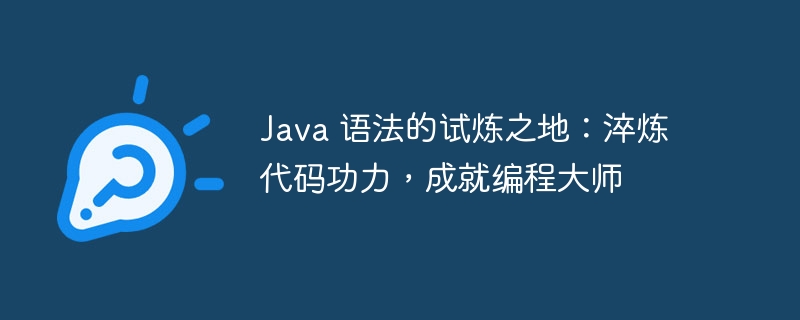
The place where Java grammar is tested: temper your coding skills and become a programming master
Java is a widely used programming language. For beginners, mastering its syntax and techniques is the first step into the programming world. For experienced developers, continuously deepening their understanding and application of Java syntax is the only way to become a true programming master. In this place where Java grammar is tested, PHP editor Banana will reveal to you some secrets for improving your coding skills, helping you better grasp the essence of Java and achieve programming skills.
- Master the declaration, initialization and conversion of different data types in Java.
- Understand variable scope, life cycle and memory management.
- Be able to skillfully use basic data types (such as int, double, String), and reference data types (such as objects, arrays).
2. Operators and expressions
- Familiar with arithmetic, logical and comparison operators in Java.
- Understand the precedence and associativity of arithmetic, logical and assignment expressions.
- Can write complex expressions and perform conditional branching and loop control.
3. Control flow
- Master the conditional statements (if-else), loop statements (while, do-while, for-each) and jump statements (break, continue) in Java.
- Understand control flow structures, including nesting and branching.
- Be able to design and implement complex control algorithms.
4. Methods and functions
- Create and call methods, and understand their parameters, return values and scope.
- Use overloading and rewriting to enhance code maintainability and flexibility.
- Be familiar with variable parameters, default parameters and lambda expressions.
5. Classes and Objects
- UnderstandObject-orientedBasic concepts of programming (OOP), including classes, objects, encapsulation and inheritance.
- Create and instantiate a class and use its methods and fields.
- Use inheritance and polymorphism to create more scalable and maintainable code.
6. Interfaces and abstract classes
- Create and use interfaces and abstract classes, and understand their role in OOP.
- Distinguish between interfaces and abstract classes, and understand their differences in defining and implementing behavior.
- Use interfaces and abstract classes to promote code decoupling and modularization.
7. Arrays and Collections
- Master the basic operations of arrays, including declaration, initialization and accessing elements.
- UnderstandCollectionFramework, including the main interfaces and implementations of List, Set and Map.
- Can use collections for data storage, retrieval and operation.
8. Input and output
- Understand file input and output operations in Java.
- Use the Scanner class to read data from the console and the PrintWriter class to write to a file.
- Be familiar with basic file handling concepts such as opening, closing, and reading/writing files.
9. Exception handling
- Understand the exception mechanism in Java, including try-catch-finally blocks.
- Catch and handle exceptions to ensure the robustness and maintainability of the code.
- Distinguish between different types of exceptions and handle them appropriately.
10. Threads and concurrency
- Understand the multithreading concept in Java, including thread creation, scheduling and communication.
- Use synchronization mechanisms, such as locks and wait notifications, to ensure the correct execution of concurrent code.
- Familiar with thread pool and executor framework to effectively manage concurrent tasks.
The above is the detailed content of The testing ground of Java syntax: refine your coding skills and become a programming master. For more information, please follow other related articles on the PHP Chinese website!