Go Programming Guide: Steps to Delete Data in Files
This article provides a Go language guide for deleting data from a file. The specific steps are as follows: Create and fill the file with the data to be deleted. Open the file for reading and writing. Set the file offset to the starting position of the data to be deleted. Truncate the file from the offset. Close the file by removing data
Go Programming Guide: Steps to delete data in the file
Introduction
Deleting data in files is a common operation in Go programs. This article will provide you with a step-by-step guide on how to delete data from files using Go language.
Steps
1. Create a file
First, you need to create a file that contains the data you want to delete.
import ( "io/ioutil" "os" ) func main() { data := []byte("这是要删除的数据") err := ioutil.WriteFile("file.txt", data, 0644) if err != nil { fmt.Println("创建文件失败:", err) return } }
2. Open the file
Next, you need to open the file to be modified for writing.
file, err := os.OpenFile("file.txt", os.O_RDWR, 0644) if err != nil { fmt.Println("打开文件失败:", err) return }
3. Set the file offset
Determine the location of the data to be deleted. You can set the file offset to a specific position using the Seek
method.
offset, err := file.Seek(-len(data), io.SeekEnd) if err != nil { fmt.Println("设置偏离量失败:", err) return }
4. Truncate the file
Use the Truncate
method to truncate the file and specify the length of the data to be removed.
err = file.Truncate(offset) if err != nil { fmt.Println("截断文件失败:", err) return }
5. Close the file
Finally, use the Close
method to close the file.
file.Close()
Practical case
The following is a practical case to delete all data in the file.txt
file:
package main import ( "io/ioutil" "os" ) func main() { err := ioutil.WriteFile("file.txt", []byte("这是要删除的数据"), 0644) if err != nil { fmt.Println("创建文件失败:", err) return } file, err := os.OpenFile("file.txt", os.O_RDWR, 0644) if err != nil { fmt.Println("打开文件失败:", err) return } offset, err := file.Seek(0, io.SeekEnd) if err != nil { fmt.Println("设置偏离量失败:", err) return } err = file.Truncate(offset) if err != nil { fmt.Println("截断文件失败:", err) return } file.Close() }
The above is the detailed content of Go Programming Guide: Steps to Delete Data in Files. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


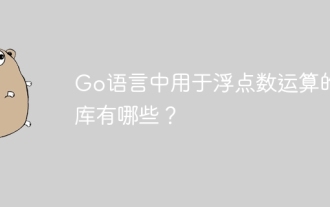
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
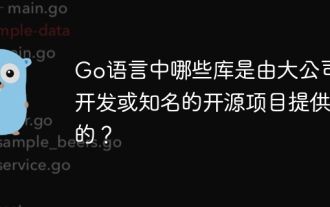
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
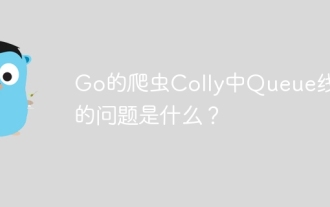
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
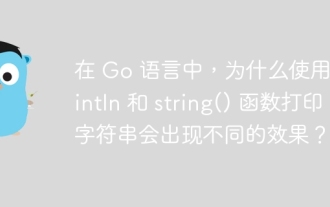
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
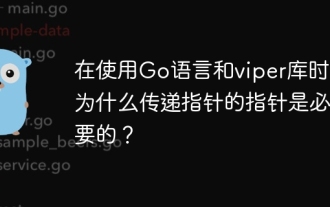
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
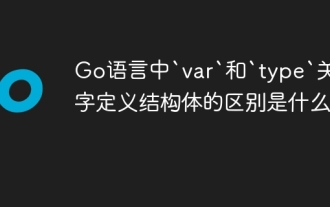
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
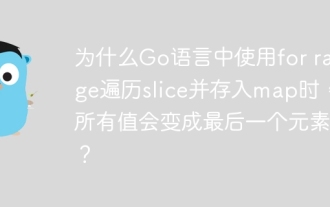
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
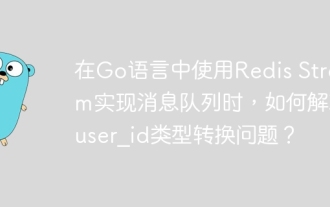
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
