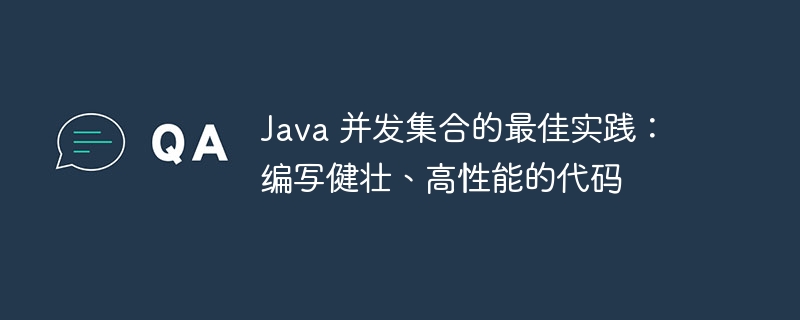
Java is a very popular programming language with a wide range of applications. At the same time, Java's concurrent programming has also received widespread attention, because concurrency is an indispensable part of Java development. PHP editor Xigua will introduce you to some best practices and techniques for writing robust and high-performance Java concurrent programming. This article will discuss threads, synchronization, locks, atomic variables, thread pools and other topics in Java concurrent programming, and provide some tips and techniques for writing efficient Java concurrent programming. Whether you are a Java beginner or an experienced developer, this article can bring you inspiration and help.
-
ConcurrentHashMap: Used for key-value pair storage in high concurrency scenarios.
-
CopyOnWriteArrayList: Used for list operations with more reading and less writing.
-
BlockingQueue: Used for communication and task queue management between threads.
Synchronous access
-
synchronized blocks: Use the synchronized keyword to synchronize code blocks.
-
Lock object: Create a dedicated lock object and use the synchronized(lock) synchronization method.
-
Concurrent atomic classes: Use atomic classes such as AtomicInteger and AtomicBoolean to perform atomic update operations.
Concurrent modification control
-
Copy-on-write strategy: Create a copy of the collection during a write operation to avoid concurrent modification exceptions.
-
Optimistic Concurrency Control (OCC): Detect and handle concurrent modifications using version numbers or timestamps.
Capacity and expansion
-
Set initial capacity: Set a reasonable initial capacity for the collection to minimize expansion operations.
-
Control the expansion factor: Adjust the expansion factor of the collection to optimize performance and memory usage.
-
Use lock-free expansion algorithm: Use a collection of lock-free expansion algorithms such as ConcurrentHashMap to improve concurrency performance.
Thread safety
-
Thread-safe collections: Use thread-safe collections provided by Java, such as ConcurrentHashMap.
- Custom thread-safe collection: For custom collections, use synchronization mechanism or lock-free algorithm to ensure thread safety.
- Defensive Copy: Create a copy before passing the collection to other threads to prevent concurrent modifications.
Deadlock Prevention
- Avoid deadlock loops: Avoid threads waiting for each other to lock, such as using a common lock sequence.
- Use timeouts: Set a timeout for lock operations to automatically release locks when a deadlock occurs.
Performance optimization
- Read-write ratio: Optimize the read-write ratio of the collection to maximize performance.
- Local variables: Store collections in local variables when possible to reduce lock contention.
- Use concurrency tools: Leverage Java concurrency tools , such as the Fork/Join framework, to improve parallelism.
Exception handling
- Handling concurrency exceptions: Expect and handle concurrency-related exceptions, such as ConcurrentModificationException.
- Use custom exceptions: Define custom exceptions to provide meaningful error information for easy debugging.
Monitoring and Diagnosis
- Use concurrency tool library: Use Java Monitor Virtual Machine (JMX) or other concurrency tool library to monitor concurrency performance.
- Logging: Log concurrent events such as deadlocks and exceptions for troubleshooting purposes.
- Unit Tests: Write unit tests to verify concurrency behavior and detect potential problems.
The above is the detailed content of Best Practices for Java Concurrent Collections: Writing Robust, High-Performance Code. For more information, please follow other related articles on the PHP Chinese website!