Comparative analysis of C language and C++: Do you understand it?
The advantages of C compared to the C language are: more flexible syntax, support for object-oriented programming, automatic memory management, advanced functions such as function overloading and templates, and improved code readability and maintainability.
Comparative analysis of C language and C: in-depth understanding
Preface
C language and C are both widely used programming languages, but with significant differences in functionality and features. This article will provide an in-depth comparison of these two languages to help you understand their advantages, disadvantages, and applicable scenarios.
Grammar
The syntax of C language is relatively simple, while the syntax of C is more complex and flexible. C introduced object-oriented programming (OOP) concepts, adding features such as classes, inheritance, and polymorphism.
Data types
C provides a rich set of built-in data types, including Boolean values, integers, floating point types and character types. Additionally, it supports user-defined data types such as classes and structures.
Memory Management
Memory in C language is manually managed by programmers, which is prone to memory leaks or errors. Instead, C provides automatic memory management, and the compiler is responsible for allocating and freeing memory.
Object-oriented programming
Object-oriented programming (OOP) is one of the core features of C. It encapsulates data and operations into objects, enhancing the modularity and reusability of the program.
Function Overloading
Function overloading allows the creation of multiple functions in C with the same name but different parameter lists. This improves code readability and maintainability.
Templates
Templates are powerful tools in C that allow the creation of generic code that can be applied to different types of data. This eliminates the need to duplicate code.
Practical Case
Suppose you want to develop a program to manage student information. The following code shows how to perform basic tasks in C and C:
C Language
#include <stdio.h> struct Student { int id; char name[50]; float gpa; }; int main() { struct Student s; s.id = 12345; strcpy(s.name, "John Doe"); s.gpa = 3.5; printf("Student ID: %d\n", s.id); printf("Student Name: %s\n", s.name); printf("Student GPA: %.2f\n", s.gpa); }
C Class
#include <iostream> class Student { public: int id; std::string name; float gpa; // 构造函数 Student(int id, std::string name, float gpa) : id(id), name(name), gpa(gpa) {} // 获取器和设置器 int getId() { return id; } void setId(int id) { this->id = id; } std::string getName() { return name; } void setName(std::string name) { this->name = name; } float getGpa() { return gpa; } void setGpa(float gpa) { this->gpa = gpa; } }; int main() { Student s(12345, "John Doe", 3.5); std::cout << "Student ID: " << s.getId() << std::endl; std::cout << "Student Name: " << s.getName() << std::endl; std::cout << "Student GPA: " << s.getGpa() << std::endl; }
The above is the detailed content of Comparative analysis of C language and C++: Do you understand it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


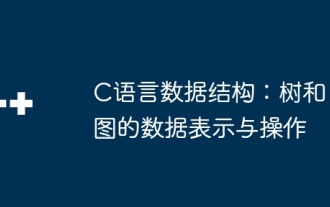
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
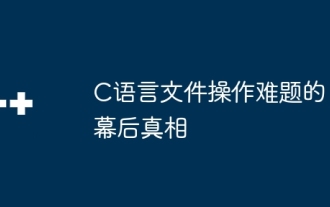
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
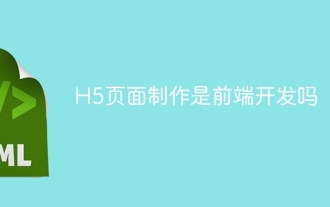
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
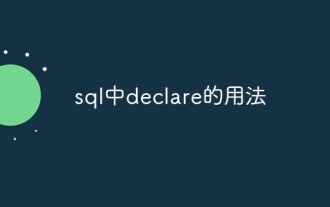
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates
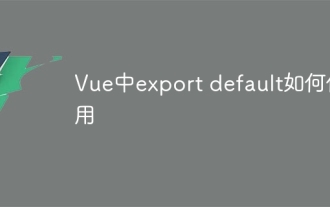
Export default in Vue reveals: Default export, import the entire module at one time, without specifying a name. Components are converted into modules at compile time, and available modules are packaged through the build tool. It can be combined with named exports and export other content, such as constants or functions. Frequently asked questions include circular dependencies, path errors, and build errors, requiring careful examination of the code and import statements. Best practices include code segmentation, readability, and component reuse.
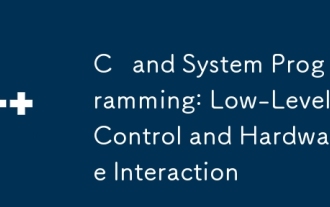
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
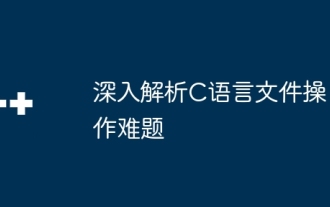
In-depth analysis of C language file operation problems Preface file operation is an important function in C language programming. However, it can also be a challenging area, especially when dealing with complex file structures. This article will deeply analyze common problems in C language file operation and provide practical cases to clarify solutions. When opening and closing a file, there are two main modes: r (read-only) and w (write-only). To open a file, you can use the fopen() function: FILE*fp=fopen("file.txt","r"); After opening the file, it must be closed after use to free the resource: fclose(fp); Reading and writing data can make
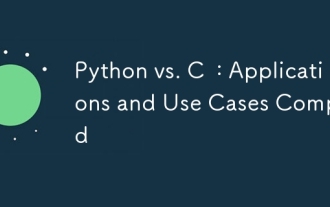
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
