


Golang is like the wings of programming: exploring its role in promoting modern software development
Go Language: The Wings of Programming, Promoting Modern Software Development
Go language, an open source programming language developed by Google, is known for its efficiency, strong concurrency and code Known for portability. It has quickly become one of the preferred languages in modern software development for the following reasons:
Efficiency:
The Go language provides a compiler that can compile source code directly to the machine code, thereby improving execution speed and performance.
Concurrency:
Go language supports goroutine, which is a lightweight thread that can run at the same time to achieve high concurrency.
Code portability:
The Go language uses a static type system and provides cross-platform support, allowing code to run on a variety of operating systems and architectures.
Practical case: distributed web service
Using the Go language, we can build high-performance distributed web services. The following is a simple example that demonstrates how to create a simple HTTP server using the Go language:
package main import ( "fmt" "log" "net/http" ) func main() { // 创建一个 HTTP 路由器 mux := http.NewServeMux() // 添加一个处理程序来处理根路由(/) mux.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Hello, World!") }) // 侦听端口 8080 上的传入连接 log.Fatal(http.ListenAndServe(":8080", mux)) }
In this example:
- We first create a
HTTP
Router to handle incoming requests. - Then we added a handler to handle requests sent to the root route (
"/"
). - Finally, we start the server and listen for connections on port 8080.
Once the server is started, you can make requests locally using a browser or cURL
to test the service.
Conclusion
The Go language is becoming an indispensable tool in modern software development due to its efficiency, concurrency and code portability. It provides the key features needed to build high-performance, scalable, and maintainable applications. This article demonstrates the practical application of Go language through a simple distributed web service example to help you understand its powerful capabilities.
The above is the detailed content of Golang is like the wings of programming: exploring its role in promoting modern software development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
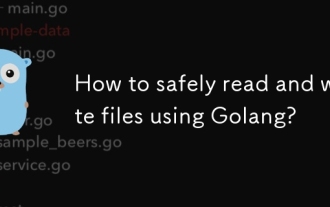
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
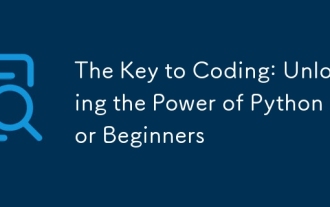
Python is an ideal programming introduction language for beginners through its ease of learning and powerful features. Its basics include: Variables: used to store data (numbers, strings, lists, etc.). Data type: Defines the type of data in the variable (integer, floating point, etc.). Operators: used for mathematical operations and comparisons. Control flow: Control the flow of code execution (conditional statements, loops).
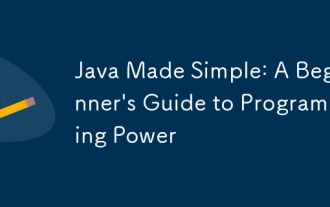
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
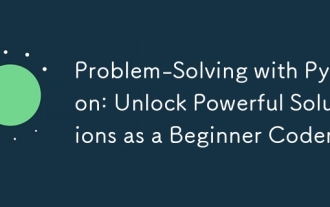
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
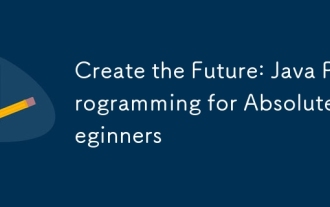
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
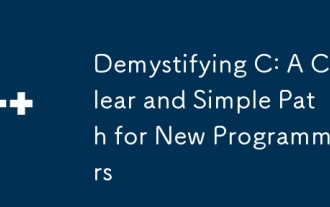
C is an ideal choice for beginners to learn system programming. It contains the following components: header files, functions and main functions. A simple C program that can print "HelloWorld" needs a header file containing the standard input/output function declaration and uses the printf function in the main function to print. C programs can be compiled and run by using the GCC compiler. After you master the basics, you can move on to topics such as data types, functions, arrays, and file handling to become a proficient C programmer.
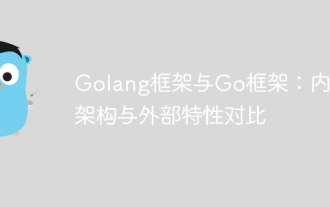
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
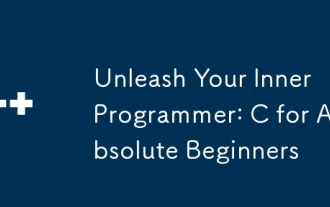
C is an ideal language for beginners to learn programming, and its advantages include efficiency, versatility, and portability. Learning C language requires: Installing a C compiler (such as MinGW or Cygwin) Understanding variables, data types, conditional statements and loop statements Writing the first program containing the main function and printf() function Practicing through practical cases (such as calculating averages) C language knowledge
