


How to correctly use the C++sort function to implement customized sorting function
The sort function uses a custom comparison function to implement customized sorting: write a comparison function: specify the sorting rules, define parameter types and return values. Call the sort function: pass the custom comparison function as the third parameter to sort the elements in the container. Example: Sort integers in descending order and strings according to custom rules (empty string first, length first, lexicographic order).
How to use the sort function in C to implement customized sorting function
sort
function is an important function in the C standard library, used to sort The elements in the container are sorted. It receives a comparison function by reference, allowing the user to sort elements based on custom criteria.
The syntax of comparison function
The syntax of comparison function is as follows:
bool compare(const T1& a, const T2& b);
Among them:
- ##T1
and
T2are the element types to be compared.
Return - true
means
ais less than
b.
Return - false
means
ais greater than or equal to
b.
Implementing a custom sort
To implement a custom sort using thesort function, you need to write a custom comparison function that specifies the sorting behavior . Here is an example:
#include <algorithm> #include <vector> using namespace std; bool compareIntsDescending(int a, int b) { return a > b; } int main() { vector<int> numbers = {1, 5, 2, 4, 3}; sort(numbers.begin(), numbers.end(), compareIntsDescending); for (auto& num : numbers) { cout << num << " "; } cout << endl; return 0; }
Output of this program:
5 4 3 2 1
compareIntsDescending comparison function converts integers from large to small Sort.
Practical case: Sorting strings by custom rules
Suppose you have an array of strings and you want to sort them according to the following rules:
- Empty strings are sorted first. Longer strings are sorted first (or in alphabetical order if the length is the same).
bool compareStrings(string a, string b) { // 检查是否为空字符串 if (a.empty() && !b.empty()) { return true; } else if (!a.empty() && b.empty()) { return false; } // 空字符串相等 if (a.empty() && b.empty()) { return false; } // 比较长度 if (a.length() < b.length()) { return true; } else if (a.length() > b.length()) { return false; } // 长度相同时按字母顺序比较 return (a < b); }
#include <algorithm> #include <vector> using namespace std; int main() { vector<string> strings = {"apple", "banana", "cherry", "dog", "cat", ""}; sort(strings.begin(), strings.end(), compareStrings); for (auto& str : strings) { cout << str << " "; } cout << endl; return 0; }
Output of this program:
dog cat apple banana cherry
The above is the detailed content of How to correctly use the C++sort function to implement customized sorting function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


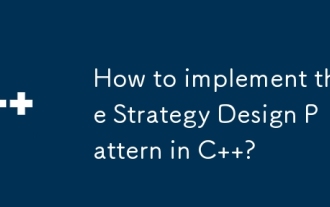
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
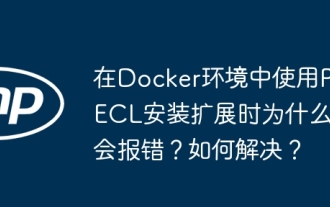
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
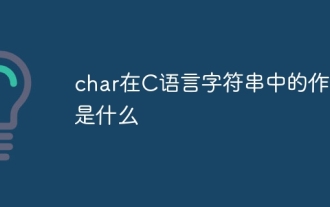
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
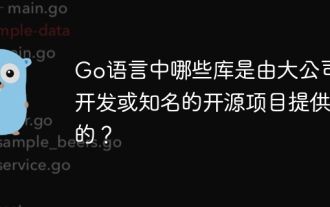
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
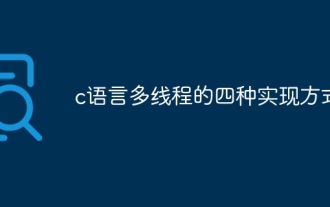
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
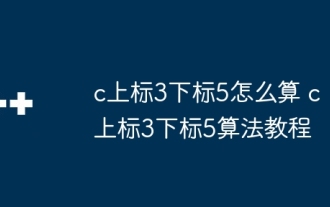
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
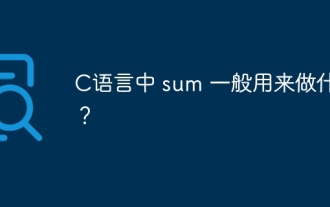
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
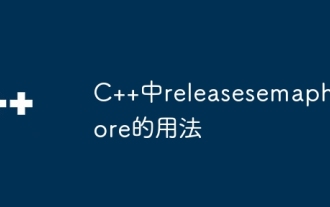
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
