In-depth understanding of Go language design principles
A deep understanding of the design principles of the Go language is critical to taking full advantage of its powerful features, including: Concurrency and goroutines: The Go language implements concurrency through goroutines, allowing blocks of code to be executed in parallel. Channel: Channel implements secure data exchange and synchronization between goroutines. Interface: An interface defines a set of methods to promote code modularity and reusability. Garbage collection: The Go language's garbage collector automatically releases memory that is no longer used to prevent memory leaks.
In-depth understanding of Go language design principles
Go language, originally developed by Google, is famous for its simple syntax and efficient compilation Widely praised for its performance and superior concurrency. In order to take full advantage of its powerful capabilities, it is crucial to have a deep understanding of the design principles behind it.
Concurrency and goroutines
The Go language adopts a concurrent programming paradigm called "concurrency". It does this by using lightweight threads called goroutines. Goroutines run on separate stacks, allowing blocks of code to be executed in parallel. This makes the Go language ideal for handling large numbers of concurrent requests or complex calculations.
package main import ( "fmt" "time" ) func main() { go func() { for i := 0; i < 10; i++ { fmt.Println("Routine 1:", i) } }() go func() { // 另一个 goroutine for i := 0; i < 10; i++ { fmt.Println("Routine 2:", i) } }() time.Sleep(1 * time.Second) // 等待 goroutine 完成 }
Channels
Channels are another key concept in concurrent programming. They allow goroutines to safely exchange data between goroutines and enable synchronization between different goroutines.
package main import ( "fmt" "time" ) func main() { ch := make(chan int) // 创建一个通道 go func() { ch <- 10 // 发送数据到通道 }() v := <-ch // 从通道接收数据 fmt.Println(v) time.Sleep(1 * time.Second) // 等待 goroutine 完成 }
Interface
Interface allows defining a set of methods without specifying implementation details. This promotes code modularity and reusability.
package main import "fmt" type Animal interface { // 定义接口 Speak() } type Dog struct{} func (d Dog) Speak() { // 实现接口方法 fmt.Println("Woof!") } func main() { var a Animal = Dog{} // 接口变量指向结构实例 a.Speak() }
Garbage Collection
The Go language uses a memory management technology called garbage collection. The garbage collector automatically releases memory that is no longer in use, thus preventing memory leaks and упрощает development.
Practical Case
The following is an example of using Go language design principles to build a web server:
package main import ( "fmt" "net/http" "time" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { mux := http.NewServeMux() mux.HandleFunc("/", handler) srv := &http.Server{ Addr: ":8080", Handler: mux, ReadTimeout: 10 * time.Second, WriteTimeout: 10 * time.Second, } srv.ListenAndServe() }
The above is the detailed content of In-depth understanding of Go language design principles. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
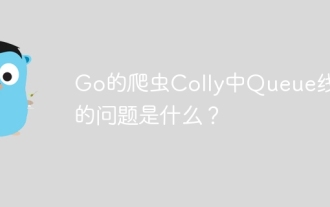
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
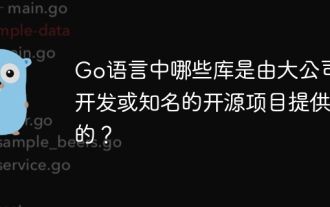
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
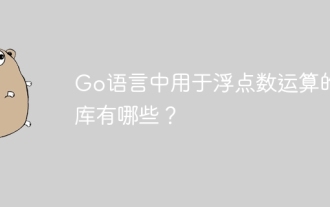
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
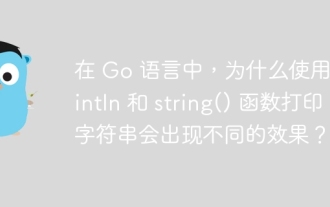
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
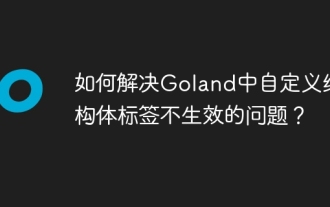
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
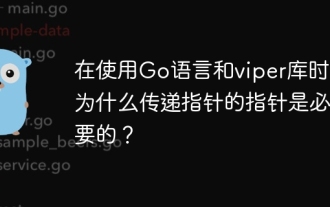
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
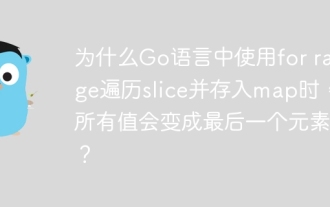
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
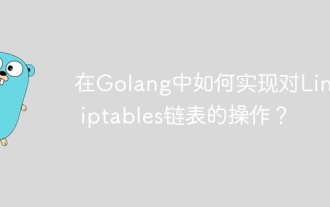
Using Golang to implement Linux...
