


Golang structure strong transfer: detailed explanation of implementation principles and techniques
Golang 中结构体强转是将一种结构体类型的值转换为另一种类型。可以通过断言强转、反射强转、指针间接强转等技巧实现。断言强转使用类型断言,反射强转使用反射机制,指针间接强转避免值复制。具体步骤为:1. 断言强转:使用 type assertion 语法;2. 反射强转:使用 reflect.Type.AssignableTo 和 reflect.Value.Convert 函数;3. 指针间接强转:使用指针解引用。
Golang 结构体强转:实现原理与技巧详解
前言
在 Go 语言中,强转是将一种类型的值转换为另一种类型的值。结构体强转是指将一种结构体类型的值转换为另一种结构体类型的值。本文将深入探讨 Golang 中结构体强转的实现原理和各种技巧,并通过实战案例加深理解。
实现原理
在底层,Golang 中的结构体类型是一种聚合类型,它包含了多个成员变量。强转是一种内存重新解释的过程,它将一种类型的内存布局重新解释为另一种类型。
对于结构体强转,编译器会根据目标结构体的类型信息,对原结构体的内存进行重新分配和解释。具体来说:
- 原结构体的每个成员变量都以其原始类型对应的内存大小和对齐要求在目标结构体中分配空间。
- 编译器将原结构体的每个成员变量复制到目标结构体中对应的位置。
- 如果原结构体和目标结构体有相同的成员变量(名称和类型均相同),则该成员变量只需要一次内存分配和复制。
技巧
-
使用断言强转: 断言强转使用
type assertion
语法,它可以同时执行类型检查和强转操作。如果断言失败(目标结构体的类型不正确),将触发运行时恐慌。
myStruct := MyStruct{Name: "foo"} myOtherStruct, ok := myStruct.(MyOtherStruct)
- 使用反射强转: 反射是一种允许程序在运行时检查类型和值的技术。它可以通过
reflect.Type.AssignableTo
和reflect.Value.Convert
函数实现强转。
type1 := reflect.TypeOf(myStruct) type2 := reflect.TypeOf(MyOtherStruct{}) if type1.AssignableTo(type2) { myOtherStruct := reflect.ValueOf(myStruct).Convert(type2).Interface().(MyOtherStruct) }
- 使用指针(间接强转): 对于指向结构体的指针,可以使用指针解引用来实现强转。该方法避免了值复制,提高了性能。
myPtr := &MyStruct{Name: "foo"} myOtherPtr := (*MyOtherStruct)(myPtr) // 间接强转,myPtr指向myOtherStruct
实战案例
以下是一个使用强转技巧进行结构体转换的实战案例:
package main import ( "fmt" "reflect" ) type MyStruct struct { Name string Age int } type MyOtherStruct struct { Name string Age int City string } func main() { // 使用断言强转 myStruct := MyStruct{Name: "John", Age: 30} myOtherStruct, ok := myStruct.(MyOtherStruct) if ok { fmt.Println(myOtherStruct) // 打印 {John 30} } // 使用反射强转 type1 := reflect.TypeOf(myStruct) type2 := reflect.TypeOf(MyOtherStruct{}) if type1.AssignableTo(type2) { myOtherStruct := reflect.ValueOf(myStruct).Convert(type2).Interface().(MyOtherStruct) fmt.Println(myOtherStruct) // 打印 {John 30} } // 使用指针间接强转 myStructPtr := &MyStruct{Name: "Jane", Age: 25} myOtherStructPtr := (*MyOtherStruct)(myStructPtr) // 间接强转 fmt.Println(myOtherStructPtr) // 打印 {Jane 25 } }
The above is the detailed content of Golang structure strong transfer: detailed explanation of implementation principles and techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


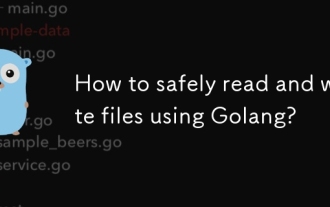
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
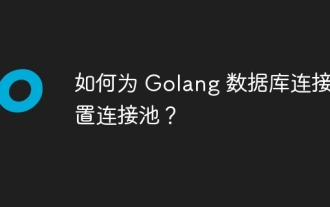
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
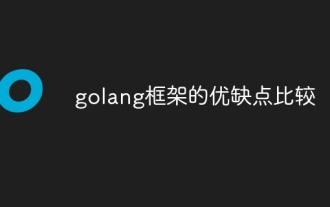
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
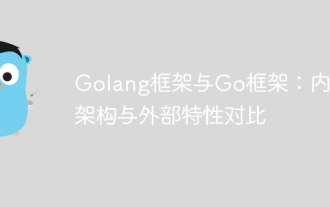
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
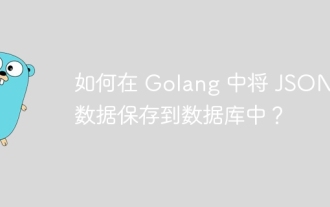
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
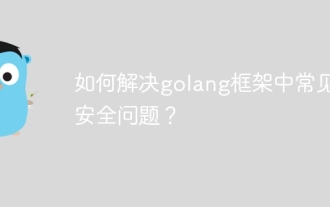
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
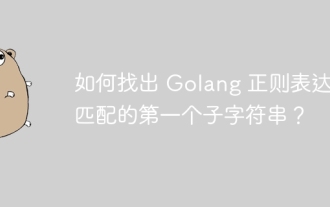
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
