Efficiently execute POST requests in Go language
To send a POST request in Go language, you can follow the following steps: Import the necessary packages. Create an http client. Create an http request, specifying the method, URL, and request body. Set necessary request headers. Execute the request and get the response. Process the response body.
Efficient execution of POST requests in the Go language
POST requests are very useful when interacting with a web server, such as submitting a form or creating a new resource. In Go, use the net/http
package to easily send POST requests.
1. Import necessary packages
import "net/http"
2. Create http
client
Create a http
client to handle the request:
client := http.Client{}
3. Create a http
request
Use http.NewRequest
Create a new http
request, specifying the method, URL, and request body (if required):
req, err := http.NewRequest("POST", "https://example.com", body) if err != nil { // 处理错误 }
4. Set the request headers
Settings for the request Any necessary headers, such as Content-Type
:
req.Header.Set("Content-Type", "application/json")
5. Execute the request
Use client.Do
to execute the request and get the response:
resp, err := client.Do(req) if err != nil { // 处理错误 }
6. Processing the response
Use resp.Body
to read and process the response body:
defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { // 处理错误 } fmt.Println(string(body))
Practical case: Create a new user
Consider an API where we need to create a new user using a POST request:
const userURL = "https://example.com/api/v1/users" type User struct { Name string `json:"name"` } func main() { client := http.Client{} user := User{ Name: "My New User", } jsonBytes, err := json.Marshal(user) if err != nil { // 处理错误 } req, err := http.NewRequest("POST", userURL, bytes.NewReader(jsonBytes)) if err != nil { // 处理错误 } req.Header.Set("Content-Type", "application/json") resp, err := client.Do(req) if err != nil { // 处理错误 } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { // 处理错误 } fmt.Println(string(body)) }
In the above example, we first create a User
structure to represent the new user. We then serialized the user data to JSON and built a new http.Request
. Finally, we execute the request and process the response.
The above is the detailed content of Efficiently execute POST requests in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


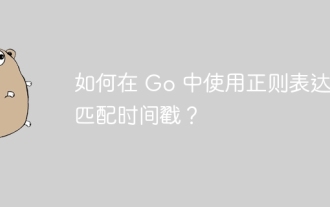
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
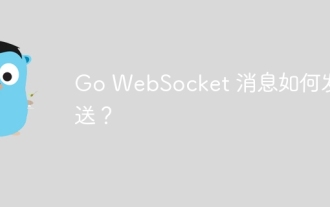
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
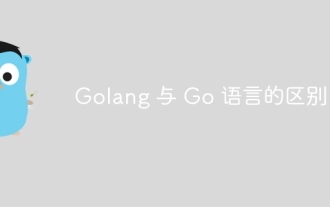
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
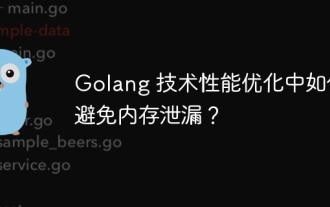
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
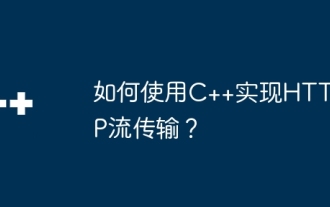
How to implement HTTP streaming in C++? Create an SSL stream socket using Boost.Asio and the asiohttps client library. Connect to the server and send an HTTP request. Receive HTTP response headers and print them. Receives the HTTP response body and prints it.
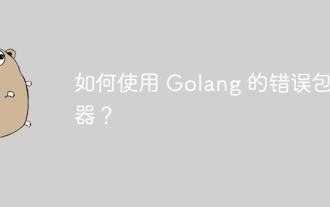
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
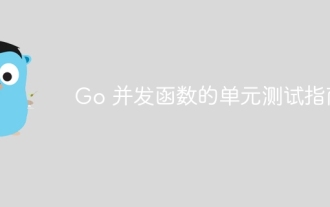
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.
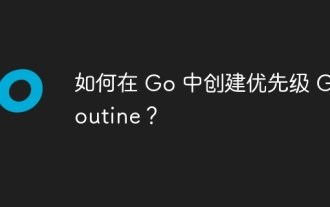
There are two steps to creating a priority Goroutine in the Go language: registering a custom Goroutine creation function (step 1) and specifying a priority value (step 2). In this way, you can create Goroutines with different priorities, optimize resource allocation and improve execution efficiency.
