C++ and C++: What's the difference?
The difference between C vs. C: Type system: C is weaker, C is stronger and requires explicit conversion. Objects and classes: Not supported by C, supported by C, allowing the creation and use of custom types. Inheritance and polymorphism: Not supported by C, supported by C, allowing class inheritance and reuse features. Function and operator overloading: Not supported by C, supported by C, allowing custom function and operator behavior.
C vs. C: Nuance Analysis
Foreword
C Yes An object-oriented programming language derived from the C language. Despite the similarities, there are important differences between the two languages. This article explores these differences and provides code examples to deepen understanding.
Type system
- C: Use a weaker type system where variables can be implicitly converted to other types.
- C: Has a more robust type system that requires explicit type conversions, thus improving code safety and readability.
// C int a = 10; double b = a; // 隐式转换 // C++ int a = 10; double b = static_cast<double>(a); // 显式转换
Objects and Classes
- C: Object-oriented programming concepts (classes, objects) are not supported.
- C: Supports object-oriented programming, allowing the creation of user-defined types (classes) and instances (objects) from them.
// C++ class Person { public: string name; }; Person john; // 创建一个 Person 对象 cout << john.name; // 访问对象的成员
Inheritance and polymorphism
- C: Inheritance and polymorphism are not supported.
- C: Supports inheritance and polymorphism, allowing derived classes to inherit the properties and methods of the base class.
// C++ class Employee : public Person { public: int salary; }; Employee mary; // 创建一个 Employee 对象 cout << mary.name << ", " << mary.salary; // 访问对象属性和方法
Function overloading and operator overloading
- C: Function overloading or operator overloading is not supported.
- C: Supports function overloading (functions with the same name but different parameters) and operator overloading (defining operators for custom types).
// C++ int add(int a, int b); // 函数重载 double operator+(double a, double b); // 运算符重载
Practical case
Consider a program that calculates the average:
C
#include <stdio.h> int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); float avg = (num1 + num2) / 2.0; printf("Average: %.2f\n", avg); }
C
#include <iostream> using namespace std; int main() { int num1, num2; cout << "Enter two numbers: "; cin >> num1 >> num2; double avg = static_cast<double>(num1 + num2) / 2; cout << "Average: " << fixed << setprecision(2) << avg << endl; }
In the C version, explicit type conversion is used to ensure that avg
is of type double
for accurate averaging value. Additionally, cout
and cin
are used for input and output, enhancing the user interface.
The above is the detailed content of C++ and C++: What's the difference?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


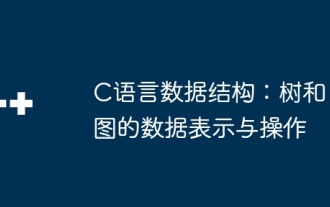
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
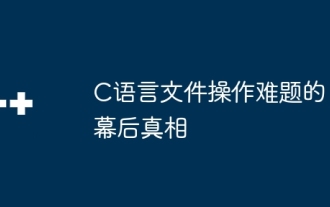
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
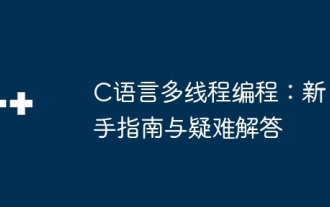
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
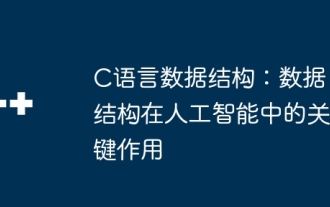
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
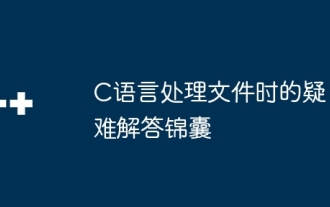
Troubleshooting Tips for C language processing files When processing files in C language, you may encounter various problems. The following are common problems and corresponding solutions: Problem 1: Cannot open the file code: FILE*fp=fopen("myfile.txt","r");if(fp==NULL){//File opening failed} Reason: File path error File does not exist without file read permission Solution: Check the file path to ensure that the file has check file permission problem 2: File reading failed code: charbuffer[100];size_tread_bytes=fread(buffer,1,siz
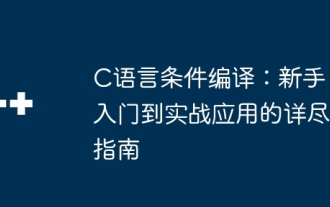
C language conditional compilation is a mechanism for selectively compiling code blocks based on compile-time conditions. The introductory methods include: using #if and #else directives to select code blocks based on conditions. Commonly used conditional expressions include STDC, _WIN32 and linux. Practical case: Print different messages according to the operating system. Use different data types according to the number of digits of the system. Different header files are supported according to the compiler. Conditional compilation enhances the portability and flexibility of the code, making it adaptable to compiler, operating system, and CPU architecture changes.
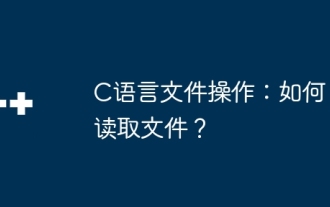
C language file operation: Read file introduction File processing is a crucial part of C language programming, which allows programs to interact with external storage devices such as disks and flash drives. This article will explore how to read files in C language. Steps to read a file to open the file: use the fopen function to open the file. This function requires two parameters: file name and open mode. Check whether the file is open: Check whether the pointer returned by the fopen function is NULL. If NULL, the file cannot be opened. Read file: Use the fread function to read data from the file to the buffer. This function requires four parameters: buffer address, buffer element size, number of elements to be read, and file pointer. Close the file: Use f
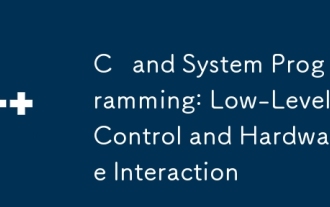
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
