Anatomy of Go reflection: understanding its principles and usage
Introduction: The reflection function of the Go language allows developers to inspect and modify the code structure at runtime, and obtain type and value metadata through the built-in interface. Principle: Based on the built-in interfaces reflect.Type (type metadata), reflect.Value (value metadata) and reflect.Kind (basic type name enumeration). Usage: Check type, modify value. Practical example: Create a custom type and use reflection to generate JSON output.
Analysis of Go reflection: understanding its principles and usage
Introduction
Reflection It is an advanced feature of programming languages that allows applications to inspect and modify the structure of their own code at runtime. The reflection support in the Go language is very powerful, allowing developers to go deep into the application and dynamically perform type checking, value modification, and code generation.
Principle
Go reflection is based on a set of built-in interfaces:
-
reflect.Type
: represents Go Type metadata. -
reflect.Value
: Metadata representing a Go value, including its type and underlying value. -
reflect.Kind
: An enumeration type that defines the names of various basic types.
By using these interfaces, you can obtain various information about Go types and values, such as type names, field names, and method signatures.
Usage
Checking the type
The following code shows how to check the http.Request
type Field:
import ( "fmt" "reflect" "net/http" ) func main() { req := http.Request{} t := reflect.TypeOf(req) for i := 0; i < t.NumField(); i++ { field := t.Field(i) fmt.Printf("%s: %s\n", field.Name, field.Type) } }
Modify value
Reflection can also modify Go values. The following code shows how to modify the URL field in http.Request
:
import ( "net/http" "reflect" ) func main() { req := http.Request{} // 获取 URL 字段的 reflect.Value field := reflect.ValueOf(&req).Elem().FieldByName("URL") // 检查 URL 字段是否可设置 if field.CanSet() { // 动态设置 URL 字段 newURL := &http.URL{Host: "example.com"} field.Set(reflect.ValueOf(newURL)) } }
Practical case
In the following practical case, we use reflection to create a custom type and use it to generate JSON output:
import ( "encoding/json" "fmt" "reflect" ) // 自定义类型 type Person struct { Name string Age int } func main() { // 反射获取 Person 类型 personType := reflect.TypeOf(Person{}) // 创建 Person 值 person := Person{"John", 30} // 创建 Person 值的 Value 对象 personValue := reflect.ValueOf(person) // 构建 JSON 输出 jsonOutput := fmt.Sprintf(`{"name": "%s", "age": %d}`, personValue.FieldByName("Name").String(), personValue.FieldByName("Age").Int(), ) // 输出 JSON fmt.Println(jsonOutput) }
The above is the detailed content of Anatomy of Go reflection: understanding its principles and usage. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
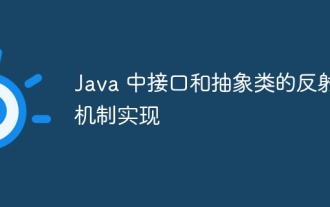
The reflection mechanism allows programs to obtain and modify class information at runtime. It can be used to implement reflection of interfaces and abstract classes: Interface reflection: obtain the interface reflection object through Class.forName() and access its metadata (name, method and field) . Reflection of abstract classes: Similar to interfaces, you can obtain the reflection object of an abstract class and access its metadata and non-abstract methods. Practical case: The reflection mechanism can be used to implement dynamic proxies, intercepting calls to interface methods at runtime by dynamically creating proxy classes.
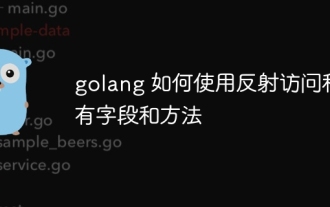
You can use reflection to access private fields and methods in Go language: To access private fields: obtain the reflection value of the value through reflect.ValueOf(), then use FieldByName() to obtain the reflection value of the field, and call the String() method to print the value of the field . Call a private method: also obtain the reflection value of the value through reflect.ValueOf(), then use MethodByName() to obtain the reflection value of the method, and finally call the Call() method to execute the method. Practical case: Modify private field values and call private methods through reflection to achieve object control and unit test coverage.
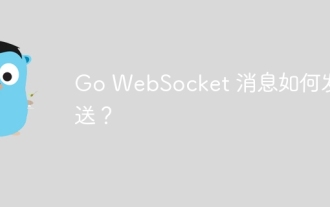
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
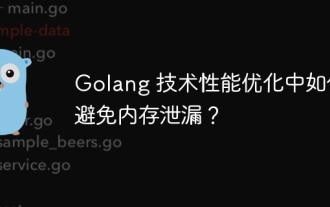
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
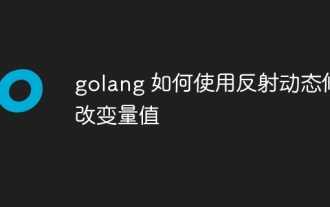
Go language reflection allows you to manipulate variable values at runtime, including modifying Boolean values, integers, floating point numbers, and strings. By getting the Value of a variable, you can call the SetBool, SetInt, SetFloat and SetString methods to modify it. For example, you can parse a JSON string into a structure and then use reflection to modify the values of the structure fields. It should be noted that the reflection operation is slow and unmodifiable fields cannot be modified. When modifying the structure field value, the related fields may not be automatically updated.
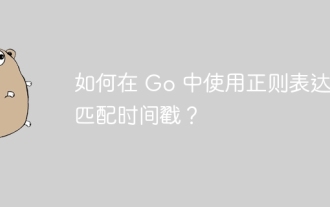
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
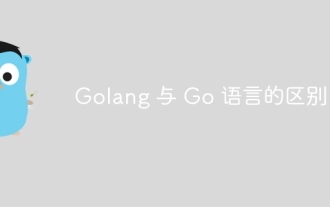
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
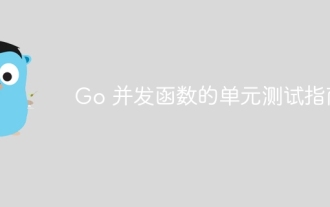
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.
