How to type π in C#
Output π in C
#In C#, there are the following methods to output π:
1. Use the built-in Math.PI constant
The Math.PI constant stores the approximate value of π, which is approximately 3.141592653589793. To use it, just write this in your code:
Console.WriteLine(Math.PI);
2. Use Math.Atan2(1, 0)
Math.Atan2(y, x) The function returns the angle (in radians) formed by y and x. When y is 1 and x is 0, we get π/2. By multiplying the result by 2, we can get π:
Console.WriteLine(2 * Math.Atan2(1, 0));
3. Using a Loop Approximation
We can use a loop to approximate the value of π. The following code uses Machen's formula to calculate an approximation of π:
double pi = 0; for (int i = 0; i < 100000; i++) { pi += Math.Pow(-1, i) / (2 * i + 1); } Console.WriteLine(pi * 4);
4. Using the System.Numerics namespace
.NET 5 and above includes System. The Numerics namespace provides a BigInteger type specifically designed to represent numbers of arbitrary precision:
using System.Numerics; BigInteger piApprox = BigInteger.Atan2(BigInteger.One, BigInteger.Zero) * 2; Console.WriteLine(piApprox.ToString());
Which method you choose depends on the required precision and performance requirements. For most cases, using Math.PI constants is sufficient.
The above is the detailed content of How to type π in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


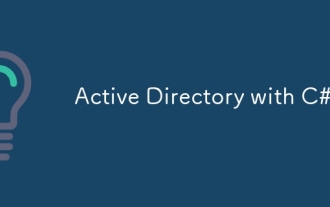
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
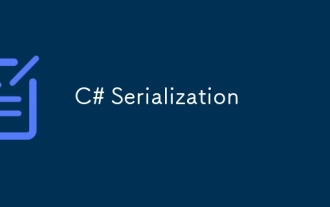
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
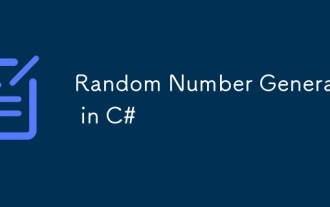
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
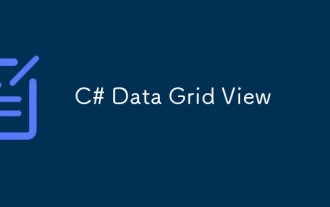
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
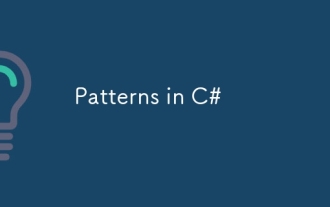
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
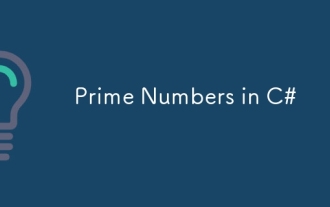
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
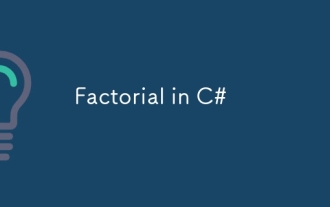
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
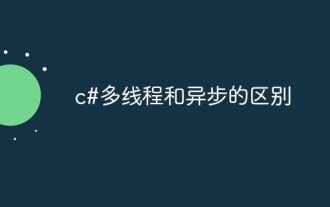
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
