How to use vue framework
How to use the Vue framework: Install Vue.js dependencies. Include the Vue.js script in the HTML file. Create a Vue instance and mount it on a DOM element. Build reusable components. Leverage data responsiveness for automatic UI updates. Use event listeners to respond to user interactions. Handle page navigation via Vue Router. Manage global state via Vuex.
How to use the Vue framework
Vue.js is a progressive JavaScript framework for building user interface. Its main features include data responsiveness, componentization and scalability.
Getting Started
To start using Vue.js, just follow these steps:
- Install Vue.js dependencies:
npm install vue
- Include the Vue.js script in your HTML file:
<script src="vue.js"></script>
- Create a Vue instance and mount it on the DOM element:
new Vue({ el: '#app' })
Build component
Vue.js encourages the use of reusable components to build user interfaces. A component can be treated as an independent UI element, with its own template, style and logic:
<code>// MyComponent.vue <template> <div>Hello, {{ name }}!</div> </template> <script> export default { props: ['name'] }; </script></code>
Data responsiveness
One of the core features of Vue.js One is data responsiveness. This means that when the data in the Vue instance changes, the UI automatically updates. This is achieved by using a state management library like Vuex or directly modifying the data of the Vue instance.
Event Handling
Vue.js allows you to respond to user interactions using event listeners. Events can be triggered and listened to through the v-on
directive or the $emit
method:
<code><button @click="handleClick">Click me</button> <script> export default { methods: { handleClick() { // 触发一个自定义事件 this.$emit('button-clicked'); } } }; </script></code>
Routing and state management
For more complex applications, Vue.js provides routing and state management solutions. Vue Router is used to handle page navigation, while Vuex is used to manage global state:
- Vue Router: https://router.vuejs.org/
- Vuex: https:// vuex.vuejs.org/
Other Features
Vue.js also provides a range of other features, including:
- Template syntax: Used to define the UI declaratively
- Transition effects: Used to smoothly transition between elements
- Animation: For creating dynamic and interactive animations
Learning resources
- ##Vue.js official documentation: https:// vuejs.org/v2/guide/
- Vue.js Tutorial: https://www.vuemastery.com/courses/intro-to-vue-js/
- Vue.js Community Forum :https://forum.vuejs.org/
The above is the detailed content of How to use vue framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


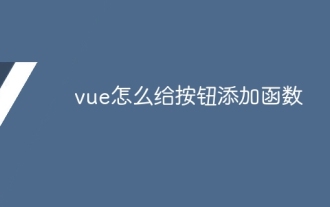
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
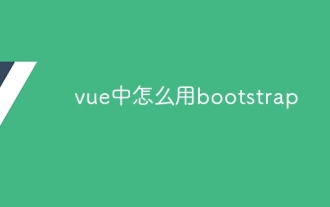
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
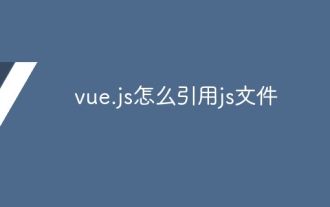
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
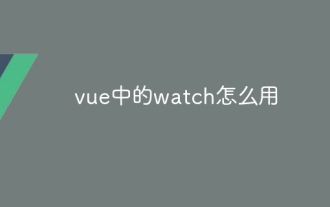
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
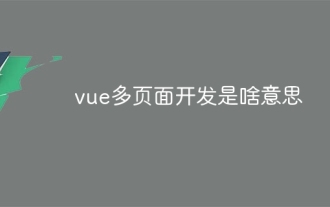
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
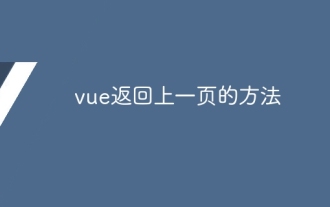
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
