Go Exploration: An in-depth look at reflection capabilities
Reflection is a feature that inspects and modifies the structure and behavior of code at runtime, implemented in Go through the reflect package. Reflection allows: Obtaining the structure and metadata of a type Dynamically creating values Calling methods
Go Explore: Deep analysis of reflection capabilities
Reflection is a powerful form of programming Feature that allows applications to inspect and modify the structure and behavior of code at runtime. In Go language, reflection is provided through the reflect
package. This article will lead you to deeply explore the reflection function of Go and demonstrate its application through practical cases.
Basics
Each type in Go corresponds to a type object represented by reflect.Type
. Type objects provide access to type structures, fields, and methods. It also allows creating new values of the type and calling its methods.
Accessing type information
To get the type object of a type, use the reflect.TypeOf
function:
type Person struct { Name string Age int } func main() { t := reflect.TypeOf(Person{}) fmt.Println(t.Name(), t.Kind()) }
This will Print:
Person struct
Dynamicly created values
You can use the reflect.New
function to dynamically create values of type:
v := reflect.New(t).Elem() v.FieldByName("Name").SetString("John") v.FieldByName("Age").SetInt(30)
Now v
refers to a Person
value whose Name
field is "John" and the Age
field is 30.
Call Method
You can use the reflect.Value.Method
method to call a method of type:
m := v.MethodByName("String") m.Call(nil)
This will call #String
method on the ##Person type and prints "John (30)".
Practical Case
Reflection is widely used in practical applications in Go. Here are some common examples:- Dynamic generation of data formats such as JSON or YAML
- Serialization and deserialization of complex objects
- Implementing code generators
- Constructing objects based on command line arguments
Conclusion
Go’s reflection capabilities provide a powerful and flexible way to deal with the structure and behavior of your code. By understanding basic concepts and practical examples, you can use reflection to solve various problems.The above is the detailed content of Go Exploration: An in-depth look at reflection capabilities. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


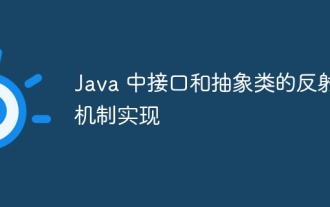
The reflection mechanism allows programs to obtain and modify class information at runtime. It can be used to implement reflection of interfaces and abstract classes: Interface reflection: obtain the interface reflection object through Class.forName() and access its metadata (name, method and field) . Reflection of abstract classes: Similar to interfaces, you can obtain the reflection object of an abstract class and access its metadata and non-abstract methods. Practical case: The reflection mechanism can be used to implement dynamic proxies, intercepting calls to interface methods at runtime by dynamically creating proxy classes.
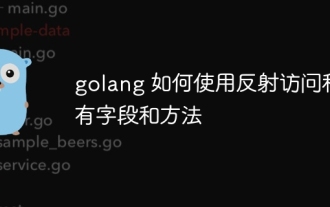
You can use reflection to access private fields and methods in Go language: To access private fields: obtain the reflection value of the value through reflect.ValueOf(), then use FieldByName() to obtain the reflection value of the field, and call the String() method to print the value of the field . Call a private method: also obtain the reflection value of the value through reflect.ValueOf(), then use MethodByName() to obtain the reflection value of the method, and finally call the Call() method to execute the method. Practical case: Modify private field values and call private methods through reflection to achieve object control and unit test coverage.
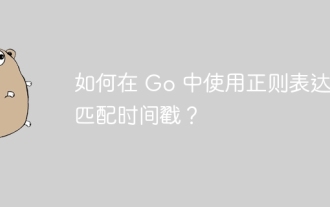
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
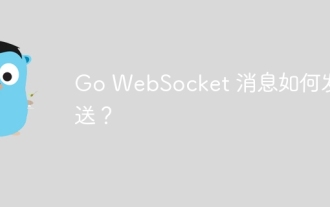
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
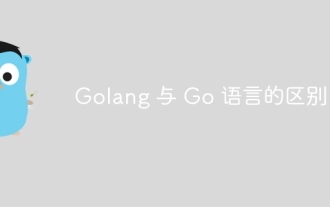
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
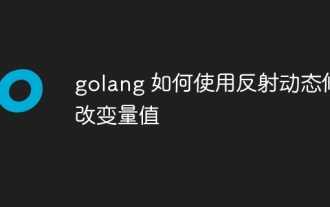
Go language reflection allows you to manipulate variable values at runtime, including modifying Boolean values, integers, floating point numbers, and strings. By getting the Value of a variable, you can call the SetBool, SetInt, SetFloat and SetString methods to modify it. For example, you can parse a JSON string into a structure and then use reflection to modify the values of the structure fields. It should be noted that the reflection operation is slow and unmodifiable fields cannot be modified. When modifying the structure field value, the related fields may not be automatically updated.
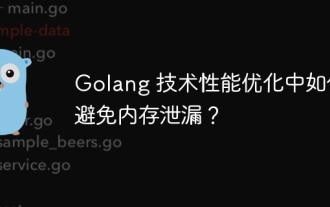
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
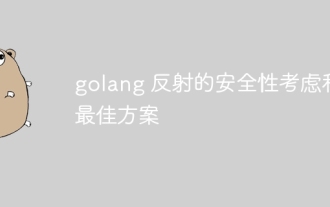
Reflection provides type checking and modification capabilities in Go, but it has security risks, including arbitrary code execution, type forgery, and data leakage. Best practices include limiting reflective permissions, operations, using whitelists or blacklists, validating input, and using security tools. In practice, reflection can be safely used to inspect type information.
