


Detailed explanation of Go language data types: overview of basic data types
Go language provides the following basic data types: bool: boolean int: signed integer uint: unsigned integer float: floating point number complex: complex byte: character string: string
Detailed explanation of Go language data types: Overview of basic data types
Go language is a statically strongly typed programming language, which provides a variety of basic data types to represent different data values. These data types limit the type and range of values that can be stored in variables and constants.
Basic data types
Go language provides the following basic data types:
- Boolean (bool): Represents a true or false value, the size is 1 byte.
- Integer: Includes int, int8, int16, int32 and int64, representing signed integers of different sizes.
- Unsigned integer: Includes uint, uint8, uint16, uint32 and uint64, representing unsigned integers of different sizes.
- Floating point type: Includes float32 and float64, representing floating point numbers with different precisions and ranges.
- Complex type (complex): represents a complex number with real and imaginary parts, with a size of 16 bytes.
- Character type (byte): represents ASCII characters, the size is 1 byte.
- String (string): represents a string of characters, which is essentially an immutable byte array.
Type inference
Go language supports type inference, which means that the compiler can automatically infer the type of a variable or constant without explicit declaration. For example:
var age int = 25 var name string = "John Doe" var isValid bool = true
Practical case
The following is a simple program example that demonstrates how to use basic data types:
package main import "fmt" func main() { age := 25 name := "John Doe" isValid := true fmt.Println("Age:", age) fmt.Println("Name:", name) fmt.Println("Is Valid:", isValid) }
Output:
Age: 25 Name: John Doe Is Valid: true
The above is the detailed content of Detailed explanation of Go language data types: overview of basic data types. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


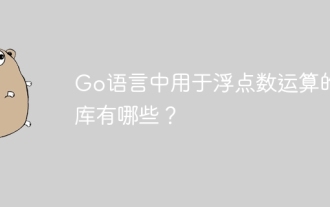
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
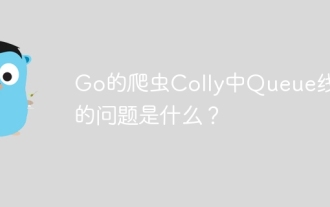
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
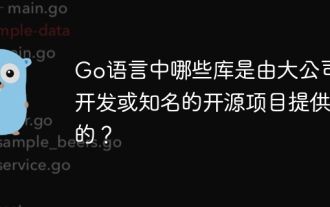
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
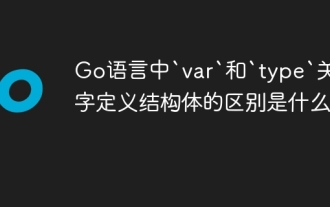
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
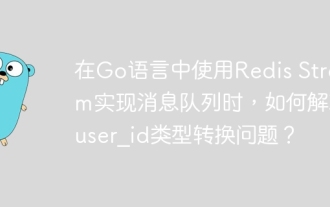
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
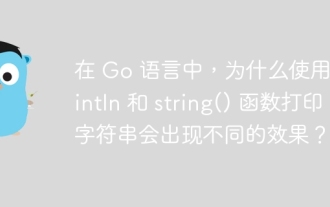
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
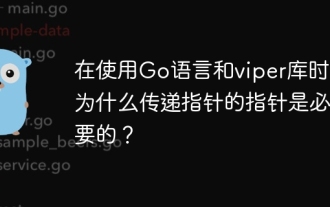
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
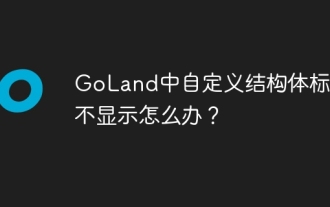
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
